Question: How to stop Redis server?
Answer
To stop the Redis server, you can use the SHUTDOWN
command which gracefully shuts down the server. There are two ways to issue this command:
- Using the redis-cli command-line tool:
```
redis-cli SHUTDOWN
``` - Sending a signal to the Redis process using the
kill
command:
```
kill <pid>
```
Replace<pid>
with the process ID of the Redis server. You can find the process ID by running the following command:
```
ps aux | grep redis-server
```
Note that sending a SIGTERM signal (default when using kill
) to Redis is equivalent to issuing a SHUTDOWN SAVE
command, which saves the data to disk before shutting down. If you want to shut down Redis without saving the data to disk, you can send a SIGINT signal instead:
```
kill -2 <pid>
```
It's generally recommended to use the SHUTDOWN
command to ensure that Redis shuts down gracefully and all data is saved properly.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
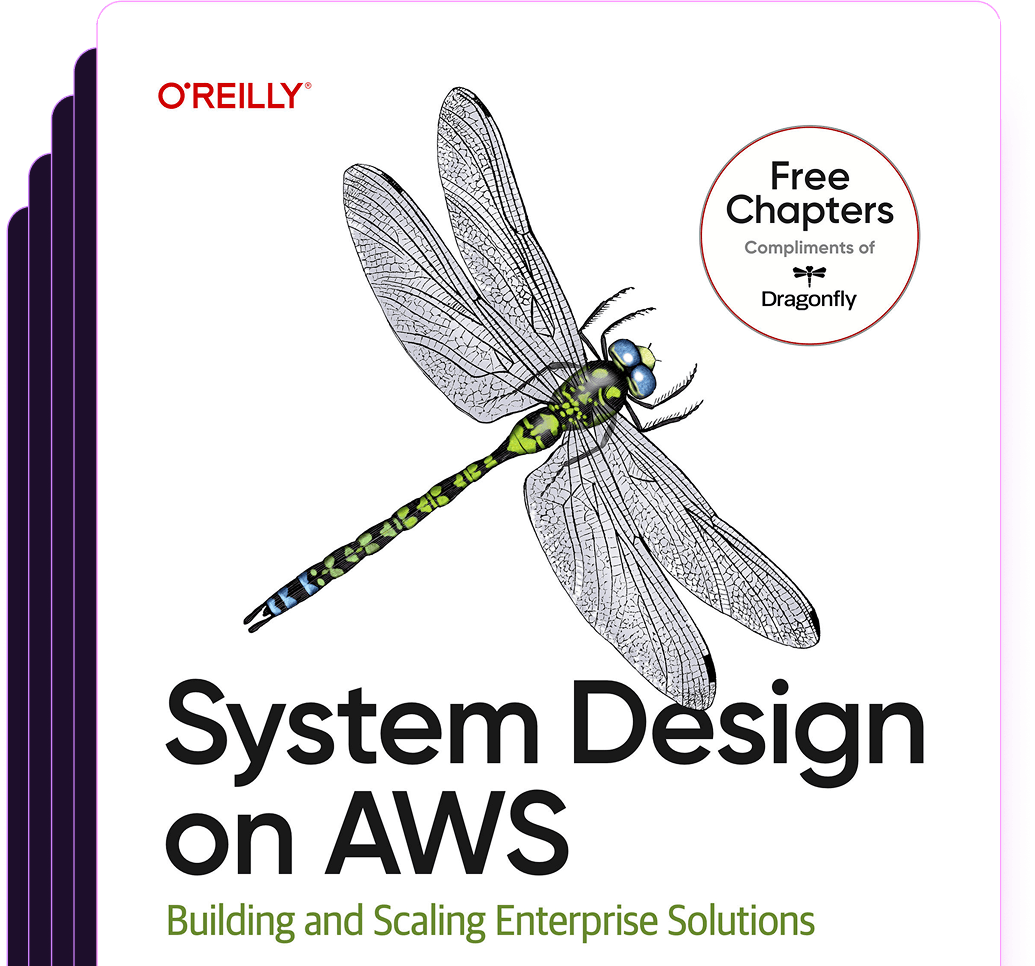
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost