Getting Memory Stats in Redis using Python (Detailed Guide w/ Code Examples)
Use Case(s)
Memory stats are crucial for monitoring and managing your Redis setup. They can provide insights into memory usage, fragmentation ratio, keyspace hits/misses, and other valuable indicators of performance or potential issues.
Code Examples
Example 1: Basic example to get memory stats. CODE_BLOCK_PLACEHOLDER_0
In this example, we first import the redis
module and then establish a connection to the Redis server. We use the info
method with the 'memory' argument to get memory information.
Example 2: Getting specific memory stats. CODE_BLOCK_PLACEHOLDER_1
This example is similar to the previous one, but we're extracting and printing specific memory statistics.
Best Practices
- Regularly monitor your memory usage to identify potential memory leaks or inefficient data structures.
- Clean up unused connections and keys to optimize memory use.
Common Mistakes
- Ignoring memory stats can lead to bloating and eventually crashing of your Redis instance.
- Not closing unused connections can leak memory over time.
FAQs
Q: How can I reduce memory usage in Redis? A: You can use memory-efficient data types, enable key expiry, and configure Redis for LRU eviction when memory is full.
Q: How often should I check memory stats? A: This depends on your application's needs. However, it's generally good practice to monitor them regularly for any unusual trends or spikes.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
- Querying Redis Eviction Policy in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
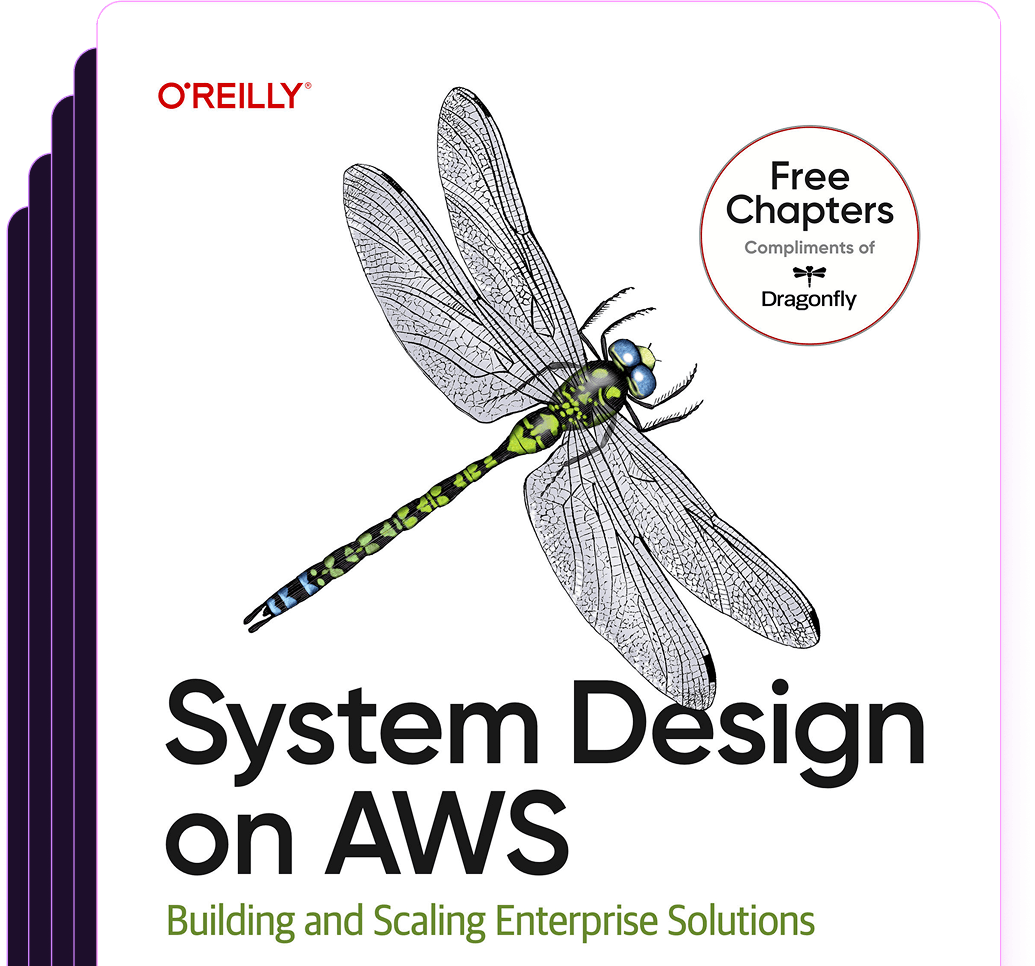
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost