Getting All Hash Keys Using Redis in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Commonly, Redis is used for caching and message passing, but its data structures make it useful for more complex use cases as well. One such instance is when you have a hash object in Redis, and you want to retrieve all keys from that hash, which can be useful when the keys are dynamic and not known beforehand.
Code Examples
In Python, with redis-py library, hashes in Redis can be interacted with much like standard Python dicts:
import redis
r = redis.Redis()
# Assuming you have a hash named 'myhash'
all_keys = r.hkeys('myhash')
print(all_keys)
This will output all the keys of the hash 'myhash'. Keep in mind that the keys are returned as bytes in Python 3, so you might need to decode them to get strings:
all_keys = [key.decode('utf-8') for key in r.hkeys('myhash')]
print(all_keys)
Best Practices
- It's common practice to handle Redis operations within exception blocks to manage any connection issues or errors while executing commands.
- When dealing with large amounts of data, keep an eye on memory usage and performance times. Redis operations are usually fast, but significant amounts of data could potentially slow down your applications.
Common Mistakes
- Not closing the connection after using it. It's important to close the connection when you're done with it to avoid maxing out connection limits.
- Using inappropriate data structures. Redis offers numerous data structures (like lists, sets, sorted sets etc.) in addition to hashes. Choosing the right one is critical for efficient data manipulation.
FAQs
- Are keys in Redis hash unique? Yes, much like a Python dictionary, keys in a Redis hash are unique.
- How do I delete a key from a Redis hash? You can use the
hdel()
function. For example:r.hdel('myhash', 'key_to_delete')
- Are Redis commands case sensitive? Yes, Redis commands are case sensitive. It's important to use the correct case when issuing commands.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Redis: Get Key Where Value in Python
- Python: Redis Get Keys Without TTL
- Getting Redis Replicas in Python
- Troubleshooting Wrong Type Error in Python Redis GET
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
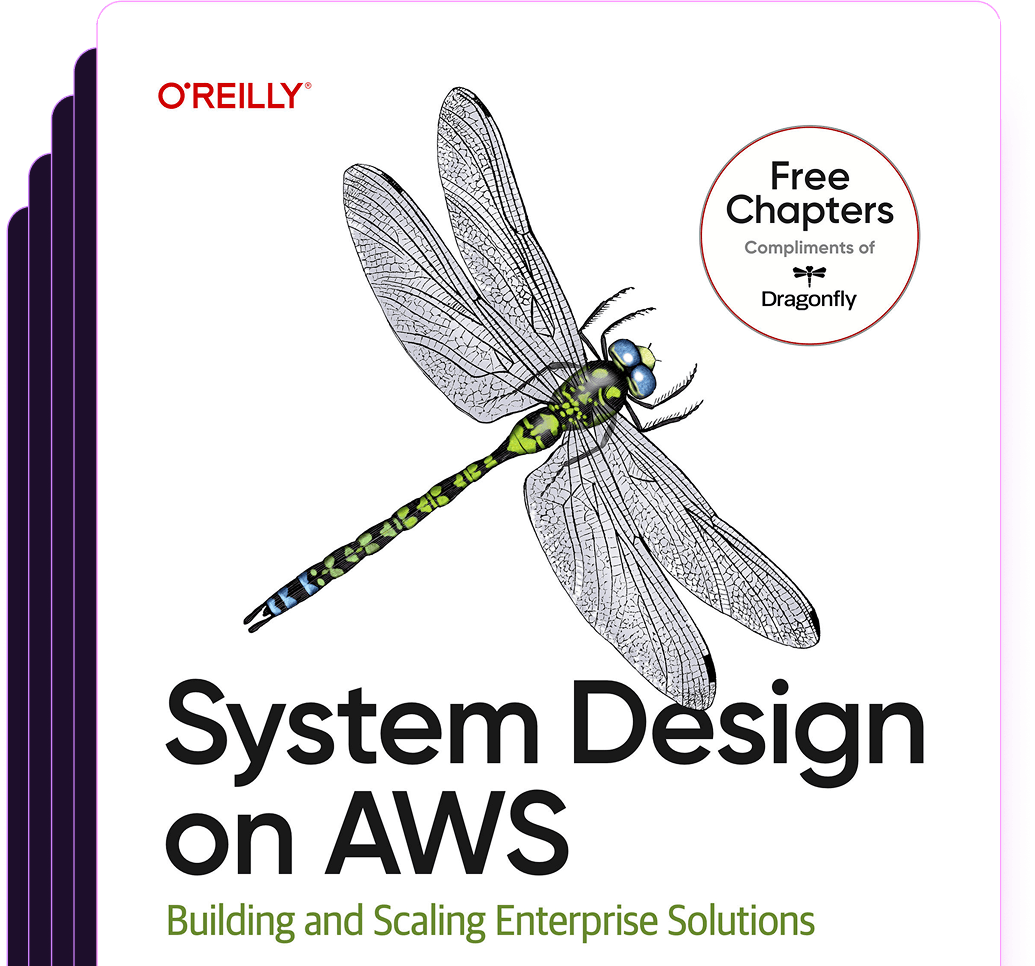
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost