Question: How does Redis store data in memory?
Answer
Redis is an open-source, in-memory data structure store that uses RAM for storage. Redis supports different types of data structures including strings, hashes, lists, sets, sorted sets with range queries, bitmaps, hyperloglogs, and geospatial indexes with radius queries.
Here's a simplified explanation of how Redis stores data in memory:
- Basic Storage: When you set a key-value pair in Redis, it stores this pair in a dictionary-like data structure in RAM. The keys are always strings but the values can be multiple types of data.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('foo', 'bar')
print(r.get('foo')) # output: 'bar'
- Data Expiration: Redis allows keys to have a "time to live" (TTL). After this time has passed, Redis will automatically remove the key-value pair from memory.
r.setex('key', 'value', 10) # set key-value with TTL of 10 seconds
- Persistence: Although Redis is an in-memory database, it can persist data to disk. It implements this through two methods: RDB (Redis Database file) and AOF (Append Only File). While RDB takes snapshots of your dataset at specified intervals, AOF logs every write operation received by the server.
- Replication: Redis can replicate data to any number of slaves, which helps spread read operations and provides a backup in case the master goes down.
- Data Structures: Redis natively supports various complex data structures. This includes Lists, Sets, Sorted Sets, Hashes etc. These data structures are stored in memory and offer various operations with different time complexities.
For example, storing a hash in Redis:
r.hset("hash_key", "subkey1", "value1")
r.hset("hash_key", "subkey2", "value2")
# get all keys and values in the hash
print(r.hgetall("hash_key")) # output: {'subkey1': 'value1', 'subkey2': 'value2'}
The exact details of how Redis accomplishes these tasks are quite complex and involve intricate algorithms and data structures such as skip lists, prefix trees, hash tables, and so on. Also note, the efficiency of Redis greatly relies upon its intelligent use of memory and optimized data structures.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
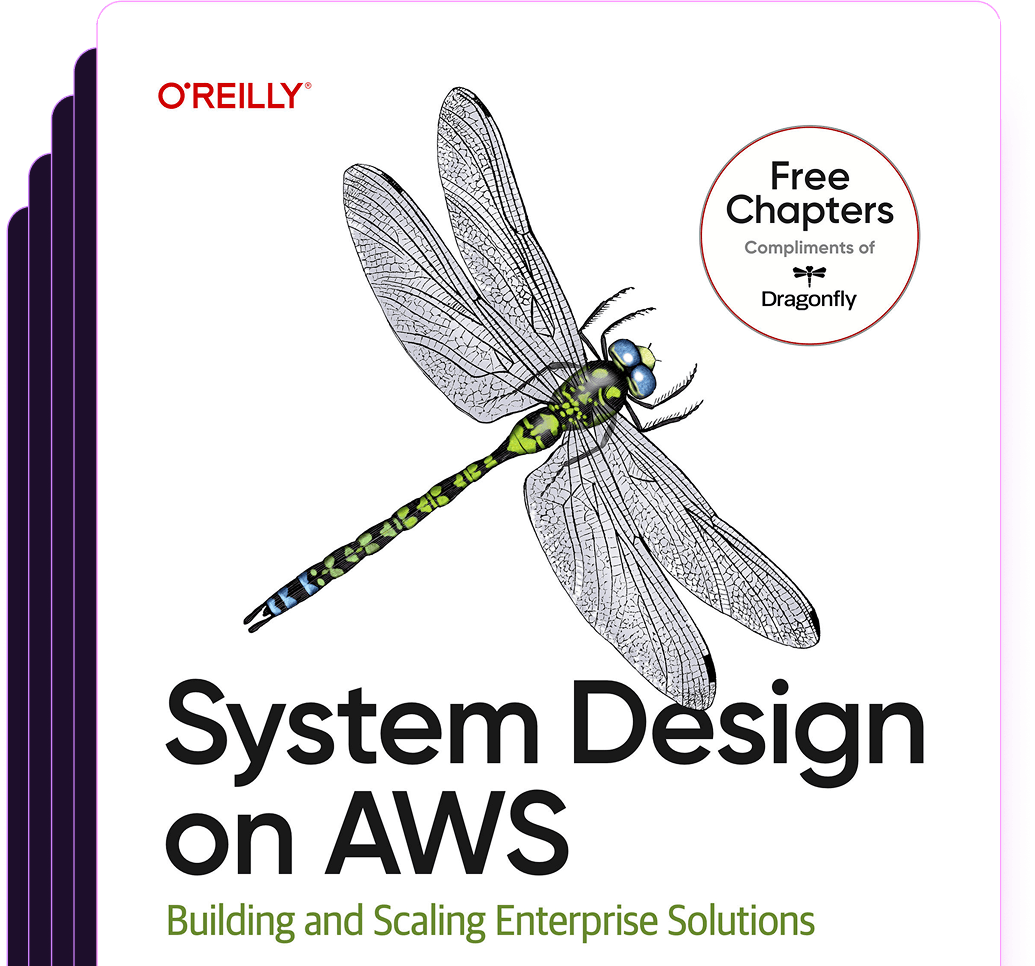
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost