Redis HMGET in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Redis HMGET
is commonly used when there's a need to retrieve multiple field values of a Hash object in a single command. This comes in handy when you have to fetch multiple related data points stored as fields in a Redis hash.
Code Examples
In Java, you often use Jedis, a popular Redis client, to interact with Redis. Here's an example:
import redis.clients.jedis.Jedis;
public class App {
public static void main(String[] args) {
//Connecting to Redis server on localhost
Jedis jedis = new Jedis("localhost");
// Set fields in the hash
jedis.hset("user:1001", "name", "John");
jedis.hset("user:1001", "email", "john@example.com");
// Get multiple fields from the hash
List<String> result = jedis.hmget("user:1001", "name", "email");
System.out.println(result);
// output: [John, john@example.com]
}
}
In this code, we first connect to the Redis server using Jedis. We then set a couple of fields in a hash identified by the key "user:1001". Using HMGET
, we can retrieve the values of the specified fields in one command.
Best Practices
- Try to structure your data such that related fields are grouped under a single hash. This optimizes the use of commands like
HMGET
. - When using
HMGET
, ensure that the fields you're trying to get actually exist - the command will return null for any non-existing fields.
Common Mistakes
- Missing error handling: Redis interactions can fail due to network issues or server problems. Always include error handling when performing Redis operations.
- Incorrect use of
HMGET
: Remember thatHMGET
is used for retrieving values from a hash, not for setting them.
FAQs
Q: What happens if the specified key does not exist?
A: If the provided key doesn't exist, Redis HMGET
will return 'nil' for every field specified in the command.
Q: Can I use HMGET
to get fields from multiple hashes at once?
A: No, you can't. HMGET
only works with one hash at a time. If you want to fetch data from multiple hashes, you'll have to use multiple commands or a transaction.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
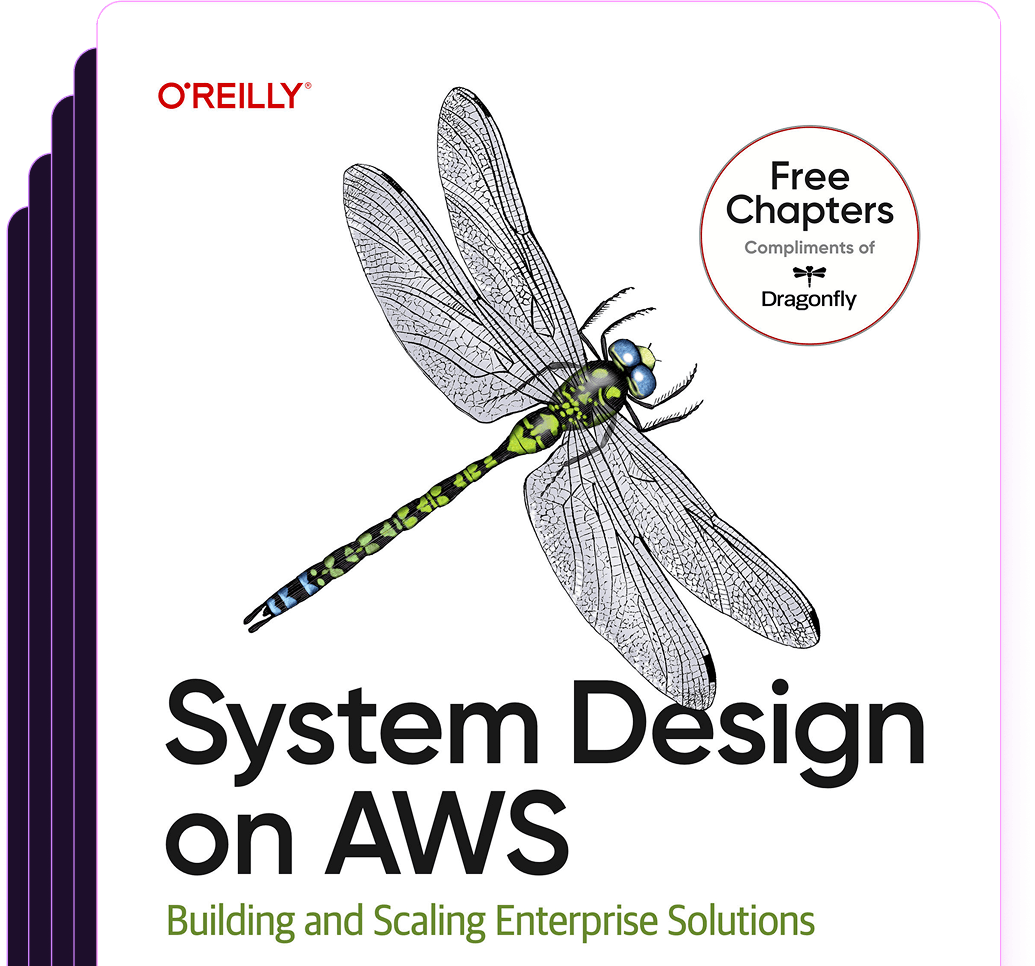
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost