Question: How can you scale WebSockets with Redis?
Answer
Redis can store session data, which allows different servers to share the state of WebSocket connections. This means if a user connects to Server A and then gets reconnected to Server B, Server B will be aware of the existing connection.
Additionally, Redis offers publish/subscribe capabilities, making it a good option for broadcasting messages to all connected clients, even if they're spread across multiple servers.
Here is a simple example demonstrating how to use Redis PUB/SUB with Node.js and WebSocket:
const WebSocket = require('ws');
const redis = require('redis');
// Create a new Redis client
const redisClient = redis.createClient();
// Create a WebSocket server
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
// Subscribe to the Redis channel on a new WebSocket connection
redisClient.subscribe('channel-name');
redisClient.on('message', (channel, message) => {
ws.send(message);
});
ws.on('message', (message) => {
// Publish the message from WebSocket to the Redis channel
redisClient.publish('channel-name', message);
});
});
In this code:
- When a new WebSocket connection is established, the server subscribes to a Redis channel.
- If a message is published on this Redis channel, every subscribed WebSocket client receives this message through Redis's
message
event. - When a WebSocket client sends a message, it's published to the Redis channel, reaching all other clients.
You can scale this setup by just starting more instances of this server. All instances are receiving and sending messages from/to the same Redis channel, allowing you to share WebSocket messages across different servers.
Remember that WebSockets might not be the best solution for every problem and it would be wise to consider your specific use case and evaluate if this approach fits your needs. Also note this example doesn't handle errors, disconnects or other edge cases, it's a simple demonstration of how Redis can help scale WebSockets.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
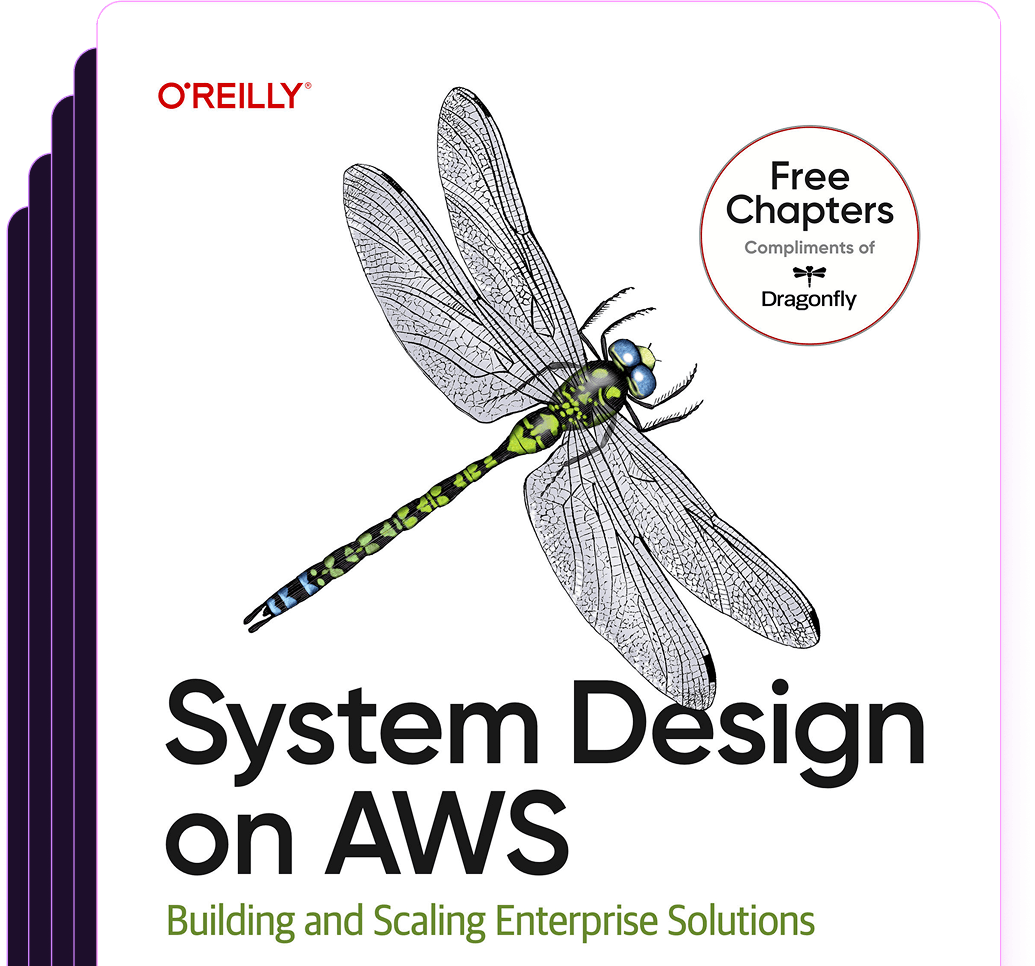
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost