Redis HSETNX in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
The HSETNX
command in Redis is used to set a field in a hash to a value, but only if the field does not already exist. In Node.js, it's commonly used to avoid overwriting existing data in a hash.
Code Examples
Here is an example using node-redis
library:
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis...');
});
// Attempt to set field 'field1' of hash 'hash1'
client.hsetnx('hash1', 'field1', 'value1', function(err, reply) {
if(err){
console.error('Error:', err);
} else {
console.log('Reply:', reply);
}
});
In this example, we're trying to set the field field1
to value1
of hash1
. If field1
does not already exist, the operation is successful and reply
will be 1. If field1
already exists, the operation is unsuccessful and reply
will be 0.
Best Practices
- Always check for errors in the callback function to handle any issues that may arise during the operation.
- Use
HSETNX
when you want to ensure that no existing data in the hash is overwritten.
Common Mistakes
- Remember that
HSETNX
only sets the field if it doesn't exist. If you need to overwrite the field regardless of its current state, useHSET
. - Be aware that Redis operations are atomic. Even if other requests are made simultaneously, each operation will be completed fully before the next starts.
FAQs
Q: What is the difference between HSET and HSETNX?
A: HSET
sets the value of a field in a hash, regardless of whether it already exists. HSETNX
, on the other hand, only sets the value if the field does not exist.
Q: What happens if an error occurs during the operation?
A: If an error occurs (for example, if there's a problem with the connection), it will be passed to the callback function as the first argument. You should always include error handling logic in your callback functions.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
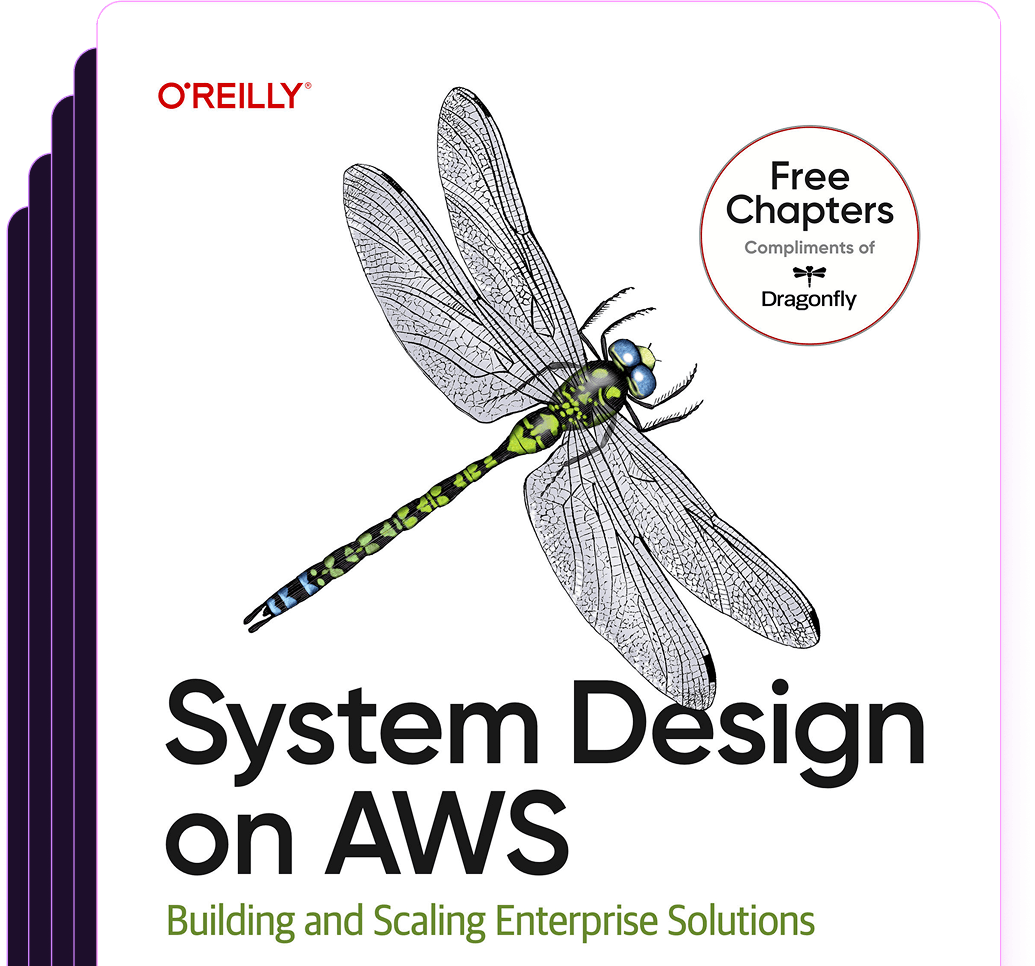
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost