Question: Does Redis Lettuce Client Connect to ElastiCache?
Answer
Yes, the Redis Lettuce client can connect to an Amazon ElastiCache cluster. The Lettuce client is a Java-based library and it supports advanced features like SSL/TLS encryption, which is required for connecting to certain ElastiCache configurations.
To connect to an ElastiCache instance using the Lettuce client, follow these steps:
- First, add the Lettuce dependency to your
pom.xml
file (if using Maven):
<dependency>
<groupId>io.lettuce.core</groupId>
<artifactId>lettuce-core</artifactId>
<version>6.1.5.RELEASE</version> <!-- Check for the latest version -->
</dependency>
- Next, in your Java code, create a Redis client with the necessary configuration options:
import io.lettuce.core.RedisClient;
import io.lettuce.core.RedisURI;
import io.lettuce.core.api.StatefulRedisConnection;
import io.lettuce.core.api.sync.RedisCommands;
public class LettuceElastiCache {
public static void main(String[] args) {
// Replace with your ElastiCache endpoint and port
String host = "<your-elasticache-endpoint>";
int port = 6379; // or your custom port
RedisURI uri = RedisURI.Builder.redis(host, port)
.withSsl(true) // Enable SSL if needed
.withVerifyPeer(false) // Disable peer verification if required
.build();
RedisClient client = RedisClient.create(uri);
StatefulRedisConnection<String, String> connection = client.connect();
RedisCommands<String, String> commands = connection.sync();
// Test the connection
commands.set("hello", "world");
String value = commands.get("hello");
System.out.println("Value: " + value);
connection.close();
client.shutdown();
}
}
Replace <your-elasticache-endpoint>
with your ElastiCache instance's endpoint and update the port if necessary.
Remember to enable SSL/TLS encryption and disable peer verification only if it's required by your ElastiCache configuration.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common ElastiCache Questions (and Answers)
- How to configure ElastiCache in AWS?
- How to view ElastiCache data?
- Is ElastiCache stateless?
- What is ElastiCache Replication Group?
- When to use ElastiCache vs DynamoDB?
- When to use ElastiCache?
- Can ElastiCache store session data?
- How to improve ElastiCache performance?
- How does AWS ElastiCache work?
- Can't connect to ElastiCache Redis
- Is ElastiCache a database?
- How to clear Elasticache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
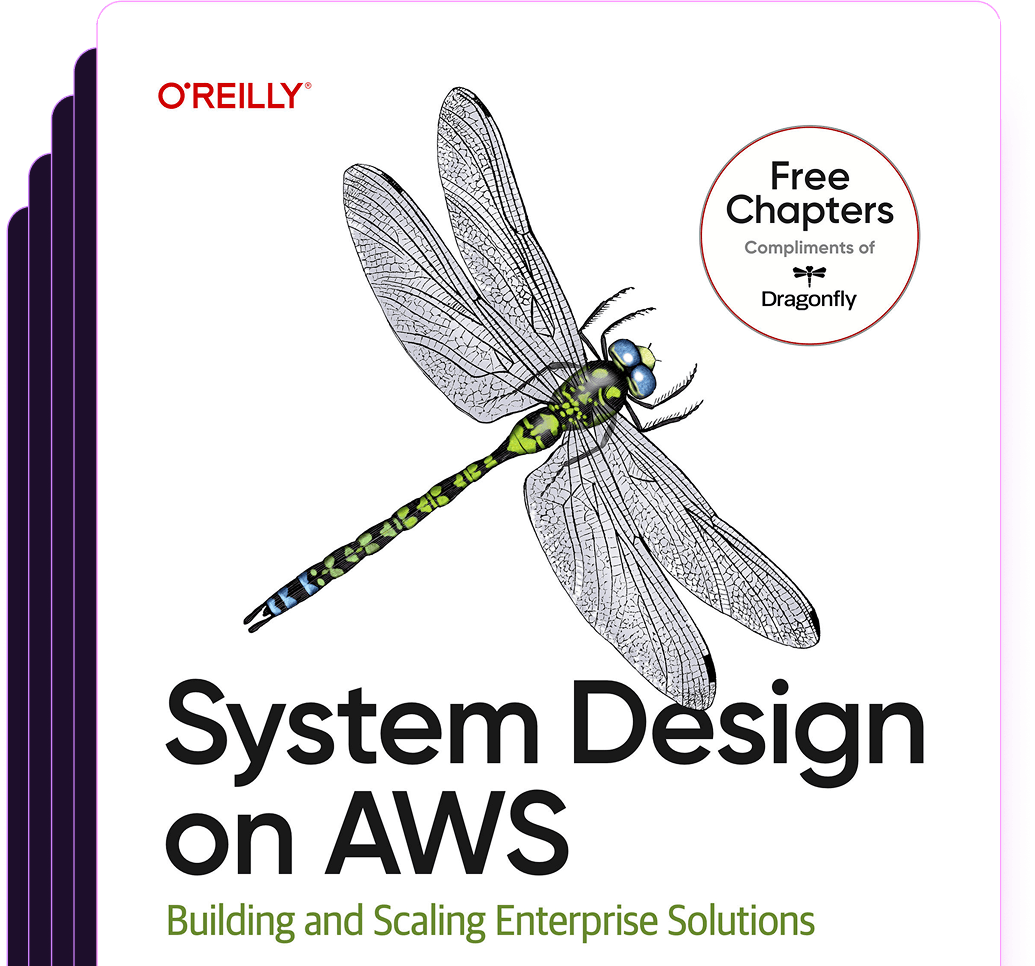
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost