Redis HINCRBY in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
Redis HINCRBY
command is used to increase the number associated with a specified field in a hash stored at a key. The increment value can be both positive and negative. In Node.js, this operation is commonly used in scenarios where you need to increment or decrement a numeric value atomically, such as keeping track of user scores, page views, or any other numerical metrics.
Code Examples
Let's say we have a hash "user_scores" that keeps track of scores for users, and we want to increment the score of "john".
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis...');
});
client.hincrby('user_scores', 'john', 5, function(err, reply) {
if (err) {
console.error(err);
} else {
console.log(`John's new score is ${reply}`);
}
});
In the example above, we're connecting to Redis from Node.js using the redis
module. After a successful connection, we're using the hincrby
method to increment "john"'s score by 5 in the "user_scores" hash. If the operation succeeds, it will return the new score.
Best Practices
- It's good practice to handle errors during the execution of the
HINCRBY
operation. This could be due to network issues or data type mismatches (e.g., attempting to increment a non-numeric value). - Remember that
HINCRBY
works only on hashes. Ensure you are not trying to use it on other data types (like lists, sets).
Common Mistakes
- One common mistake is to assume that the field exists and is a number. If the field does not exist, Redis assumes its value is 0 before the operation. However, if the field contains non-numeric values, an error will be returned.
FAQs
1. Can HINCRBY
be used on non-integer values? No, HINCRBY works only with integer values. For floating-point numbers, use HINCRBYFLOAT
.
2. What happens if the key or field does not exist? If the key does not exist, a new hash with the key-field-value relation is created. If the field doesn't exist, it is created with a value of 0 before the operation.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
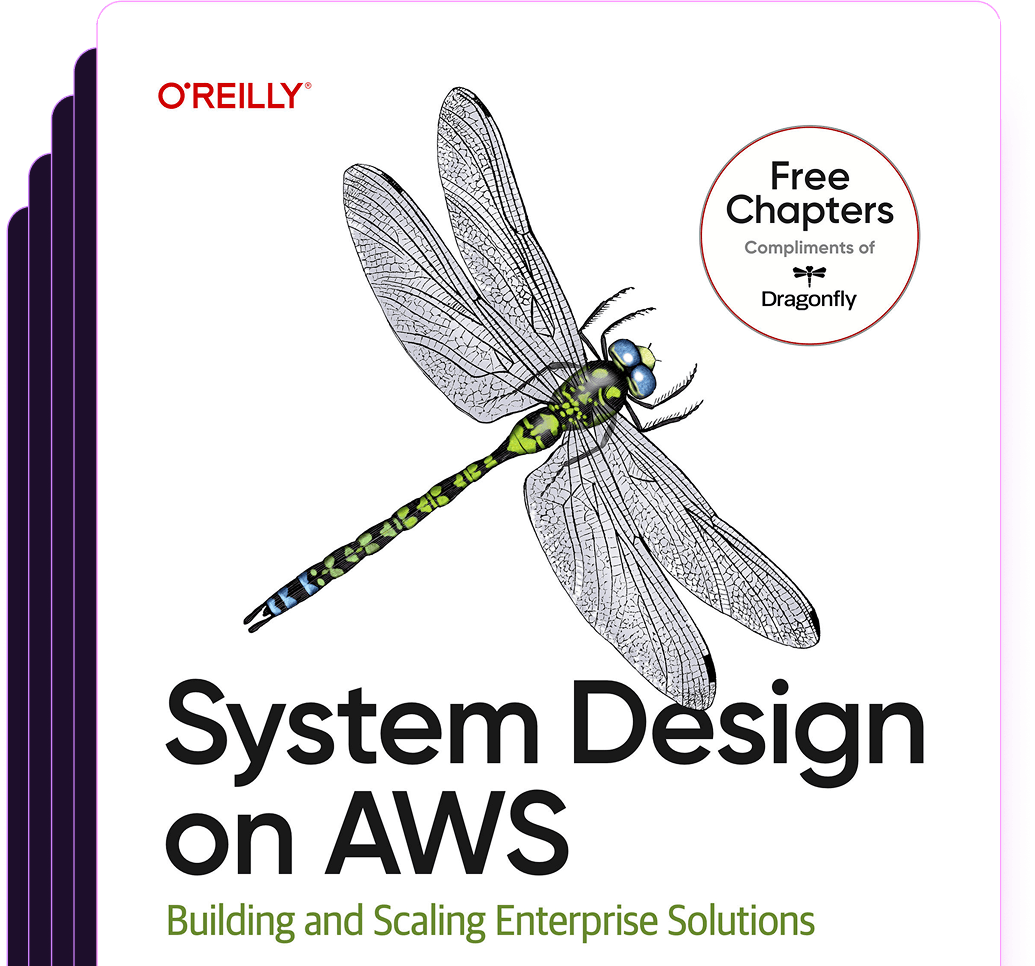
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost