Redis HDEL in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
Redis HDEL
is commonly used when there is a need to delete one or more fields from a hash stored at a particular key. This is useful in scenarios where you want to remove specific items from a hash without impacting other fields. For example, if you have a user profile stored in a hash and you want to delete specific attributes like 'email' or 'phone number', you could use HDEL
.
Code Examples
Here's an example of using HDEL
in Node.js with the node-redis
client:
const redis = require('redis');
const client = redis.createClient();
client.on('error', function(error) {
console.error(error);
});
// Setting a hash
client.hmset("user:1000", "email", "john@example.com", "phone", "555-1234", (err, res) => {
if (err) throw err;
// Deleting a field
client.hdel("user:1000", "phone", (err, res) => {
if (err) throw err;
console.log(`Deleted ${res} field.`);
});
});
In this example, we first create a Redis client and handle any errors that may occur during the connection. Then, we set a hash for the key "user:1000". Next, we delete the "phone" field from "user:1000" using HDEL
.
Best Practices
- Always check for errors in callbacks. Redis operations are asynchronous and any issues will be returned in the callback.
- Remember that
HDEL
will return the number of fields that were removed. If the specified fields do not exist,HDEL
will ignore them and return the count of fields actually deleted.
Common Mistakes
- A common mistake is trying to delete a field from a key that is not a hash. Redis will return an error in this case. Make sure that the key you're operating on is indeed a hash.
- Not handling errors correctly. Since Redis operations are asynchronous, any issues such as connection problems or operations on non-existing keys will be returned in the callback, and should be properly handled.
FAQs
Q: What happens if I try to delete a field that does not exist in the hash? A: Nothing. Redis will simply ignore non-existing fields and return the count of fields that were actually deleted.
Q: Can I delete multiple fields at once? A: Yes, you can. Simply pass the additional fields as arguments to the HDEL
command. CODE_BLOCK_PLACEHOLDER_1
In this example, both "phone" and "email" fields would be deleted from the "user:1000" hash.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
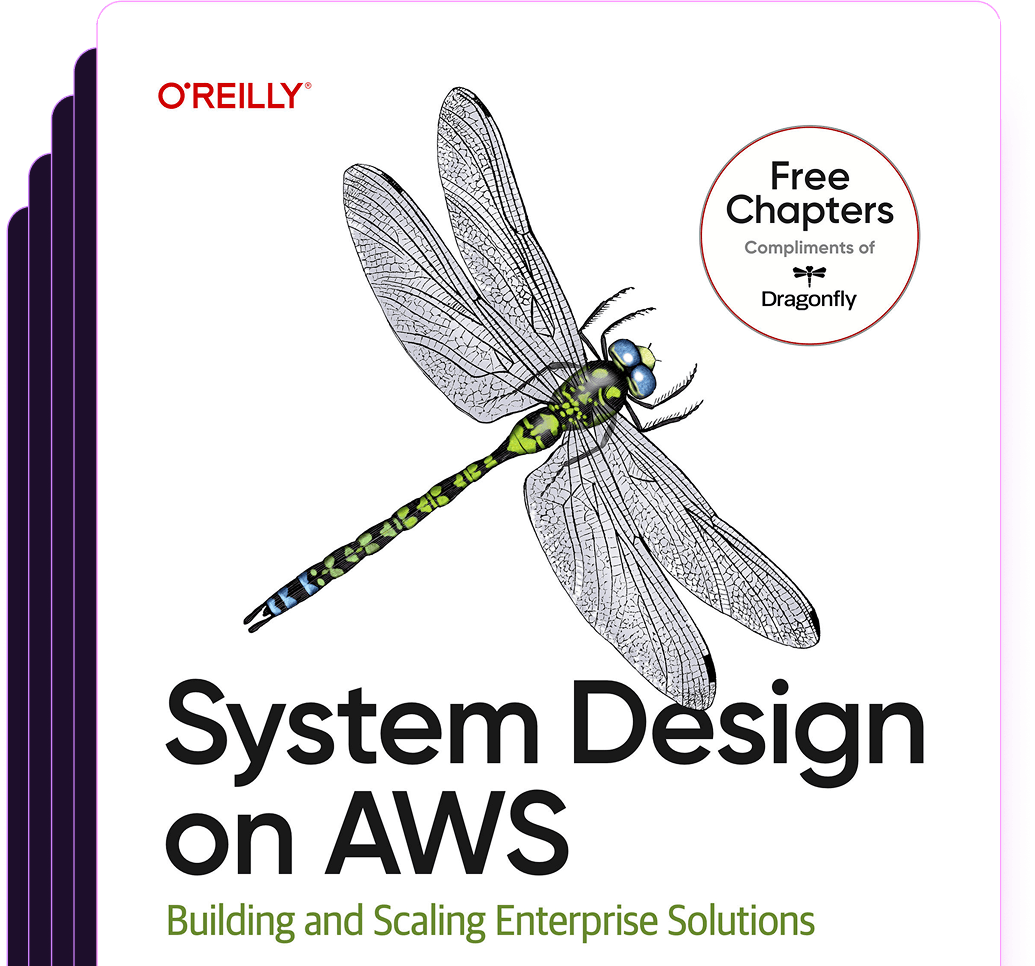
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost