Question: What is the difference between an in-memory cache and Redis?
Answer
In-memory caching and Redis are both solutions used to speed up applications by storing data in memory, but they have some key differences.
In-Memory Cache
In-Memory Caching refers to the general technique of storing data in the main memory (RAM) of a computing device to allow faster access compared to regular database or disk operations. This can be implemented in various forms including application-level caching (e.g., using arrays, lists, etc.), framework-provided caching mechanisms (e.g., Django's cache framework), or programming language-specific in-memory databases (like Python's shelve
).
The advantages include:
- Speed: As stated, accessing data from memory is faster than retrieving it from a disk.
- Simplicity: It can be straightforward to implement, especially for cache-friendly data structures like dictionaries or maps.
However, there are also drawbacks:
- Volatility: If your system crashes or restarts, all data stored in memory is lost without proper persistence mechanisms.
- Scalability: In-memory caches are harder to scale across multiple machines since they're typically local to the machine running the application.
# An example of in-memory caching in Python
cache = {}
def get_data(key):
if key not in cache:
# Fetch data from database or some other source
data = fetch_from_database(key)
cache[key] = data
return cache[key]
Redis
Redis (REmote DIctionary Server) is an open-source, in-memory data structure store that can be used as a database, cache, message broker, etc. Redis supports various kinds of abstract data structures, such as strings, lists, maps, sets, sorted sets, hyperloglogs, bitmaps, and spatial indexes.
Its advantages include:
- Persistence: Redis has mechanisms to persist data on disk periodically, which means you won't lose everything if your system crashes or restarts.
- Scalability and Replication: Redis provides features that enable you to easily scale up and out, with support for replication, partitioning and transactions.
- Data Structures: Unlike simple key-value stores, Redis has built-in support for more complex data types, making it versatile for a wide range of applications.
On the other hand, Redis can be overkill for very simple use cases, and requires additional resources to install, maintain and secure.
# An example of using Redis in Python
import redis
r = redis.Redis(host='localhost', port=6379)
# Setting a value in Redis
r.set('key', 'value')
# Retrieve a value from Redis
value = r.get('key')
In summary, while both in-memory caching and Redis serve similar purposes, Redis offers more advanced features at the cost of increased complexity. The choice between the two will depend on your specific needs and constraints.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
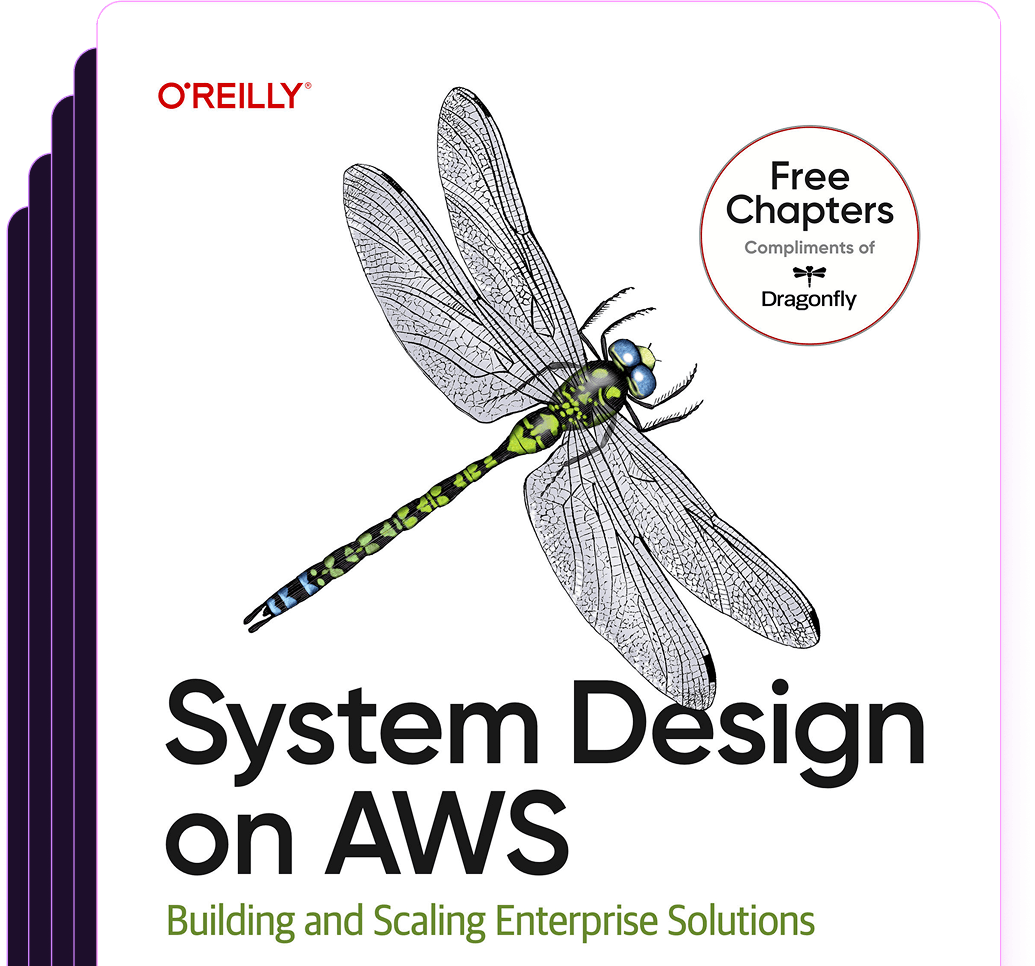
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost