Redis ZREMRANGEBYSCORE in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
The ZREMRANGEBYSCORE
command in Redis is used to remove all elements in a sorted set stored at a key with scores within the given range. This is particularly useful in scenarios such as:
- Cleaning up outdated entries from leaderboard data that fall below a certain score threshold.
- Removing expired session data where scores represent timestamps.
- Managing windows of time series data, ensuring only relevant data points are kept for processing.
Code Examples
Example 1: Removing Players with Low Scores from a Leaderboard CODE_BLOCK_PLACEHOLDER_0
This example removes all players with scores less than 50 from the 'leaderboard' sorted set. Scores are assumed to be integers.
Example 2: Clearing Expired Sessions CODE_BLOCK_PLACEHOLDER_1
In this example, all session entries with timestamps (scores) up to 1622520000 are removed, effectively clearing out expired sessions.
Best Practices
- Ensure that the use of
ZREMRANGEBYSCORE
does not block the server for large sorted sets by breaking up the job if possible or running it during low-traffic periods. - Regularly index and clean up your data using
ZREMRANGEBYSCORE
to maintain optimal performance and storage efficiency.
Common Mistakes
- Not handling errors which might arise during the execution of
zremrangebyscore
, potentially leading to unmanaged states in the application. - Incorrect usage of scoring ranges can lead to unintentional deletion of data. Always double-check the score boundaries specified in the command.
FAQs
Q: What does -inf
and +inf
mean in the context of ZREMBRANGEBYSCORE
? A: In Redis, -inf
and +inf
are used to specify the minimum and maximum possible scores, respectively, creating a range that includes all possible scores.
Q: Is ZREMRANGEBYSCORE
an atomic operation? A: Yes, ZREMRANGEBYSCORE
is atomic. This means it will execute as a single uninterrupted operation, ensuring data integrity.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
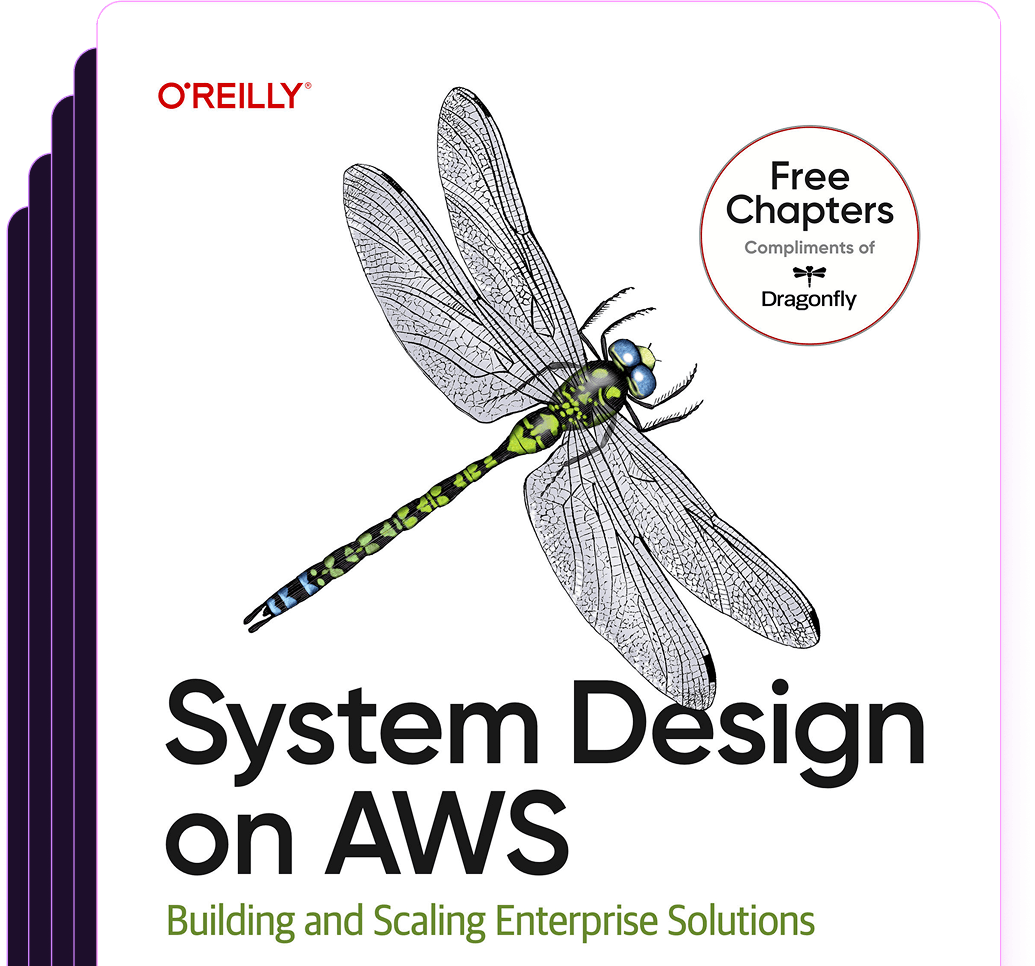
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost