Question: Does Memcached support encryption?
Answer
By default, Memcached doesn't support encryption natively. However, there are several ways to encrypt data stored in Memcached.
1. Encrypting data before storing it in Memcached
One way to encrypt data is by encrypting it using an encryption library like OpenSSL and then storing the encrypted data in Memcached. When retrieving the data, decrypt it using the same encryption library.
Here's an example of how to use OpenSSL to encrypt and decrypt data:
import OpenSSL
import memcache
key = b"my_secret_key"
value = b"My secret value"
# Encrypt the value
cipher = OpenSSL.crypto.Cipher(alg="aes_256_cbc", key=key, iv=b"0")
encrypted_value = cipher.update(value) + cipher.final()
# Store the encrypted value in Memcached
mc = memcache.Client(["127.0.0.1:11211"])
mc.set("my_key", encrypted_value)
# Retrieve the encrypted value from Memcached and decrypt it
encrypted_value = mc.get("my_key")
cipher = OpenSSL.crypto.Cipher(alg="aes_256_cbc", key=key, iv=b"0", op=OpenSSL.crypto.DECRYPT_MODE)
decrypted_value = cipher.update(encrypted_value) + cipher.final()
2. Using a proxy server
Another way to encrypt data is by using a proxy server like Stunnel or Nginx, which can act as an SSL/TLS termination point for Memcached clients. The proxy server can encrypt the data sent from the client to the server and vice versa.
Here's an example of how to set up Stunnel to encrypt data for Memcached:
- Install Stunnel:
apt-get install stunnel4
- Create a new configuration file
/etc/stunnel/memcached.conf
with the following contents:
```
[memcached]
client = yes
accept = 127.0.0.1:11212
connect = 127.0.0.1:11211
```
- Start Stunnel:
service stunnel4 start
- Configure your Memcached client to use the encrypted port
11212
instead of the default port11211
.
Note that using encryption can increase the CPU overhead of your Memcached server and reduce performance. Therefore, it's important to benchmark your system and adjust your configuration accordingly.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Memcached Questions (and Answers)
- Does Memcached expire?
- Does Memcached write to disk?
- What is the location of Memcached error log?
- How does Memcached consistent hashing work?
- Does restarting Memcached clear cache?
- How to pronounce Memcached?
- How to check Memcached version in Linux?
- How to install Memcached in CentOS 7?
- How is Memcached implemented?
- How to clear Memcached data?
- How does Memcached work in PHP?
- How to install Memcached on Windows?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
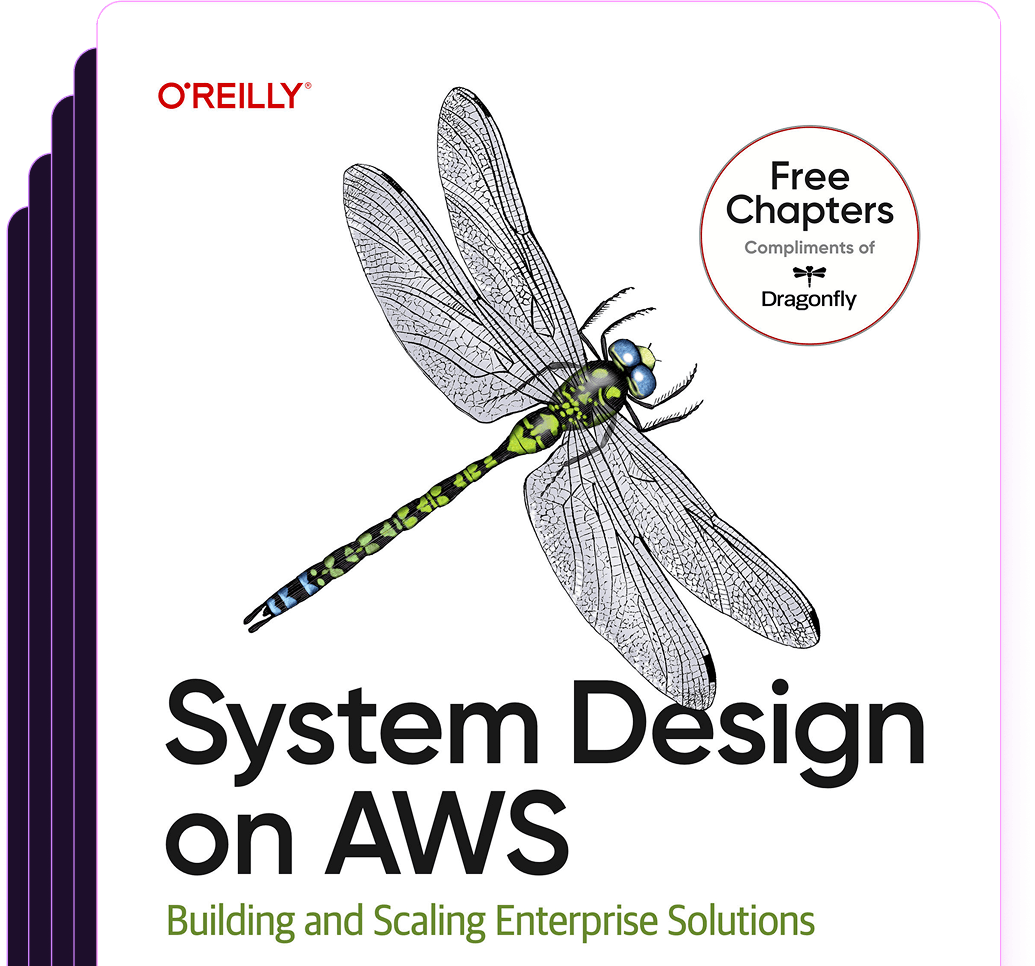
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost