Redis HMGET in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
Redis HMGET is used to retrieve the value of one or more fields stored in a hash. This is particularly useful when:
- You need to fetch multiple pieces of data from a hash in a single operation for efficiency.
- You're working with a system where data is organized in key-value pairs and grouped together under a common hash.
Code Examples
Let's assume we have a hash representing a user with fields like name, email, and age. Here's how you can use HMGET to fetch these fields.
const redis = require("redis");
const client = redis.createClient();
client.hmset("user:1001", "name", "John Doe", "email", "john@example.com", "age", "30", redis.print);
client.hmget("user:1001", "name", "email", "age", function(err, result){
if (err) {
console.error(err);
} else {
console.log(result); // Prints: [ 'John Doe', 'john@example.com', '30' ]
}
});
In this example, HMSET
is used to set the values and then HMGET
retrieves them. The result is an array that contains the values of the specified fields.
Best Practices
- Use HMGET instead of multiple GET commands to enhance efficiency when fetching multiple fields from a hash.
- Always handle errors in the callback function to avoid potential application crashes or bugs.
Common Mistakes
- HMGET returns values in the same order as they were requested. Make sure not to confuse the order, it does not necessarily match the order they were inserted.
FAQs
Q: What will happen if the requested field is not present in the hash?
A: Redis will return a special nil
value for fields that do not exist in the hash.
Q: Can I use HMGET to retrieve all fields and values from a hash?
A: No, to retrieve all fields and their respective values from a hash, use the HGETALL
command.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
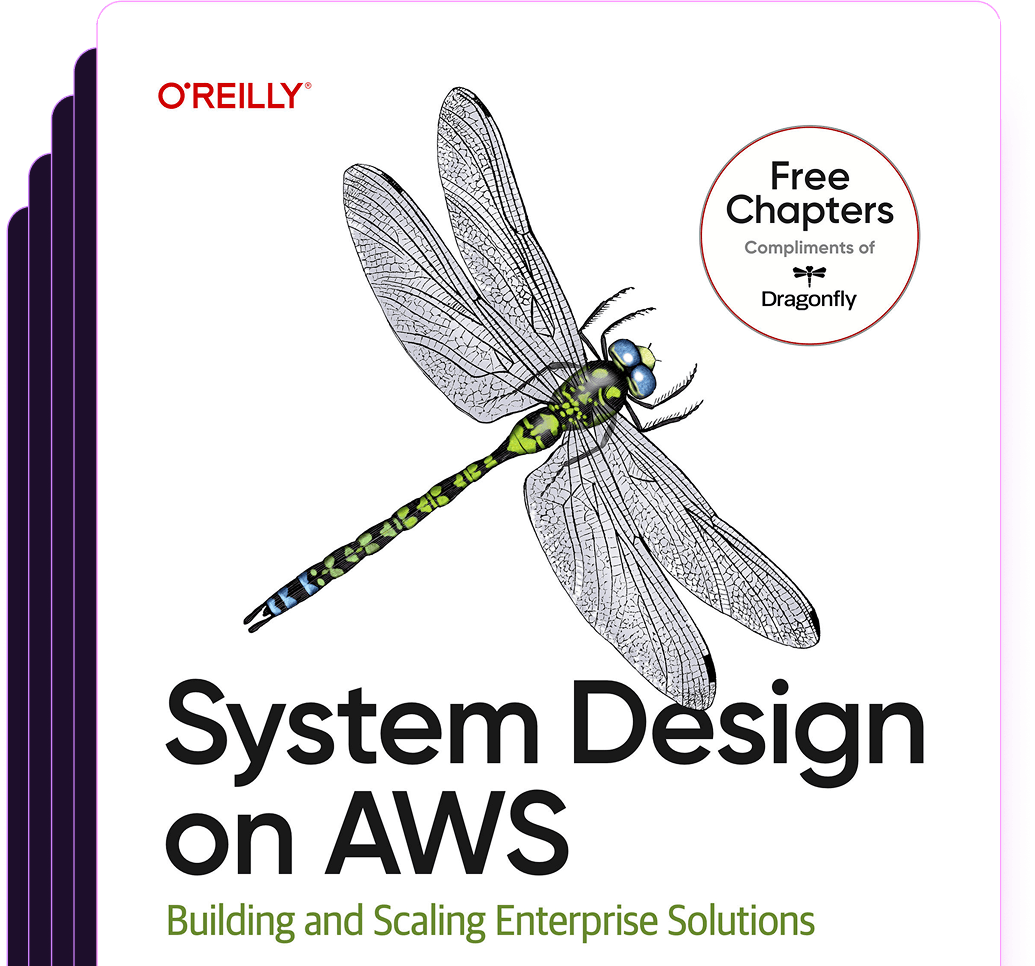
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost