Question: How to Connect to Redis in Kubernetes?
Answer
To connect to Redis in Kubernetes from your local machine, you need to set up a Redis deployment and service within your cluster, and then use port forwarding to access it. This can be achieved using Kubernetes manifests (YAML files). Following are the steps to create a simple Redis deployment and service, and then access it from your local machine.
- Create a Redis Deployment
Create a YAML file redis-deployment.yaml
with the following content:
```yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis
spec:
selector:
matchLabels:
app: redis
replicas: 1
template:
metadata:
labels:
app: redis
spec:
containers:
- name: redis
image: redis:latest
ports: - containerPort: 6379
```
- name: redis
- Apply the Deployment
Run the following command to apply the configuration and create the deployment:
```bash
kubectl apply -f redis-deployment.yaml
```
- Create a Redis Service
To expose Redis within the cluster, create a YAML file redis-service.yaml
with the following content:
```yaml
apiVersion: v1
kind: Service
metadata:
name: redis
spec:
selector:
app: redis
ports:
- protocol: TCP
port: 6379
targetPort: 6379
```
- protocol: TCP
- Apply the Service
Run the following command to apply the configuration and create the service:
```bash
kubectl apply -f redis-service.yaml
```
- Port Forwarding to Access Redis Locally
Use the following command to forward the port from your local machine to the Redis service running in the Kubernetes cluster:
```bash
kubectl port-forward svc/redis 6379:6379
```
This will make Redis accessible on localhost:6379
from your local machine.
- Access Redis from Your Local Machine
Now that Redis is accessible locally through port forwarding, you can connect to it using your preferred client. Here's an example using Python with the redis
library:
```python
import redis
# Connect to Redis
r = redis.Redis(host='localhost', port=6379)
# Set a key-value pair
r.set('my_key', 'Hello, Redis!')
# Get the value of the key
value = r.get('my_key')
print(value.decode('utf-8'))
```
By following these steps, you have successfully connected to Redis in Kubernetes from your local machine.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
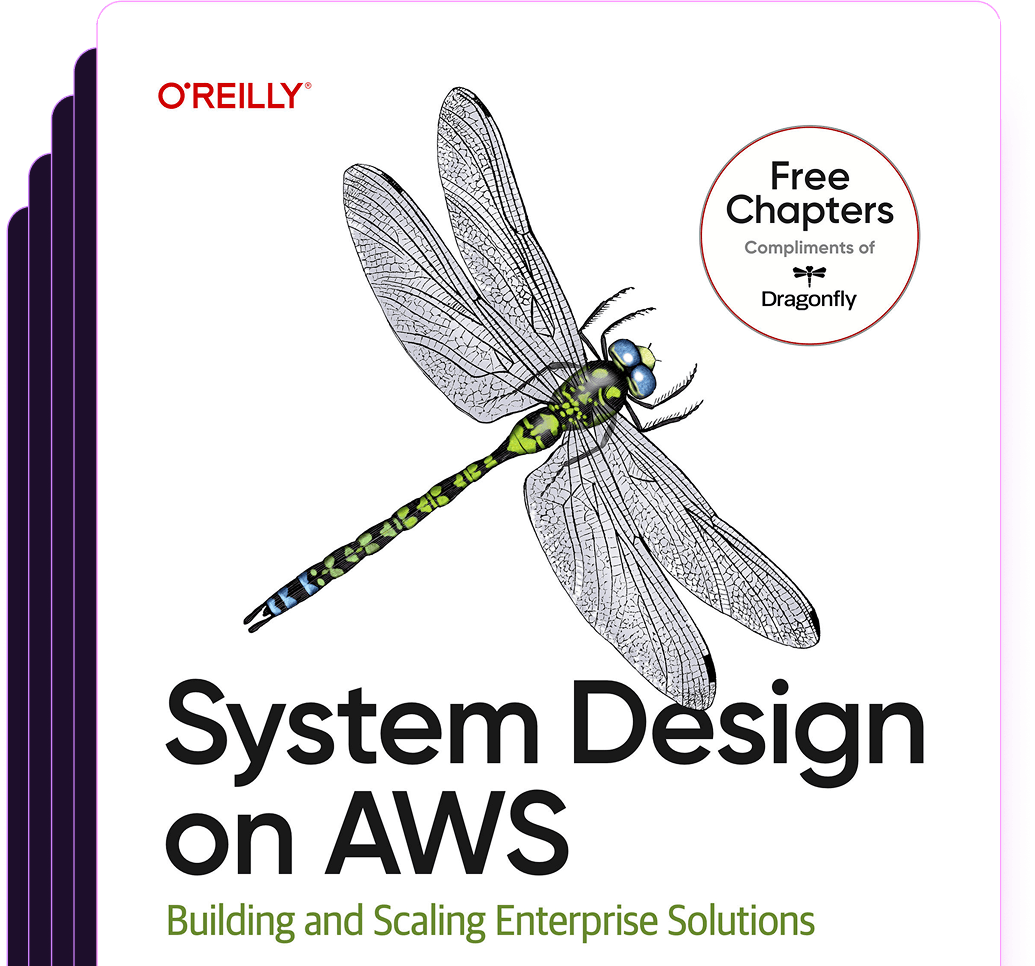
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost