Redis ZRANGEBYSCORE in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The ZRANGEBYSCORE
command in Redis is used to retrieve a subset of elements in a sorted set stored within a specified score range. Common use cases include:
- Retrieving leaderboard entries within a specific scoring range.
- Filtering items in a timeline or events within a given timeframe if scores represent timestamps.
- Segmenting data dynamically based on score thresholds in real-time applications.
Code Examples
Here is how you can use the ZRANGEBYSCORE
command in Python using the redis-py
client library.
Example 1: Basic Usage
Retrieve all members with scores between 10 and 20.
import redis
# Connect to Redis
client = redis.Redis(host='localhost', port=6379, db=0)
# Add some data to the sorted set
client.zadd('myset', {'a': 15, 'b': 10, 'c': 20, 'd': 25})
# Retrieve scores between 10 and 20
result = client.zrangebyscore('myset', 10, 20)
print(result) # Output: [b'b', b'a', b'c']
Example 2: With Additional Options
Retrieve members and their scores in descending order, limiting to the first two results.
result_with_scores = client.zrangebyscore('myset', 10, 20, withscores=True, start=0, num=2, desc=True)
print(result_with_scores) # Output: [(b'c', 20.0), (b'a', 15.0)]
Best Practices
- Index Maintenance: Ensure that sorted sets are indexed correctly and efficiently to optimize for read-heavy operations like
ZRANGEBYSCOR
. - Memory Management: Be mindful of memory usage when storing large sorted sets and retrieving large ranges.
Common Mistakes
- Ignoring Score Precision: Redis scores can be floating-point numbers. Not accounting for precision can lead to unexpected results.
- Over-fetching Data: Retrieving very large ranges without proper pagination can lead to heavy memory and network load.
FAQs
Q: What happens if no elements are found within the specified score range? A: The command returns an empty list.
Q: Can I use exclusive ranges in ZRANGEBYSCORE
? A: Yes, you can specify exclusive ranges using "("
and ")"
. For example, client.zrangebyscore('myset', '(10', 20)
retrieves elements with scores >10 and <=20.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
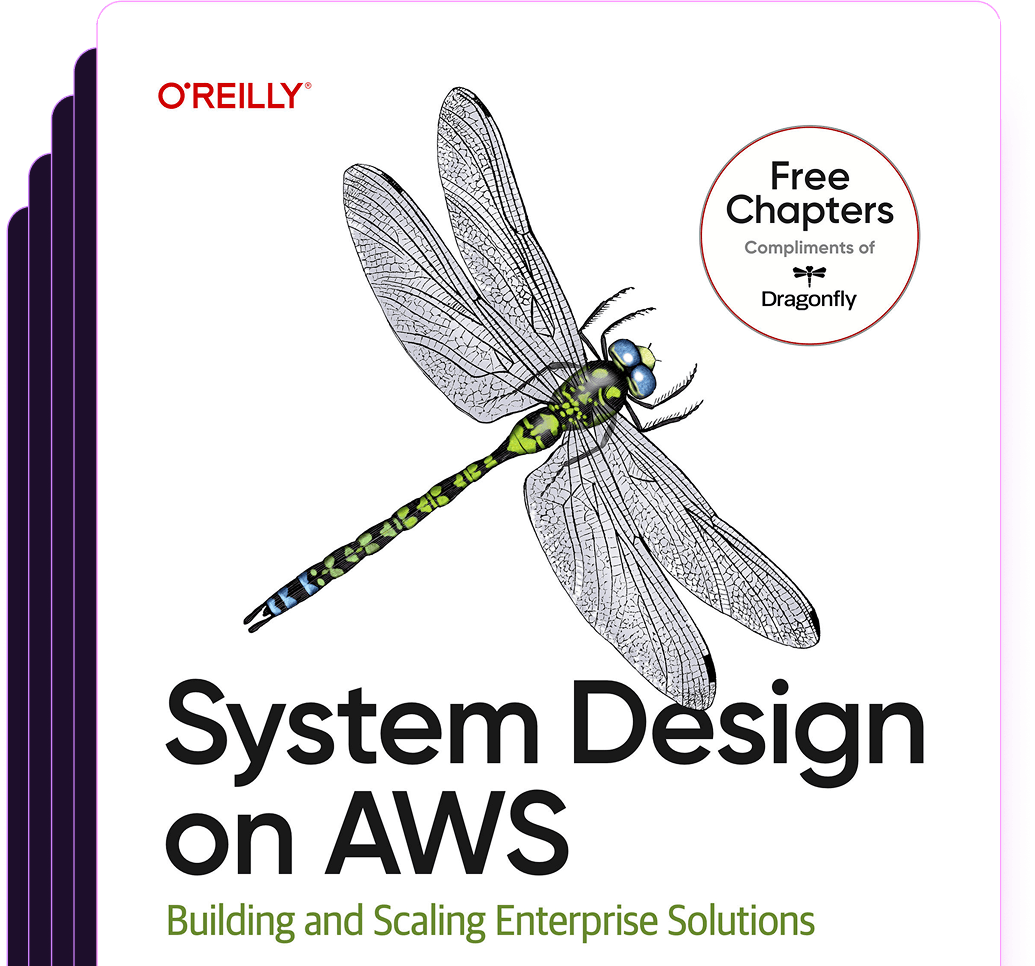
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost