Redis Sorted Set: Multiple Scores (Detailed Guide w/ Code Examples)
Use Case(s)
- Ranking systems where multiple criteria (scores) need to be considered.
- Aggregating scores from different sources for a composite ranking.
- Implementing leaderboards that require more complex scoring mechanisms than a single numeric value.
Code Examples
To handle multiple scores in Redis sorted sets, you typically need to combine them into a single score or use workarounds since Redis does not natively support multiple scores per element.
Python
Using redis-py
and a composite key: CODE_BLOCK_PLACEHOLDER_0
Node.js
Using ioredis
and a composite key: CODE_BLOCK_PLACEHOLDER_1
Golang
Using go-redis
and a composite key: CODE_BLOCK_PLACEHOLDER_2
Common Mistakes
- Failing to account for composite score calculations can lead to incorrect rankings.
- Overcomplicating the key structure when simpler approaches may suffice.
- Not handling score precision properly, leading to unexpected sorting results.
FAQs
How do I separate original scores after combining them? You must store additional information or follow a reversible combination method (like encoding both scores into one).
Can Redis handle multiple scores natively? No, Redis doesn't support multiple scores for a single element in sorted sets directly; aggregation or combination methods are required.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Get Highest Score
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Limit Size
- Redis Sorted Set: Same Score
- Redis Sorted Set: Sorting by Multiple Fields
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Expire Key
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
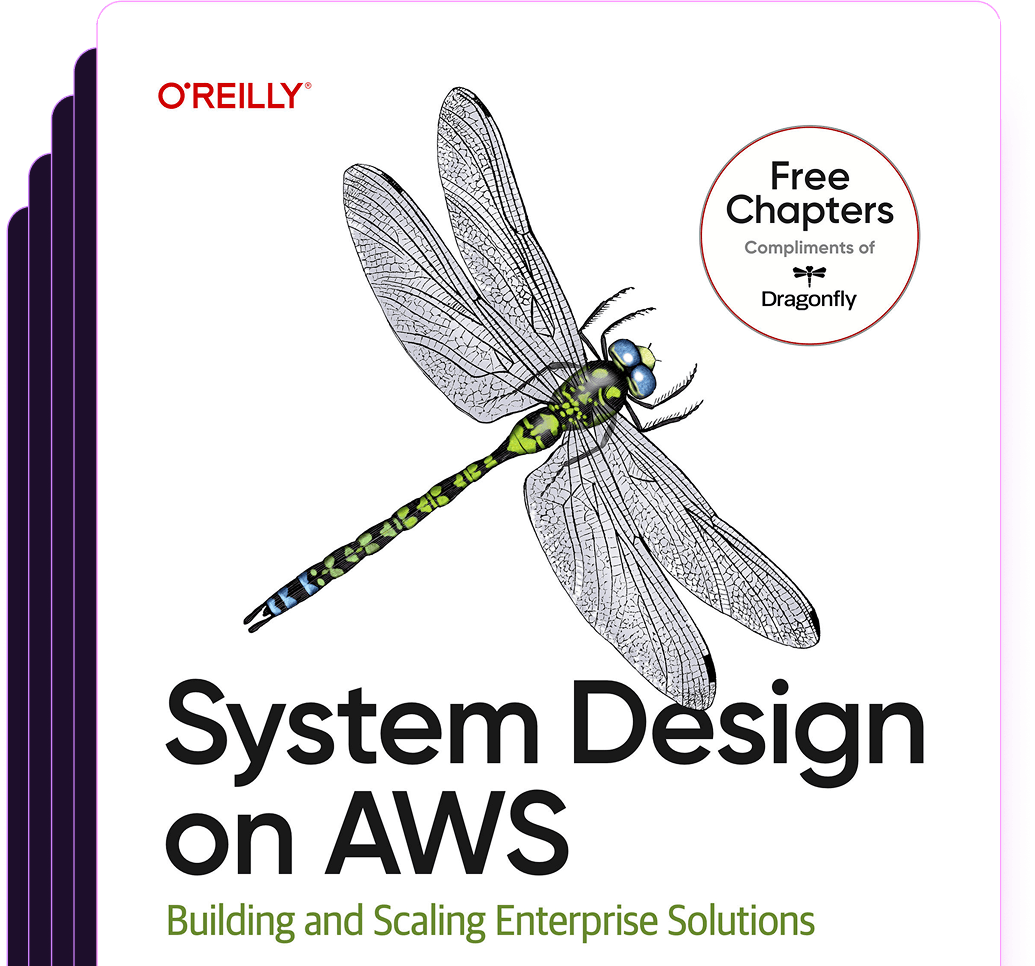
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost