Redis ZREVRANGE in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
ZREVRANGE
is a Redis command used to retrieve a range of members from a sorted set in descending order by their scores. It's particularly useful in scenarios such as leaderboards, where you want to display the top scores or items with the highest ranking first.
Code Examples
Example 1: Retrieving Members from a Sorted Set CODE_BLOCK_PLACEHOLDER_0
This example connects to the Redis server and retrieves all members of the sorted set 'game_scores' from the highest to the lowest score.
Example 2: Retrieving Members with Their Scores CODE_BLOCK_PLACEHOLDER_1
In this example, not only are the members retrieved, but their associated scores are also included, useful for displaying a leaderboard where both the player's name and score are needed.
Best Practices
- Error Handling: Always include error handling when working with network-based operations like database calls to handle unexpected issues gracefully.
- Connection Management: Ensure that Redis client connections are managed properly — opened before use and closed after operations are complete.
Common Mistakes
- Ignoring Promises: Redis operations in Node.js are asynchronous and return promises. Ignoring these promises can lead to situations where operations do not execute as expected.
FAQs
Q: Can I specify the exact range of indices for zRevRange
? A: Yes, the first two parameters of zRevRange
allow you to specify the starting and ending indices, respectively. For example, zRevRange('key', 0, 4)
retrieves the top 5 elements.
Q: What does the WITHSCORES
option do in the zRevRange
call? A: The WITHSCORES
option modifies the command to return both elements and their scores from the sorted set. Each member of the set is followed by its score in the output array.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
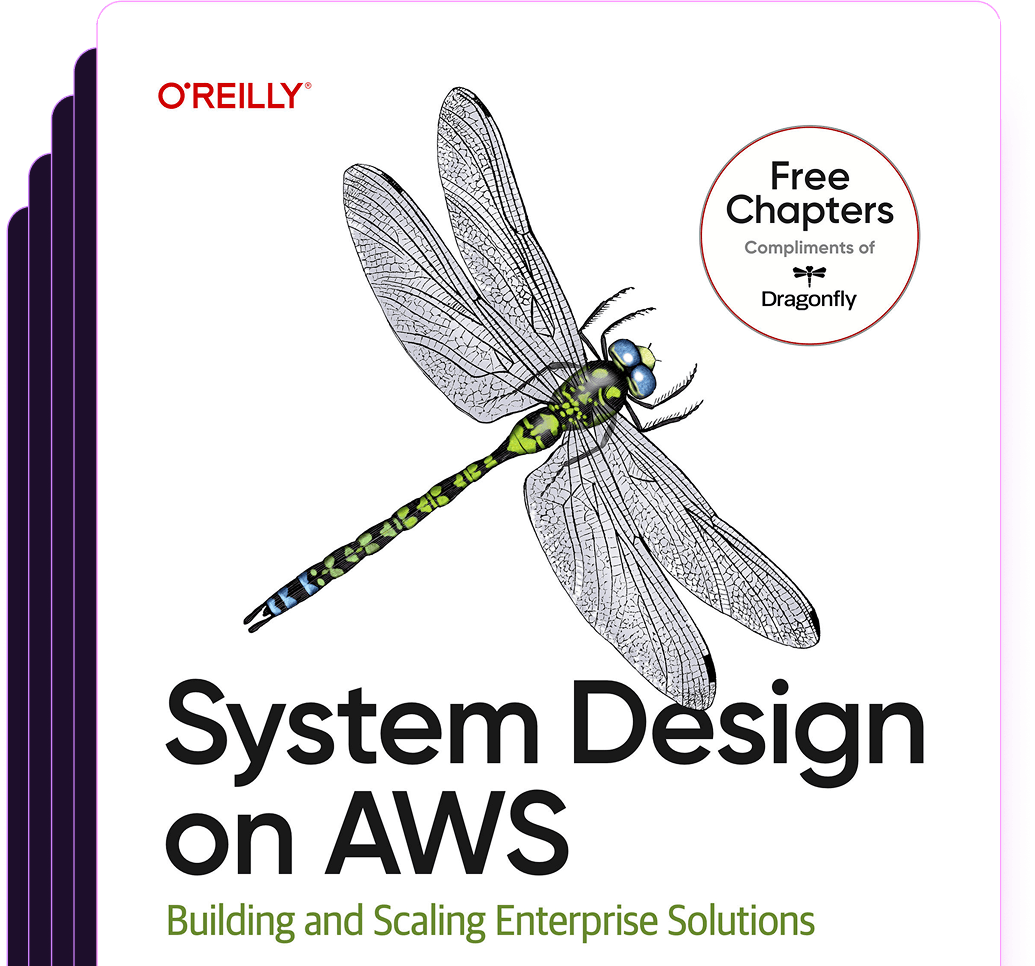
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost