Question: How to use Memcached in Laravel?
Answer
Laravel provides an easy way to use caching mechanisms to improve the performance of web applications. Memcached is one of the caching drivers supported by Laravel. In this guide, we will see how to configure and use Memcached as a caching driver in Laravel.
Step 1: Install Memcached
First, you need to install the Memcached server on your system. You can install it using the package manager of your operating system. For example, if you're using Ubuntu, you can install it with the following command:
```
sudo apt-get install memcached
```
Step 2: Install Memcached PHP Extension
You also need to install the Memcached PHP extension for Laravel to communicate with the Memcached server. You can install it using the following command:
```
sudo apt-get install php-memcached
```
Step 3: Configure Memcached in Laravel
Next, you need to configure Memcached as the cache driver in Laravel. Open the .env
file at the root of your Laravel project and set the CACHE_DRIVER
variable to memcached
. You can also set other configuration options like the Memcached server host and port.
```
CACHE_DRIVER=memcached
MEMCACHED_HOST=<memcached-server-host>
MEMCACHED_PORT=<memcached-server-port>
```
Step 4: Use Memcached in Laravel
Now that Memcached is configured in Laravel, you can use it to cache data in your application. The simplest way to use caching in Laravel is through the cache()
function. Here's an example:
// Retrieve data from cache
$data = cache('data');
if (!$data) {
// Data not found in cache, retrieve and cache it
$data = DB::table('my_table')->get();
cache(['data' => $data], 60); // Cache for 60 minutes
}
// Use the data
foreach ($data as $row) {
// Do something with the row
}
In this example, we retrieve data from the cache using the cache()
function. If the data is not found in the cache, we retrieve it from the database and cache it for 60 minutes using the cache()
function again.
That's it! You now know how to use Memcached as a caching driver in Laravel.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Memcached Questions (and Answers)
- Does Memcached expire?
- Does Memcached write to disk?
- What is the location of Memcached error log?
- How does Memcached consistent hashing work?
- Does Memcached support encryption?
- How to check Memcached version in Linux?
- How to clear Memcached data?
- How to install Memcached on Windows?
- How much memory RAM does Memcached use?
- How to install Memcached on Ubuntu?
- How to check Memcached memory usage?
- Is Memcached faster than Redis?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
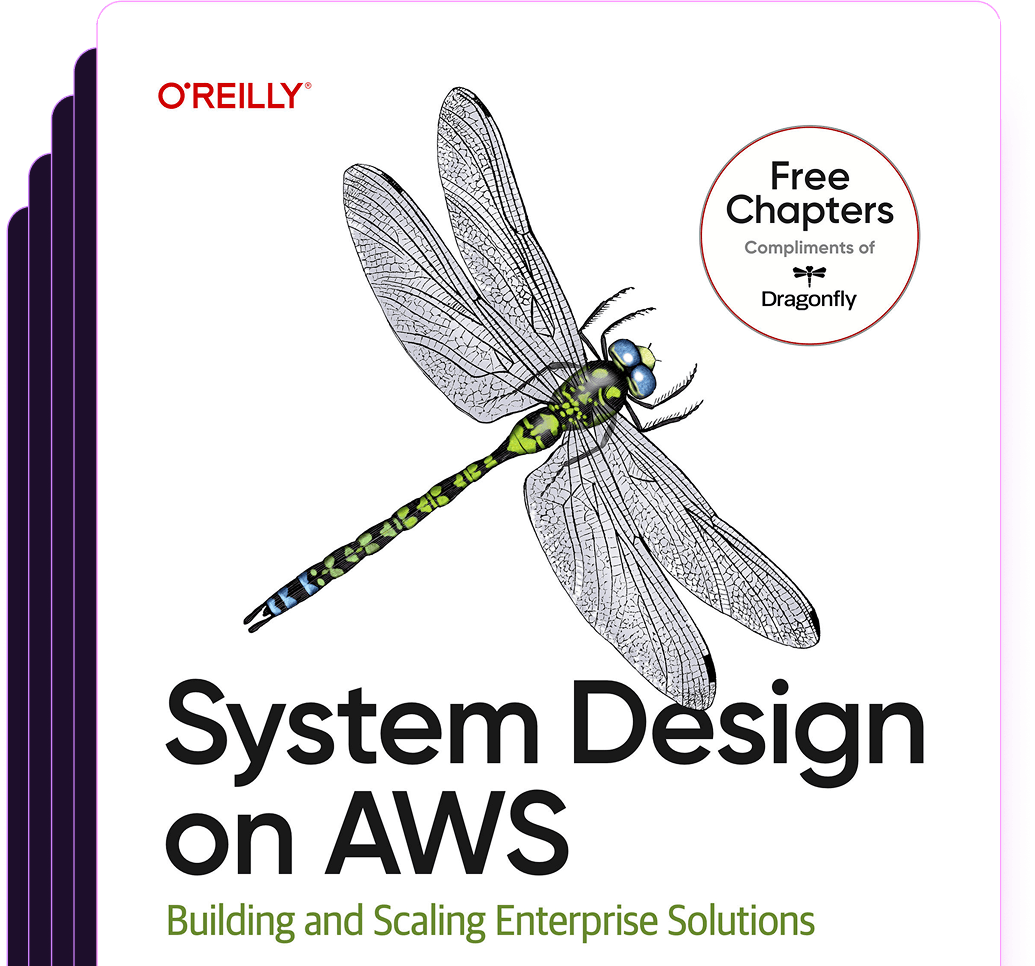
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost