Question: How to restart Redis?
Answer
To restart Redis, you can use one of the following methods depending on your Redis setup:
- Use Redis CLI
You can use the Redis command-line interface (CLI) to restart Redis.
First, connect to your Redis instance using the redis-cli
command:
```
redis-cli
```
Then, issue the SHUTDOWN
command:
```
SHUTDOWN
```
This will gracefully shut down Redis, saving any unsaved changes to disk and then restart it.
- Use Redis init script
If Redis was installed using a package manager on Linux, such as apt or yum, it likely came with an init script that you can use to start, stop, or restart Redis.
On Ubuntu, for example, you can use the following command to restart Redis:
```
sudo service redis-server restart
```
On CentOS/RHEL, you can use:
```
sudo systemctl restart redis
```
- Use Redis configuration file
You can also use the Redis configuration file to restart Redis. This method is useful if you want to change Redis settings before restarting it.
First, open the Redis configuration file in an editor:
```
sudo nano /etc/redis/redis.conf
```
Then, find the pidfile
parameter and note down its value. This parameter specifies the path to the Redis process ID file.
```
pidfile /var/run/redis/redis-server.pid
```
Now, kill the Redis process using the kill
command:
```
sudo kill $(cat /var/run/redis/redis-server.pid)
```
Finally, start Redis again:
```
sudo systemctl start redis
```
Make sure to replace the paths and commands above with the appropriate ones for your Redis setup.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
- Is Redis free?
- How to install Redis on Mac?
- How to check if Redis is running?
- Does Redis persist data?
- How to install Redis on Ubuntu?
- How to stop Redis server?
- How to see Redis version?
- How long does Redis store data?
- How is Redis so fast?
- How to connect to Redis server?
- Is Redis Multithreaded?
- Does Redis Use HTTP?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
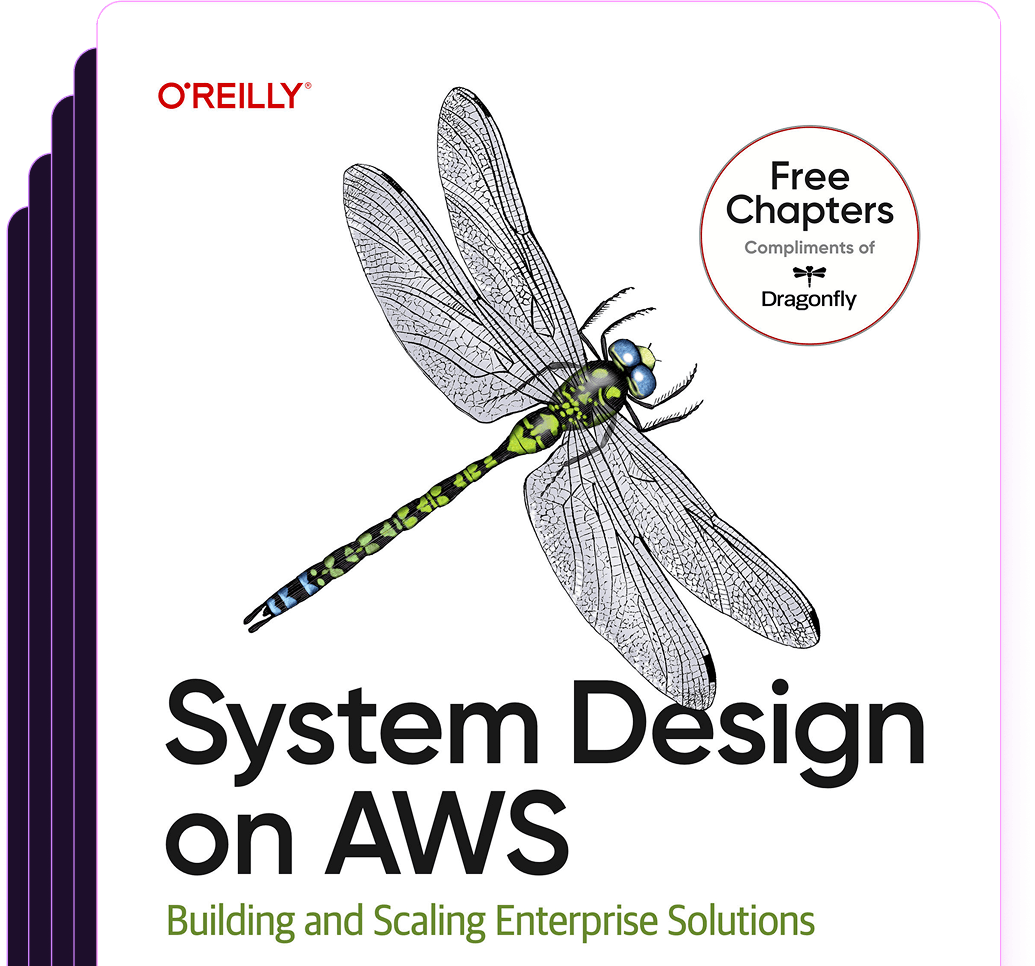
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost