Question: How can you use namespaces in a Redis cluster?
Answer
In Redis, the concept of "namespaces" doesn't exist as a built-in feature but is often implemented by developers using prefixes in key names. This approach helps to organize and segregate keys logically.
However, when working with a Redis cluster, it's important to understand that namespaces (implemented as key prefixes) won't affect the distribution of keys across the different nodes. Redis Cluster doesn't support multiple databases like the standalone version of Redis, which is often another way developers segregate data. It only provides database number 0.
Redis Cluster uses a hash slot system (a total of 16384 slots) to distribute data among the nodes. When a new key is created, Redis applies a CRC16 algorithm to the key name and then divides the result by 16384 to get the hash slot number. Therefore, even if two keys have the same prefix (or namespace), they may be stored in different nodes.
Here's an example of how you might implement namespaces:
import rediscluster
# Assuming you have a working Redis cluster setup at localhost ports 7000-7005
startup_nodes = [{"host": "127.0.0.1", "port": "7000"}, {"host": "127.0.0.1", "port": "7001"}]
rc = rediscluster.RedisCluster(startup_nodes=startup_nodes, decode_responses=True)
NAMESPACE = 'myNamespace:'
# Create some keys in your namespace
rc.set(NAMESPACE + 'key1', 'value1')
rc.set(NAMESPACE + 'key2', 'value2')
# Fetch them back
print(rc.get(NAMESPACE + 'key1')) # prints: value1
print(rc.get(NAMESPACE + 'key2')) # prints: value2
In this code, we're effectively creating a "namespace" by adding a prefix to our keys. The NAMESPACE
variable could be anything that makes sense for your application.
This is how namespaces can be used in the context of Redis. However, the actual storage of these keys will still be determined by the Cluster's hash slot system.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
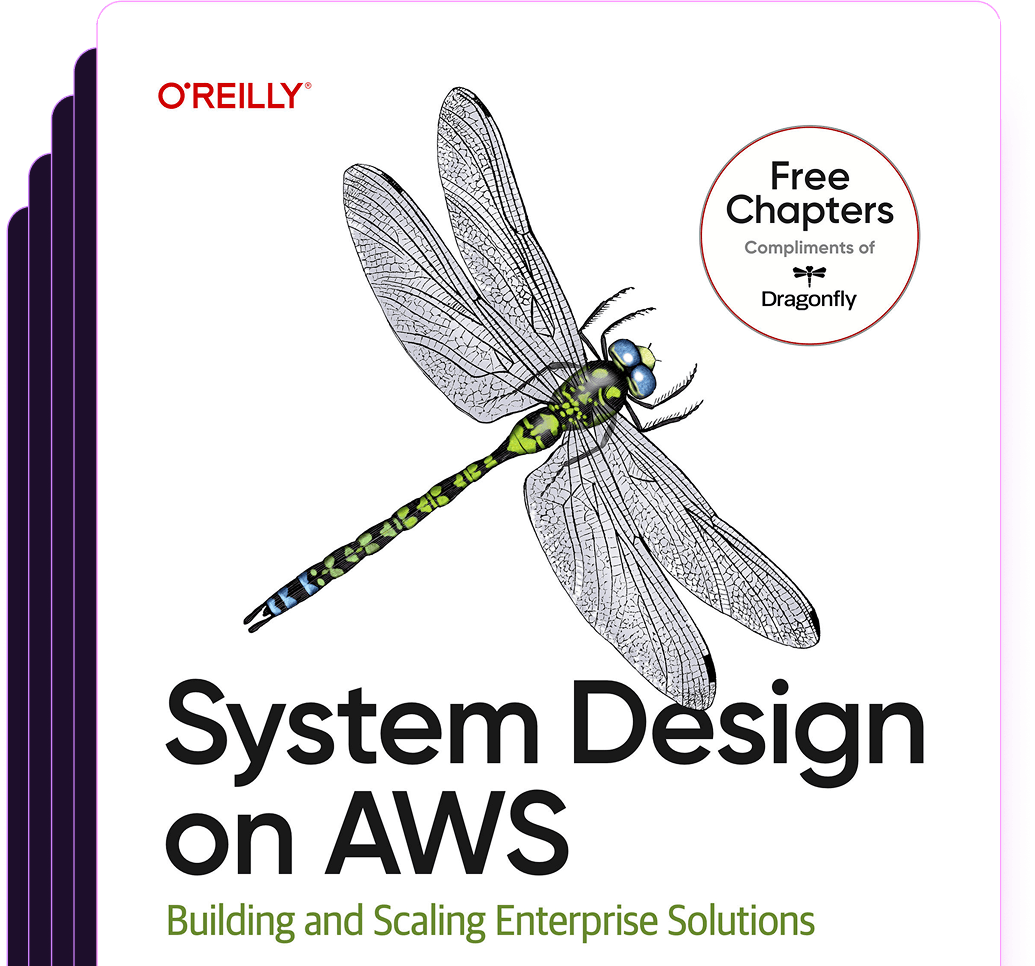
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost