Question: What are some major distributed caching solutions and how do they work?
Answer
Distributed caching is the method of storing a website or application’s data across a network of interconnected servers, promoting high availability, horizontal scaling, and fault tolerance. Here are some of the popular distributed caching solutions:
- Redis: Redis (Remote Dictionary Server) is an open-source in-memory database that can be used as a distributed cache. It supports various kinds of abstract data structures, such as strings, lists, maps, sets, and more.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('foo', 'bar')
value = r.get('foo')
- Memcached: Memcached is a high-performance distributed memory object caching system intended for use in speeding up dynamic web applications by alleviating database load. Memcached is very simple to install and use, and it's perfect for caching small and static data.
from pymemcache.client import base
client = base.Client(('localhost', 11211))
client.set('key', 'value')
result = client.get('key')
- Dragonfly: Dragonfly is a source-available, multi-threaded Redis and Memcached replacement built for modern cloud workloads. Since it's API is compatible with Redis and Memcached, the code example below (similar to Redis above) works with Dragonfly as well.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('foo', 'bar')
value = r.get('foo')
- Hazelcast: Hazelcast is a leading open-source in-memory data grid (IMDG). IMDGs are designed to provide high-availability and scalability by distributing data across multiple machines. Hazelcast also provides distributed implementations of many developer-friendly interfaces from Java such as Map, Queue, ExecutorService, Lock, and JCache.
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
IMap<Integer, String> map = hazelcastInstance.getMap("map");
map.put(1, "Value");
String value = map.get(1);
- Couchbase: Couchbase Server is an open-source, distributed multi-model NoSQL document-oriented database software package. It exposes a scale-out architecture with managed caching layers and persistence.
from couchbase.cluster import Cluster, QueryOptions
cluster = Cluster.connect("couchbase://localhost", "username", "password")
bucket = cluster.bucket("bucket-name")
coll = bucket.default_collection()
coll.upsert("key", {"name": "value"})
result = coll.get("key")
All these systems have their own benefits and trade-offs. Therefore, users need to make the choice based on their specific use case.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
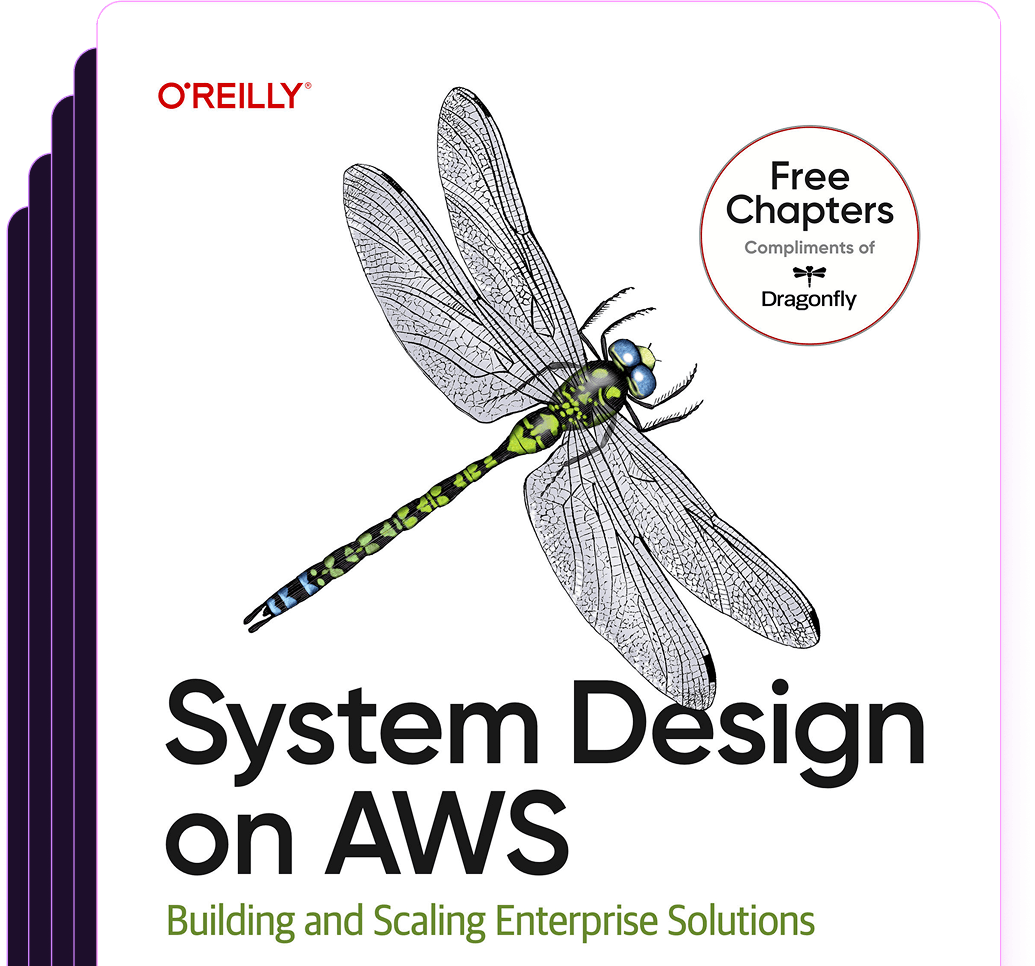
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost