Memcached Gets in C# (Detailed Guide w/ Code Examples)
Use Case(s)
The Get
and GetAsync
methods are commonly used to retrieve items from a memcached server in C#. If you have previously stored data in memcached, you can retrieve it using the key that was used during storage. This is particularly useful when caching data to improve your application's performance.
Code Examples
Here's an example of how to use the Get
method:
using Enyim.Caching;
using Enyim.Caching.Memcached;
MemcachedClient client = new MemcachedClient();
string key = "SampleKey";
string value = "Hello, Memcached!";
client.Store(StoreMode.Set, key, value);
// Retrieving the value using Get method.
string retrievedValue = client.Get<string>(key);
Console.WriteLine(retrievedValue); // Prints: Hello, Memcached!
In this code snippet, we first store a string value with a certain key, and then we retrieve it using the Get<string>
method.
If you're working with async/await, here is how you'd use GetAsync
:
var retrievedValue = await client.GetAsync<string>(key);
Console.WriteLine(retrievedValue.Value); // Prints: Hello, Memcached!
The GetAsync<string>
method returns a Task<IGetOperationResult<string>>
, which can be awaited to get the result.
Best Practices
- Only use memcached for data that can be regenerated or re-retrieved since it's not a permanent storage option.
- Always handle exceptions while interacting with memcached. The server might not always be available, and attempting to access values could result in exceptions.
- Use meaningful keys that describe the data being stored, this will help during debugging.
- Avoid storing large objects in memcached, as it could lead to increased memory usage and network traffic.
Common Mistakes
- Storing critical data: Memcached is not meant for persistent storage, so never store data in it that you can't afford to lose.
- Not using a consistent hashing mechanism: If you're using multiple servers, make sure you use a consistent hashing mechanism to ensure that keys are evenly distributed among all servers.
FAQs
1. What happens if the key doesn't exist in memcached? The Get
method will return null if the key doesn't exist.
2. How can I check if an item exists in memcached without retrieving it? You can use the TryGet
or TryGetAsync
methods which return a boolean indicating whether the item exists or not.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
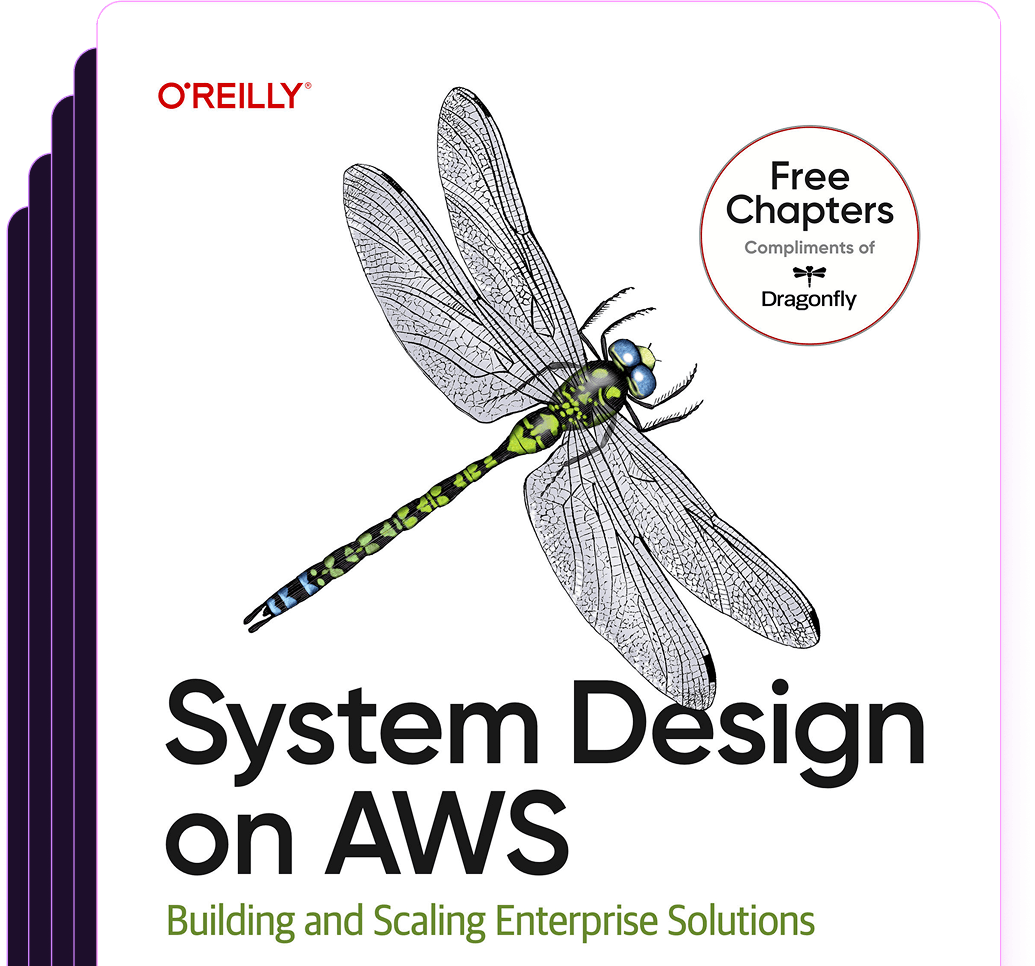
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost