Question: How can I scale write operations in Redis?
Answer
Scaling write operations in Redis can be achieved through several strategies. Let's discuss some of them.
Sharding
Sharding is the process of splitting your dataset into smaller parts and storing them across multiple instances. You can shard your data either by key range or by hash slot, depending on what better suits your use case.
Remember that client-side sharding requires careful planning to ensure even data distribution and avoid hotspots.
import rediscluster
# Here we're using a hash tag to ensure related data ends up on the same shard
startup_nodes = [{"host": "127.0.0.1", "port": "7001"}]
rc = rediscluster.RedisCluster(startup_nodes=startup_nodes, decode_responses=True)
rc.set("{user1}.name", "John")
rc.set("{user1}.email", "john@example.com")
Write-behind Caching
This strategy involves writing data to the cache and then synchronously or asynchronously updating the main database. This method helps alleviate pressure from heavy write operations on the primary database. Remember to handle potential consistency issues.
r = redis.Redis(host='localhost', port=6379, db=0)
# Writing to Redis cache first
r.set('some_key', 'some_value')
# Later, this value can be written to the main database
Pipelining
Redis pipelining allows you to send multiple commands to the server in one go, reducing the latency costs associated with multiple round trips. However, it's not suitable for all use cases as it may increase memory usage.
pipe = r.pipeline()
pipe.set('key1', 'value1')
pipe.set('key2', 'value2')
pipe.execute()
Using Redis Cluster
A Redis Cluster provides a way to run a Redis installation where data is automatically sharded across multiple nodes. It increases the write scalability by distributing loads among multiple nodes.
Keep in mind that while these strategies can help improve write scalability, they also introduce additional complexity. Always evaluate trade-offs based on your specific needs.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
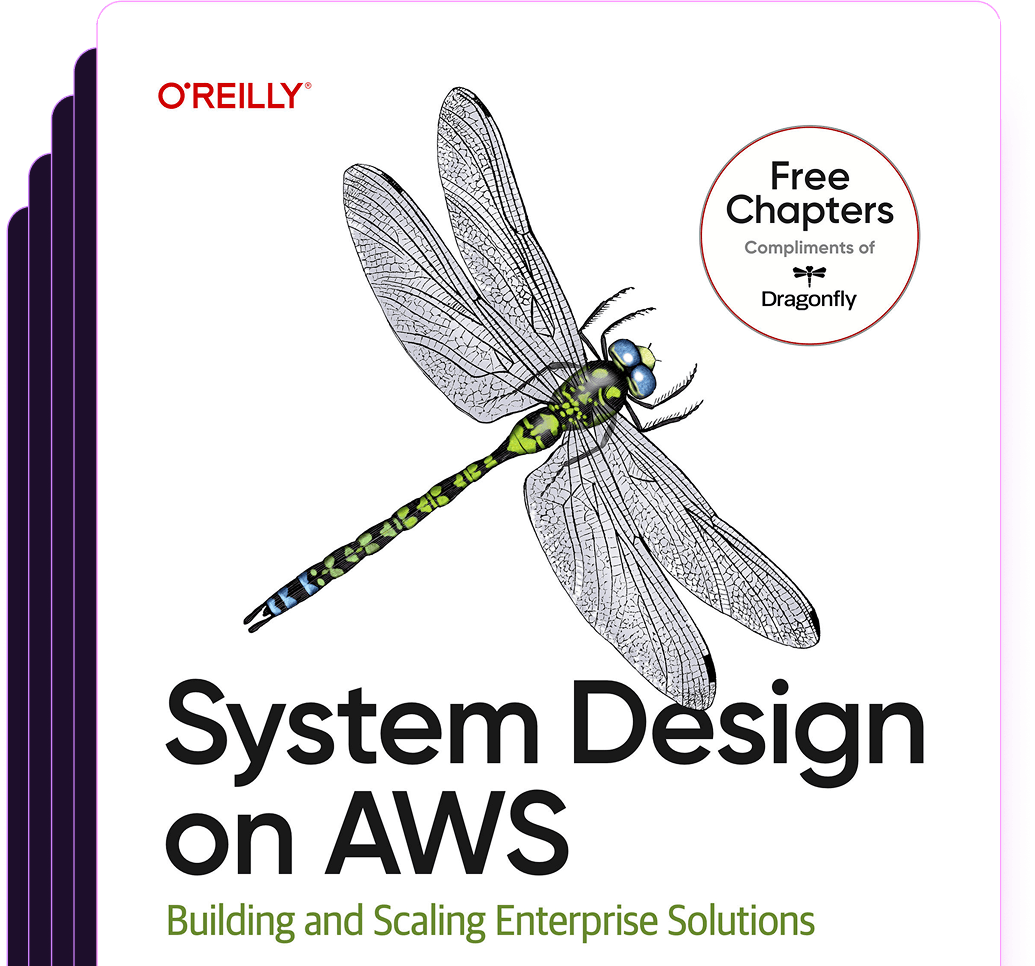
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost