Redis HGET in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
Redis HGET is a command used to retrieve the value associated with a specific field in a hash stored at a key. It's useful when you need to get specific data from a hash, such as retrieving a particular user's details from a collection of users.
Code Examples
Here is an example of how to use HGET in Node.js with the node-redis
client.
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis...');
});
// Create a hash
client.hmset('user:1001', 'name', 'John Doe', 'email', 'john.doe@example.com', function(err, reply) {
if(err) {
console.error(err);
} else {
console.log(reply); // Outputs "OK"
}
});
// Retrieve a field from the hash using HGET
client.hget('user:1001', 'name', function(err, reply) {
if(err) {
console.error(err);
} else {
console.log(reply); // Outputs "John Doe"
}
});
In this example, we first create a connection to the Redis server, then set up a hash with HMSET, and finally retrieve a specific field from the hash using HGET.
Best Practices
- Always handle potential errors in your callbacks to prevent any unhandled exceptions.
- Remember that HGET returns null when the hash or the field does not exist. So always check for null values before using the returned data.
- Close the Redis connection once you're done using it to free up system resources.
Common Mistakes
- Attempting to use HGET on non-hash data types will result in an error. Make sure the key you're accessing points to a hash.
- Forgetting to check for null when the field doesn't exist in the hash, which can lead to "null pointer" errors.
FAQs
Q: Can I use HGET to get multiple fields at once? A: No, HGET can only retrieve one field at a time. If you need to get multiple fields, consider using HGETALL or HMGET.
Q: What happens if I use HGET on a key that doesn't exist? A: Redis will return a null value, as it treats non-existing keys as empty hashes.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
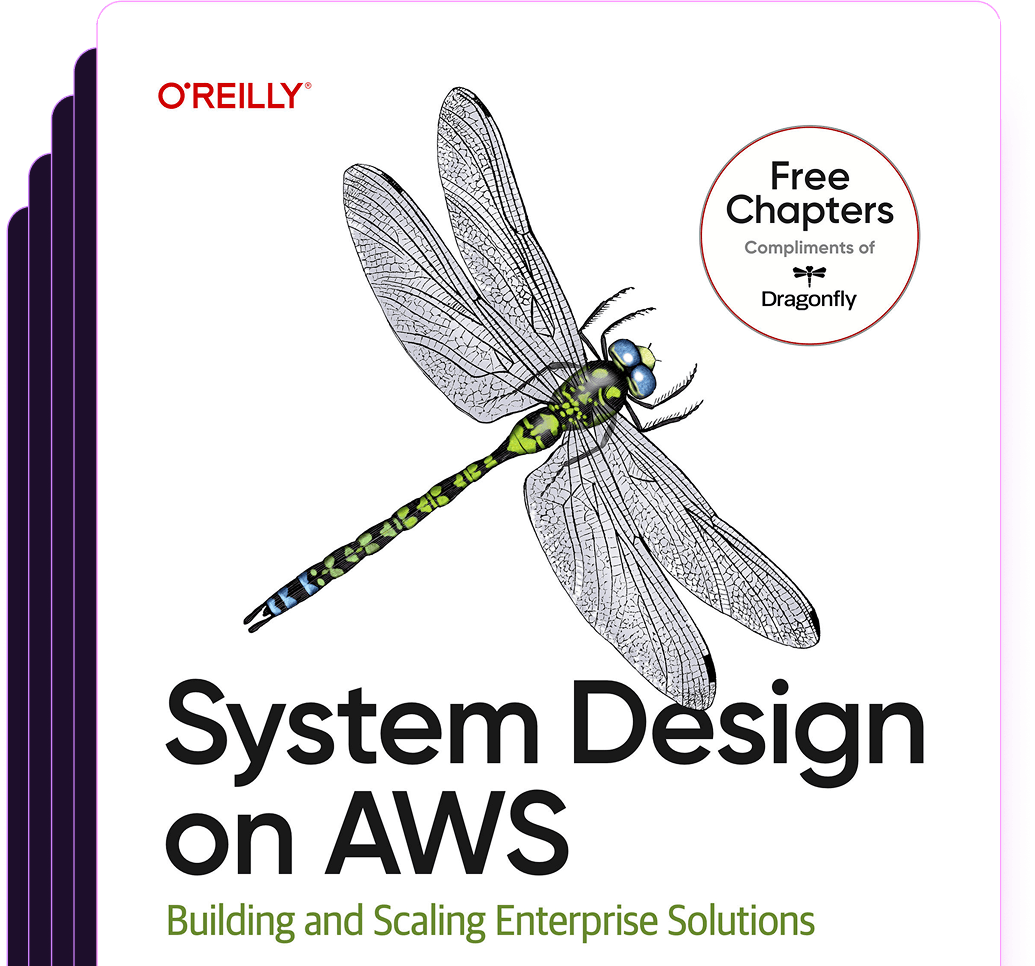
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost