Redis Get All Keys and Values in Python (Detailed Guide w/ Code Examples)
Use Case(s)
In Python, using Redis as a NoSQL database or caching system is common. The use case for getting all keys and values from Redis includes cache inspection or debugging, data migration, and bulk data processing.
Code Examples
For querying all keys and values from Redis in Python we use the redis
package. It's important to note that care should be taken while using these commands on large databases as they can consume significant resources.
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Get all keys
keys = r.keys()
# Get all values associated with the keys
for key in keys:
print('Key:', key)
print('Value:', r.get(key))
In this code, we first establish a connection to the Redis server running on localhost. Then we retrieve all keys from the database using the keys()
function. We loop through each key and retrieve its value using the get(key)
function.
Best Practices
When working with larger databases, it's best to avoid commands like KEYS in production environments because they might lead to performance issues. Instead, consider SCAN or similar commands which offer more control over the cursor and can limit the number of returned elements per call.
Common Mistakes
One common mistake is ignoring error handling while fetching keys. If the Redis server goes down or connection breaks during the operation, the application may crash. Always handle such potential errors to ensure your application's resilience.
FAQs
Q: What if a key does not exist in Redis? A: The get function will return None if the key does not exist in Redis.
Q: Can I get all keys matching a certain pattern? A: Yes, you can provide a pattern to the keys()
function, like r.keys(pattern='user:*')
to get all keys starting with 'user:'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
- Querying Redis Eviction Policy in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
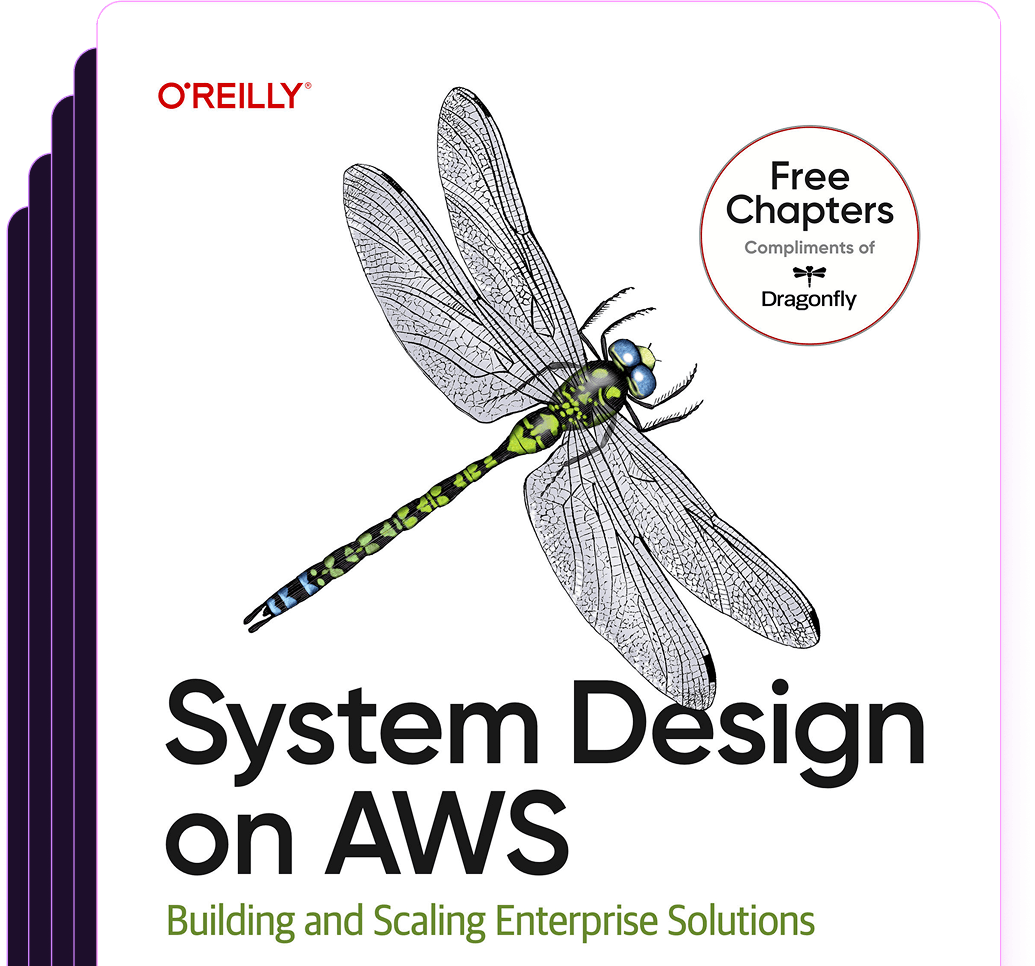
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost