Question: How to connect to Redis server?
Answer
To connect to a Redis server, you can use the redis-cli
command-line interface tool that comes with Redis.
Here are the steps to connect to a Redis server:
- Open a terminal or command-line prompt.
- Type
redis-cli
in the command line and press Enter. If Redis is running on the same machine, it will connect to the default Redis serverlocalhost
on port6379
.
redis-cli
- If your Redis server is running on a different host or port, you can specify it using the
-h
and-p
options respectively.
redis-cli -h redis.example.com -p 6380
- If you need to authenticate to access the Redis server, you can use the
-a
option followed by the password.
redis-cli -h redis.example.com -p 6380 -a mypassword
After connecting to the Redis server, you can start executing Redis commands such as SET
, GET
, HSET
, HGETALL
, and many others.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
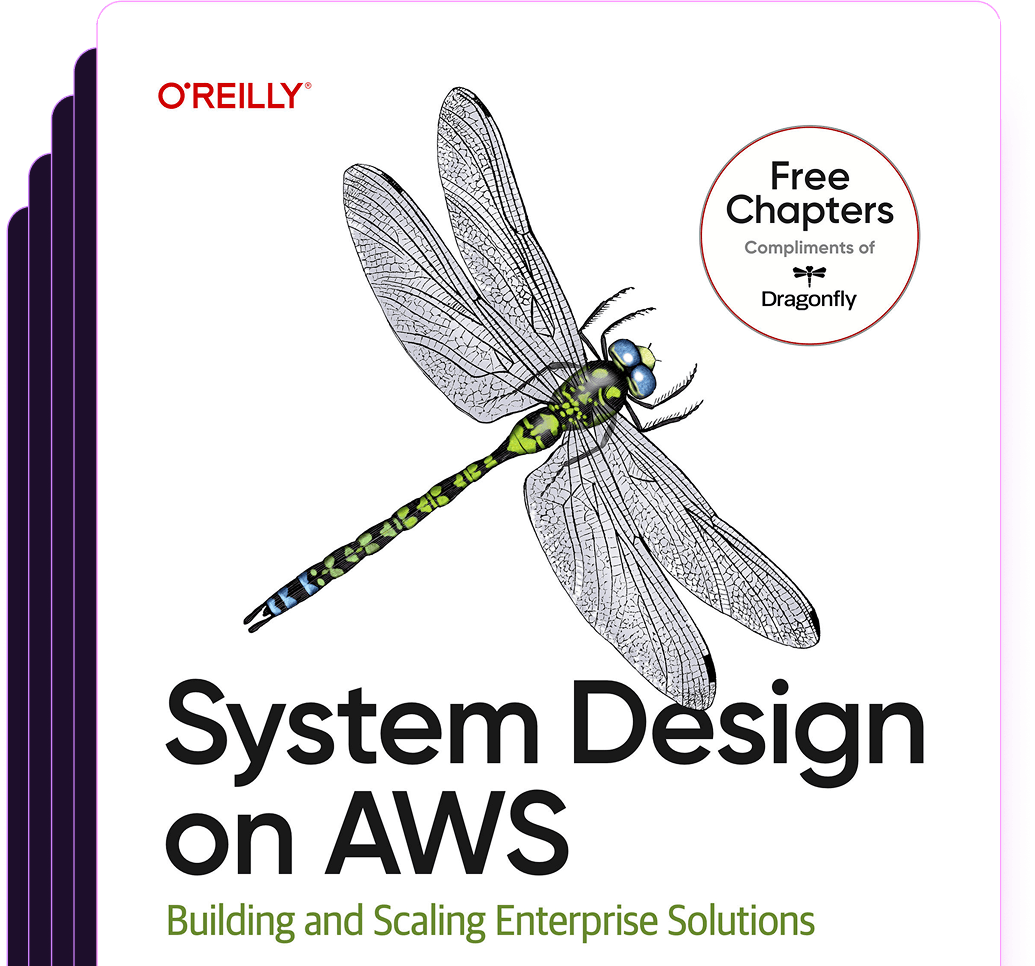
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost