Question: How can you configure a connection pool in Redis?
Answer
In order to configure a connection pool in Redis, you'll need to leverage clients that support connection pooling. Connection pooling is beneficial because it helps manage and control the number of connections that can be used at once, which can dramatically improve the performance of your Redis operations.
A popular choice for this in Python is the redis-py
library. Here's an example:
import redis
from redis.connection import BlockingConnectionPool
pool = BlockingConnectionPool(max_connections=10, timeout=10)
r = redis.Redis(connection_pool=pool)
In the above code, we're establishing a connection pool using BlockingConnectionPool
from the redis-py
library. We specify the maximum allowed connections (max_connections
) to 10 and a timeout
of 10 seconds. We then create a new Redis connection r
that will utilize this connection pool.
For Node.js, the node-redis
package can be used similarly. The createClient
method can accept an options object where the connection pool size can be defined:
var redis = require('redis');
var client = redis.createClient({
host: 'localhost',
port: 6379,
max_clients: 30,
perform_checks: false,
database: 0,
});
In the above Node.js example, we're creating a connection pool with 30 maximum clients.
Keep in mind that the actual configuration of the connection pool will depend on the specific Redis client being used so refer to the appropriate documentation for those details.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
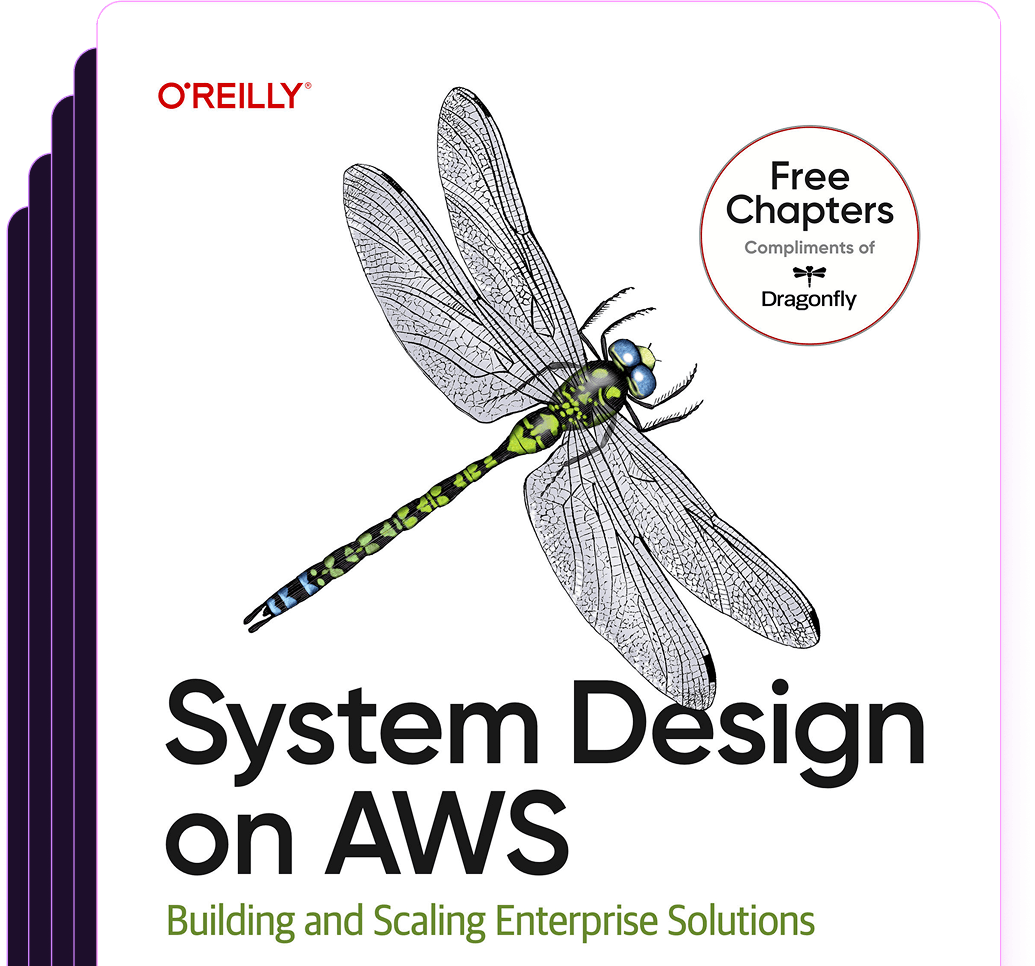
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost