Question: What are the performance considerations when using Redis ZSet?
Answer
When dealing with Redis sorted sets (ZSets), there are a few key performance considerations to keep in mind:
- Size of the ZSet: The bigger the ZSet, the more memory it will occupy. This is important to remember because Redis is an in-memory database, so all your data needs to fit into available RAM.
- Operations on ZSets: The complexity of operations on ZSets is generally dependent on the number of elements involved rather than the size of the ZSet itself. For example, the time complexity for adding an element to a ZSet (
ZADD
) or getting an element's score (ZSCORE
) is O(1). However, operations likeZRANGE
,ZREVRANGE
,ZRANGEBYSCORE
have a time complexity of O(log(N)+M) where N is the number of elements in the ZSet and M is the number of elements returned. - Redis Single Threaded Architecture: Since Redis uses a single-threaded event-driven architecture, a long-running operation can block other operations. Because operations on large ZSets can be computationally expensive, they may tie up the Redis process longer than you'd want. If such operations are common in your use case, consider breaking them down if possible, or scheduling them during off-peak hours.
Here's a simple Python code snippet showing how to interact with a ZSet using the redis-py
library:
import redis
# connect to Redis
r = redis.Redis(host='localhost', port=6379, db=0)
# add members to a ZSet
r.zadd('myzset', {'member1': 1, 'member2': 2})
# print all members of the ZSet
print(r.zrange('myzset', 0, -1))
Understanding the performance implications of using ZSets can assist you in designing more efficient applications with Redis.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
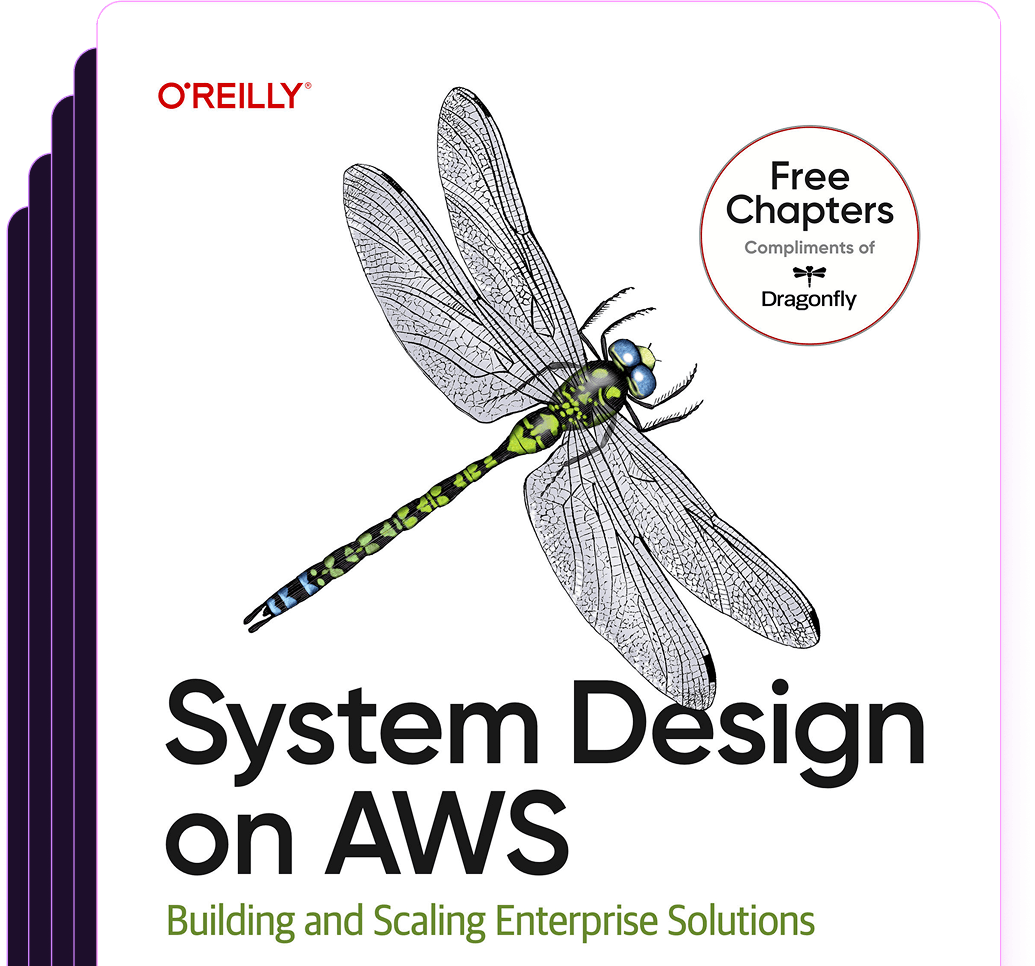
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost