Redis Sorted Set: Sorting by Timestamp (Detailed Guide w/ Code Examples)
Use Case(s)
- Storing and retrieving time-series data, such as events logged with timestamps.
- Implementing leaderboards that update over time.
- Scheduling tasks for future execution based on their timestamps.
Code Examples
Python
Using the redis-py
library to add and retrieve members sorted by timestamp:
import redis
import time
r = redis.Redis(host='localhost', port=6379, db=0)
# Add a member with the current timestamp
timestamp = time.time()
r.zadd('my_sorted_set', { 'member1': timestamp })
# Retrieve members sorted by score (timestamp)
members = r.zrange('my_sorted_set', 0, -1, withscores=True)
print(members)
Node.js
Using the ioredis
library to achieve the same goal:
const Redis = require('ioredis');
const redis = new Redis();
const timestamp = Date.now() / 1000;
// Add a member with the current timestamp
redis.zadd('my_sorted_set', timestamp, 'member1');
// Retrieve members sorted by score (timestamp)
redis.zrange('my_sorted_set', 0, -1, 'WITHSCORES', (err, result) => {
if (err) throw err;
console.log(result);
});
Golang
Using the go-redis/redis/v8
package for this task:
package main
import (
"context"
"fmt"
"time"
"github.com/go-redis/redis/v8"
)
func main() {
ctx := context.Background()
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
timestamp := float64(time.Now().Unix())
// Add a member with the current timestamp
rdb.ZAdd(ctx, "my_sorted_set", &redis.Z{Score: timestamp, Member: "member1"})
// Retrieve members sorted by score (timestamp)
members, _ := rdb.ZRangeWithScores(ctx, "my_sorted_set", 0, -1).Result()
for _, member := range members {
fmt.Println(member.Member, member.Score)
}
}
Best Practices
- Use proper expiration policies to avoid unbounded growth of sorted sets when storing time-series data.
- Consider using Redis Streams for more complex time-series use cases which might involve more sophisticated querying or high write throughput.
Common Mistakes
- Forgetting to handle the case where no elements exist in the sorted set, leading to potential errors in your application.
- Misinterpreting Unix timestamps (seconds vs milliseconds), which can lead to incorrect sorting.
FAQs
Q: Can I use Redis Sorted Sets to expire old data automatically? A: No, Redis Sorted Sets do not support automatic expiry of individual elements. You would need to manually remove elements based on their score or use other structures like Redis Streams for time-bound data retention.
Q: What are the alternatives to using timestamps for scores? A: Other than timestamps, you can use any numerical value that represents the importance or order of an element, such as scores in a game leaderboard or priority levels in a task queue.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Get Highest Score
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Limit Size
- Redis Sorted Set: Same Score
- Redis Sorted Set: Sorting by Multiple Fields
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Expire Key
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
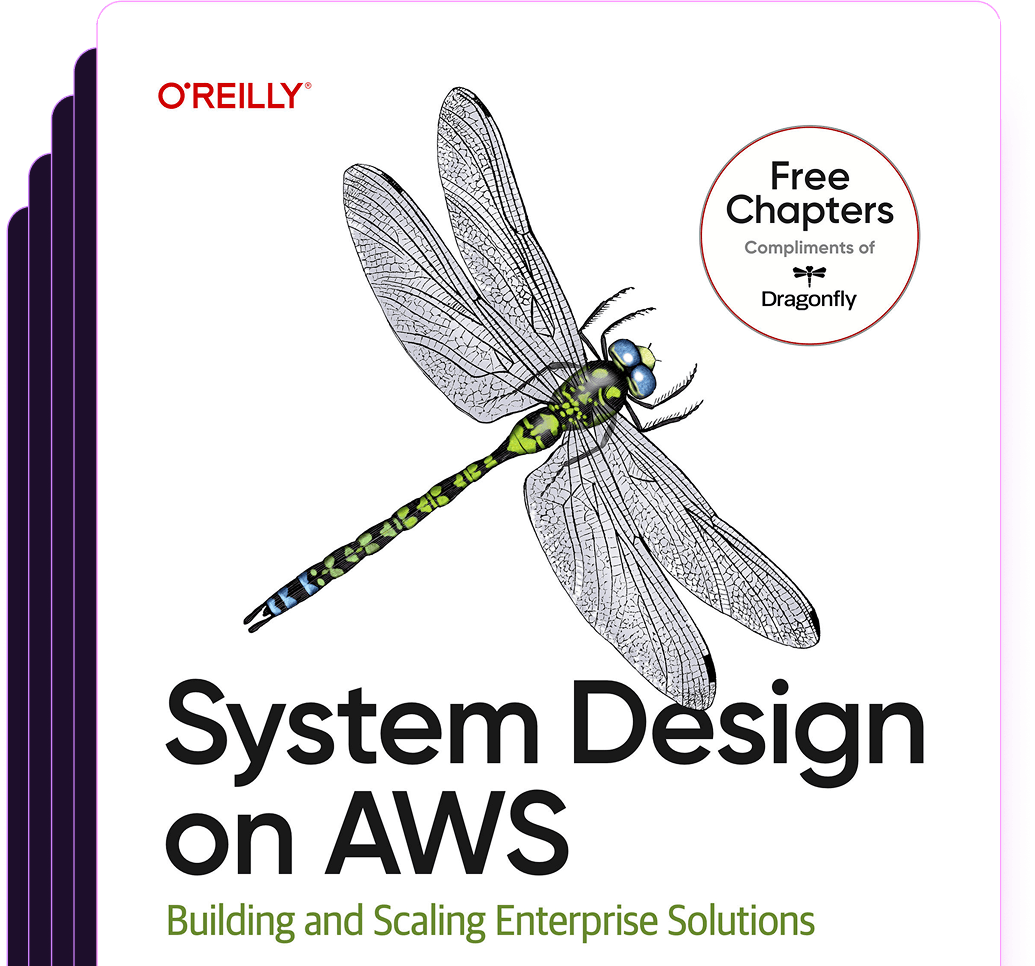
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost