Question: What are the differences between Memcached and in-memory caching?
Answer
Memcached and in-memory caching are both popular mechanisms for storing data in memory to boost performance. They serve similar purposes but have different features and use cases.
1. Memcached
Memcached is a distributed, high-performance, open-source memory caching system. It can help speed up dynamic web applications by alleviating database load.
Key Features:
- Distributed nature: Useful for horizontal scaling, as you can add more servers to expand your cache.
- Simple data model: Memcached only allows storage of key-value pairs.
- LRU eviction: When it runs out of memory, Memcached applies Least Recently Used (LRU) algorithm to remove older data.
Here's an example code snippet (Python) that shows how to work with memcached using the pymemcache library:
from pymemcache.client import base
client = base.Client(('localhost', 11211))
client.set('key', 'value')
result = client.get('key')
print(result)
2. In-Memory Caching
In-memory caching, on the other hand, is a broader term that refers to storing data in the memory of a single node (usually the application server). It’s not specific to any tool or system. Data stored in an in-memory cache is faster to read than from disk-based databases because it avoids disk I/O operations.
Key Features:
- Speed: Since the cache resides in the application's main memory, reading from and writing to it will be very fast.
- Simplicity: In-memory caches are often simpler to implement and manage.
However, in-memory caching generally lacks the distribution feature of Memcached and could lead to data loss if the application crashes. This is because the data in an in-memory cache isn't typically shared among multiple application instances.
Below is a simple Python in-memory cache example using a dictionary:
class Cache:
def __init__(self):
self.cache = {}
def get(self, key):
return self.cache.get(key)
def set(self, key, value):
self.cache[key] = value
cache = Cache()
cache.set('key', 'value')
print(cache.get('key'))
Comparison
When compared, Memcached stands out for its distributed nature, making it excellent for horizontal scaling. On the other hand, in-memory caching might be preferable for small applications where simplicity and speed are of primary importance, and there's no need for a distributed caching system. However, you should be aware of the risk of data loss if the application crashes or restarts.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- How can you implement a distributed cache using Docker?
- What are the differences between a centralized cache and a distributed cache?
- What is the best distributed cache for Java?
- What is the difference between distributed cache and local cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
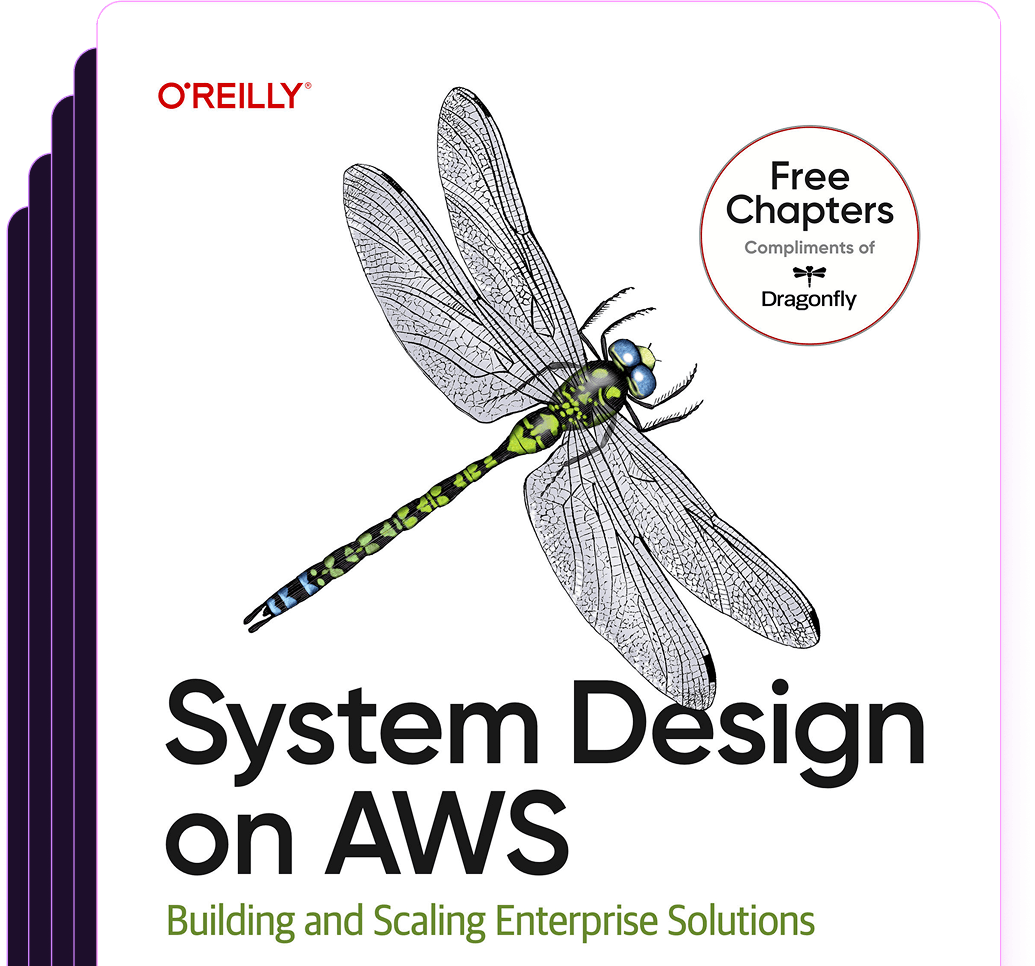
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost