Question: What is the performance of Redis MGET command?
Answer
The MGET
command in Redis is used to retrieve the values of all specified keys. It provides a noticeable improvement in performance when compared to issuing multiple GET
commands for each key, mainly because it reduces the latency costs associated with each command.
In terms of complexity, the MGET
command is O(N) where N is the number of keys being requested. This means that the time taken by the command scales linearly with the number of keys. Furthermore, since Redis operates single-threadedly, it's able to fetch and return all these keys in one round trip, avoiding the network overhead of making multiple individual GET
calls.
However, while MGET
is generally faster than multiple GET
commands, this assumes that the size of the data being fetched is not very large. If the total size of the keys being retrieved is very large, this could potentially block other clients' requests as Redis is single-threaded and handles one operation at a time.
Here's a small code example in Python using redis-py
library:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
keys = ['key1', 'key2', 'key3']
values = r.mget(keys)
print(values) # Output: ['value1', 'value2', 'value3']
In this example, MGET
retrieves the values of 'key1', 'key2', and 'key3' in a single operation which would be more efficient than doing three separate GET
operations.
In conclusion, MGET
can significantly improve performance by reducing network latency, especially when fetching multiple keys. However, be aware of the potential impact on Redis' single-threaded operation when fetching a large amount of data.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
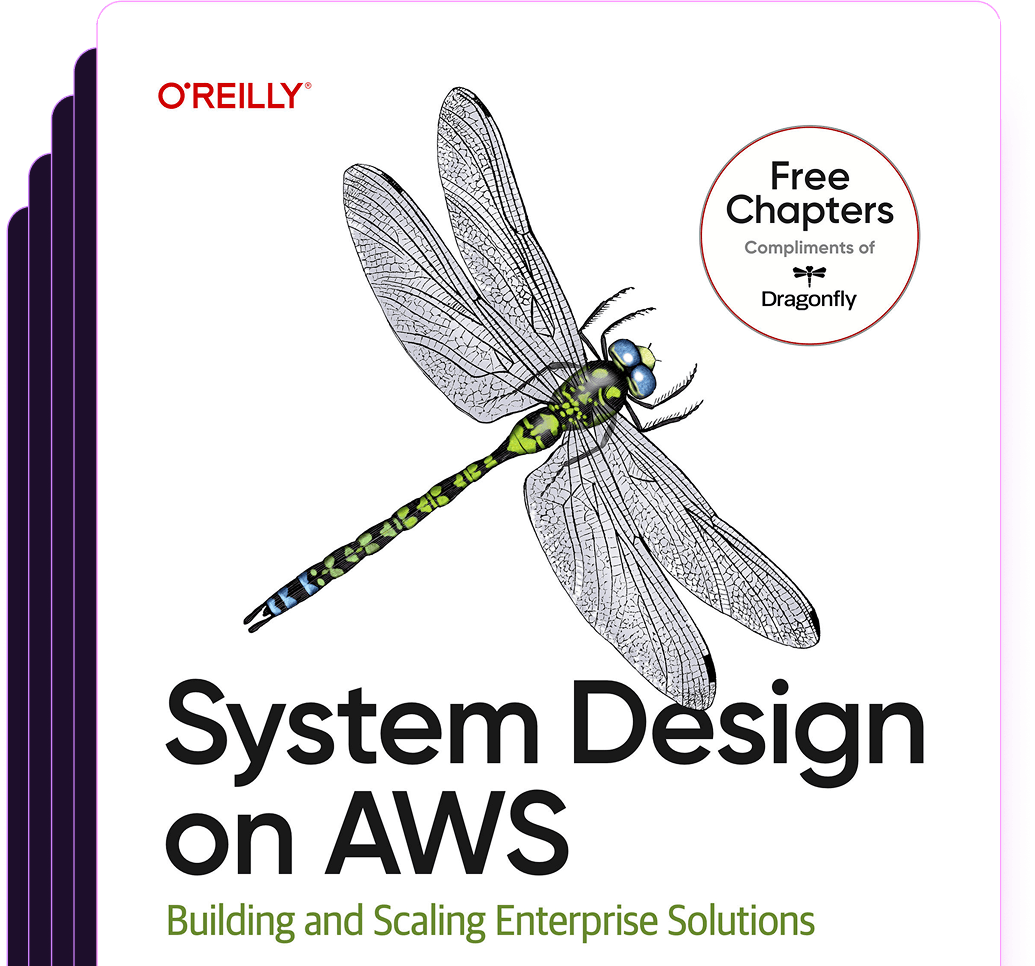
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost