Redis Sorted Set: Pagination (Detailed Guide w/ Code Examples)
Use Case(s)
- Displaying a page of results from a leaderboard.
- Fetching paginated data for infinite scroll in an application.
- Implementing paging through search results with scores.
Code Examples
Python
import redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
# Add elements to the sorted set
client.zadd('myset', {'member1': 1, 'member2': 2, 'member3': 3, 'member4': 4})
# Paginate with start and end index
start = 0
end = 2
results = client.zrange('myset', start, end, withscores=True)
print(results)
Node.js
const redis = require("redis");
const client = redis.createClient();
client.on("error", function (error) {
console.error(error);
});
client.zadd("myset", 1, "member1", 2, "member2", 3, "member3", 4, "member4");
// Paginate with start and end index
const start = 0;
const end = 2;
client.zrange("myset", start, end, "WITHSCORES", (err, result) => {
if (err) throw err;
console.log(result);
});
Golang
package main
import (
"fmt"
"github.com/go-redis/redis/v8"
"context"
)
func main() {
ctx := context.Background()
client := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
// Add elements to the sorted set
client.ZAdd(ctx, "myset", &redis.Z{Score: 1, Member: "member1"})
client.ZAdd(ctx, "myset", &redis.Z{Score: 2, Member: "member2"})
client.ZAdd(ctx, "myset", &redis.Z{Score: 3, Member: "member3"})
client.ZAdd(ctx, "myset", &redis.Z{Score: 4, Member: "member4"})
// Paginate with start and end index
start := int64(0)
end := int64(2)
results, err := client.ZRangeWithScores(ctx, "myset", start, end).Result()
if err != nil {
panic(err)
}
for _, z := range results {
fmt.Printf("%v: %f\n", z.Member, z.Score)
}
}
Best Practices
- Ensure indices used for pagination are properly calculated to avoid off-by-one errors.
- Consider using
ZREVRANGE
if you need descending order pagination. - Use
ZRANGEBYSCORE
withLIMIT
for more controlled pagination based on scores rather than indices.
Common Mistakes
- Forgetting to include the
WITHSCORES
option when needed, which can lead to confusion with results. - Using zero-based indexing incorrectly can lead to skipped or duplicated entries in pages.
- Not handling empty results correctly, which may occur at the boundaries of the dataset.
FAQs
Q: How do I handle large datasets efficiently with Redis sorted sets? A: Use cursor-based pagination with ZSCAN
to avoid loading too many elements into memory at once.
Q: Can I delete a range of elements while paginating? A: Yes, use ZREMRANGEBYRANK
to remove elements by their rank range.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Get Highest Score
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Limit Size
- Redis Sorted Set: Same Score
- Redis Sorted Set: Sorting by Multiple Fields
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Expire Key
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
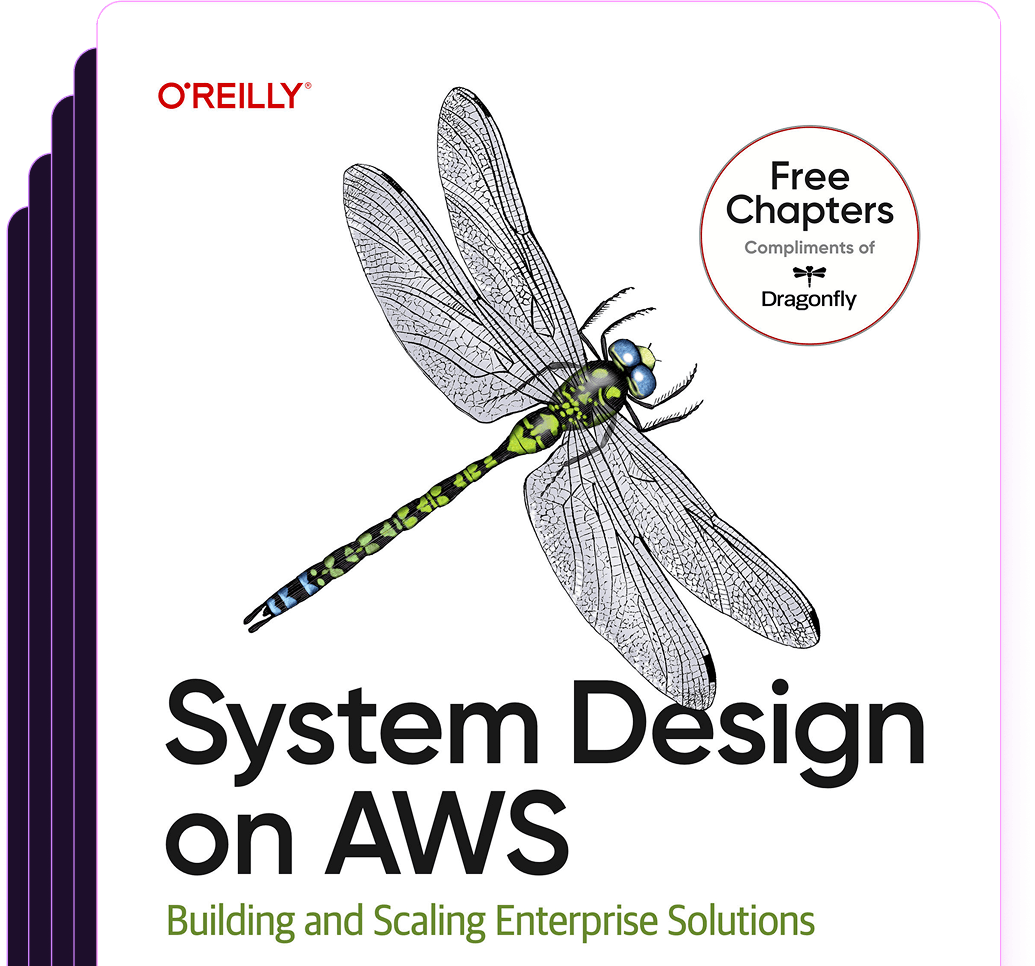
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost