Redis ZADD in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The ZADD
command in Redis is used to add one or more members to a sorted set, or to update the score for existing members. This is commonly used in scenarios such as:
- Leaderboards: Tracking high scores across users where scores can change frequently.
- Time-series data: Storing events with a timestamp as the score to fetch recent events quickly.
- Priority Queue: Managing tasks based on priority where the score determines the task's urgency.
Code Examples
Basic Usage of ZADD
The following example demonstrates how to use ZADD
to add elements to a sorted set and retrieve them in order with Python using the redis-py
library.
import redis
# Establish connection
r = redis.Redis(host='localhost', port=6379, db=0)
# Adding elements to the sorted set
r.zadd('leaderboard', {'Alice': 50, 'Bob': 40})
# Retrieving all elements in the sorted set sorted by score
print(r.zrange('leaderboard', 0, -1, withscores=True))
In this example, we add scores for Alice and Bob to the 'leaderboard' sorted set. The zrange
function is used to fetch and print all items and their scores, sorted from lowest to highest.
Updating Scores
You can also update the scores of existing members simply by reusing the zadd
method:
# Update Bob's score
r.zadd('leaderboard', {'Bob': 60})
# Retrieve updated leaderboard
print(r.zrange('leaderboard', 0, -1, withscores=True))
Here, Bob's score is updated to 60, and fetching the leaderboard again reflects this change.
Best Practices
- When working with large datasets, consider using
ZINCRBY
for incrementing the score of existing members instead of setting new values to avoid overhead. - Regularly back up your Redis data if it includes crucial information such as user scores or priorities.
Common Mistakes
- Not handling connection errors gracefully. Always ensure you have error handling in place when connecting to or interacting with Redis.
- Forgetting that scores can be floating point numbers, not just integers. This is particularly important for time-based scores or fractional increments.
FAQs
Can I use negative scores with ZADD?
Yes, Redis supports both positive and negative floating-point values as scores in sorted sets.
How can I remove elements from a sorted set in Redis?
To remove elements, you can use the ZREM
command. For example, r.zrem('leaderboard', 'Alice')
removes Alice from the 'leaderboard'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis ZCOUNT in Python
- Redis ZRANGEBYLEX in Python
- Redis ZRANGE in Python
- Redis ZREM in Python
- Redis ZREVRANGEBYSCORE in Python
- Redis ZREMRANGEBYSCORE in Python
- Redis ZREVRANGE in Python
- Redis ZUNIONSTORE in Python
- Redis ZRANK in Python
- Redis ZREVRANK in Python
- Redis ZSCORE in Python
- Redis ZRANGEBYSCORE in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
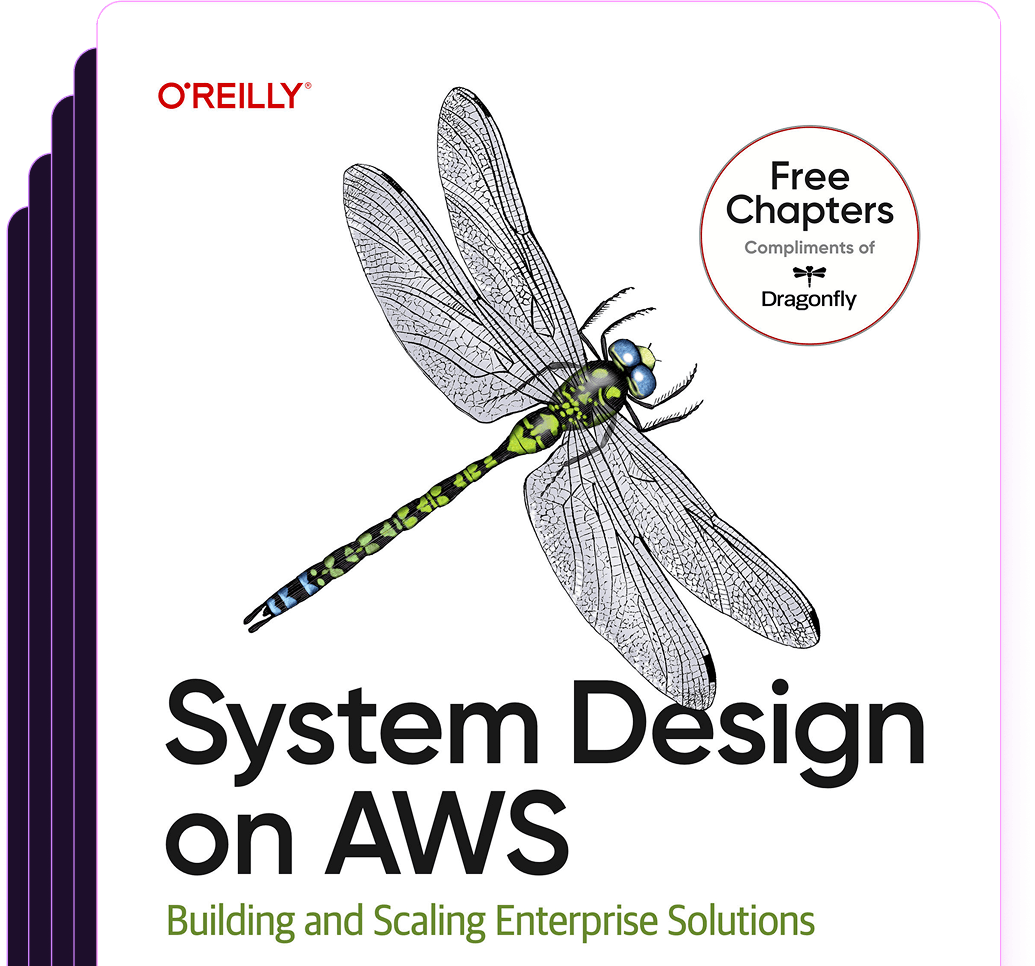
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost