PHP Redis: Get List (Detailed Guide w/ Code Examples)
Use Case(s)
Redis list data type provides efficient operations to work with lists. A common use case is when you need a queue, stack, or even a double-ended queue (deque).
Code Examples
To manipulate and retrieve elements from the Redis list in PHP, you would typically use Predis or PhpRedis extension. Here are some examples of these in action.
Example 1: Using PhpRedis extension: CODE_BLOCK_PLACEHOLDER_0
In this example, we're connecting to a Redis instance, then using the lRange method to get all elements currently stored in 'mylist'. The list content is then printed.
Example 2: Using Predis library: CODE_BLOCK_PLACEHOLDER_1
Similarly, this code connects to the Redis instance and retrieves all elements from 'mylist'. It's essentially the same operation as the previous example but done using the Predis library.
Best Practices
When working with lists in Redis:
- Keep track of the size of your lists if their lengths are not limited, because they can grow infinitely and consume a lot of memory.
- Use the appropriate Redis commands depending upon whether you are treating your lists as queues, stacks, or deques.
Common Mistakes
- Trying to get items from an non-existent list. Always ensure the list exists before trying to retrieve elements from it.
- Not handling the case when the list is empty. This could lead to unexpected behavior in your application.
FAQs
Q: Can I get a range of elements from the list instead of the entire list? A: Yes, you can use the lRange
function with different indexes to get a specific range of elements. The syntax is lRange('listname', start_index, stop_index)
.
Q: What happens if I try to get an item from an empty list? A: If you try to retrieve an item from an empty list in Redis, it will return 'nil' or NULL.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- PHP Redis: Get All Keys Matching Pattern
- PHP Redis: Get All Keys Starting With
- PHP Redis: Get Current Memory Usage
- PHP Redis: Getting Key Type
- PHP Redis - Get Hash Values at a Key
- PHP Redis: Getting All Databases
- Redis Get All Hash Keys in PHP
- Getting Memory Stats in PHP Redis
- Checking if a Key Exists in Redis using PHP
- Getting Redis Configuration Settings in PHP
- Getting Redis Key by Value in PHP
- Getting Number of Subscribers in Redis with PHP
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
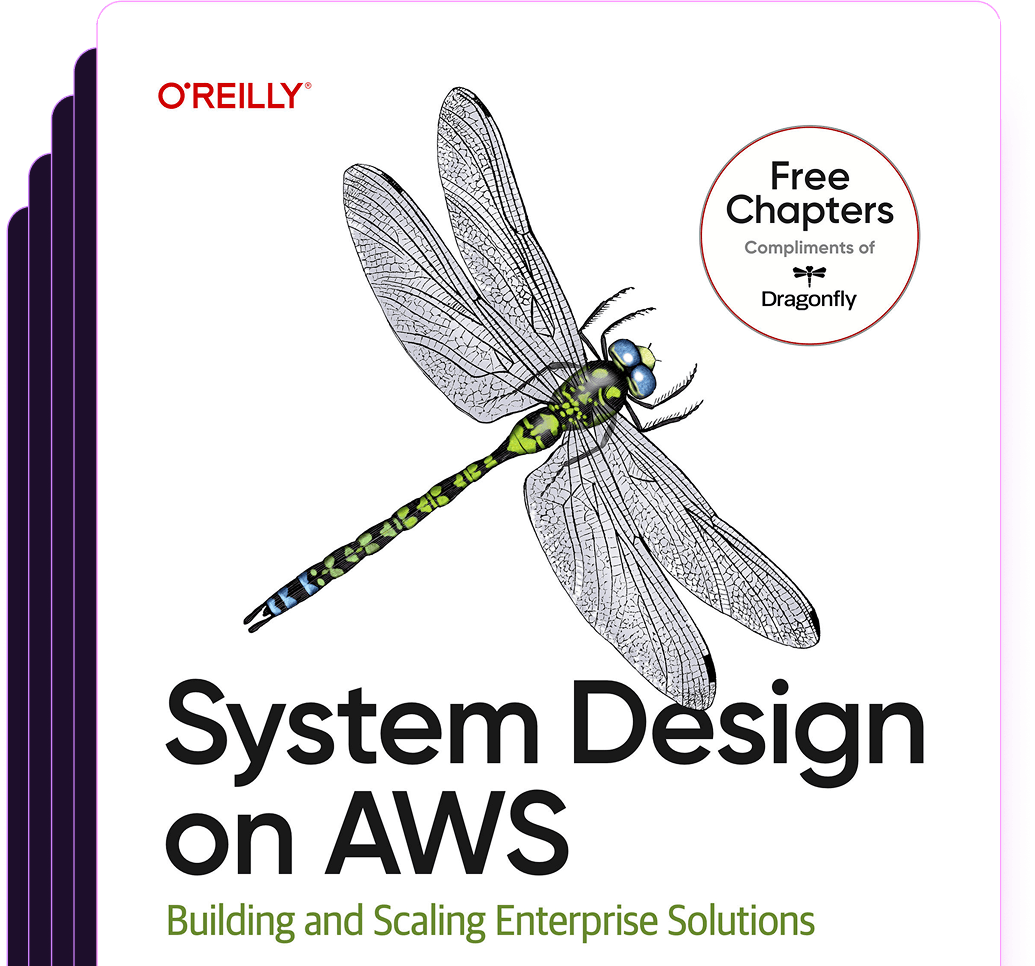
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost