Retrieving a Key by Value in Redis Using Python (Detailed Guide w/ Code Examples)
Use Case(s)
In Redis, data is stored as key-value pairs. However, Redis does not inherently support fetching a key by its associated value. This operation may be required when you have different keys with the same or similar values and you need to find those keys based on the value.
Code Examples
As Redis doesn't directly support this functionality, we need to create our own logic for this. Below is an example using python's redis
library:
import redis
db = redis.Redis(host='localhost', port=6379, db=0)
def get_keys_by_value(value):
keys = db.keys()
result_keys = [key for key in keys if db.get(key) == value]
return result_keys
print(get_keys_by_value('desired_value'))
In this example, we're first fetching all keys from the Redis database. Later, we iterate over each key, checking if it has the desired value. If it does, we add it to our result list. The final list contains all the keys that have the provided value.
Best Practices
- As getting a key by value in Redis requires scanning through every key-value pair, it can be a slow operation if the database is large. It's best to design your application in a way where direct key access is possible.
- Always try to use specific patterns while setting keys in Redis which can make the search process more efficient.
Common Mistakes
- Trying to get a key by value without considering the size of the database. This operation can be extremely slow on a large database and may have an impact on performance.
FAQs
- Can I get a key by value directly in Redis? No, Redis doesn't provide a built-in functionality to fetch a key by its value. You need to implement your own logic for this.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Redis: Get Key Where Value in Python
- Python: Redis Get Keys Without TTL
- Getting Redis Replicas in Python
- Troubleshooting Wrong Type Error in Python Redis GET
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
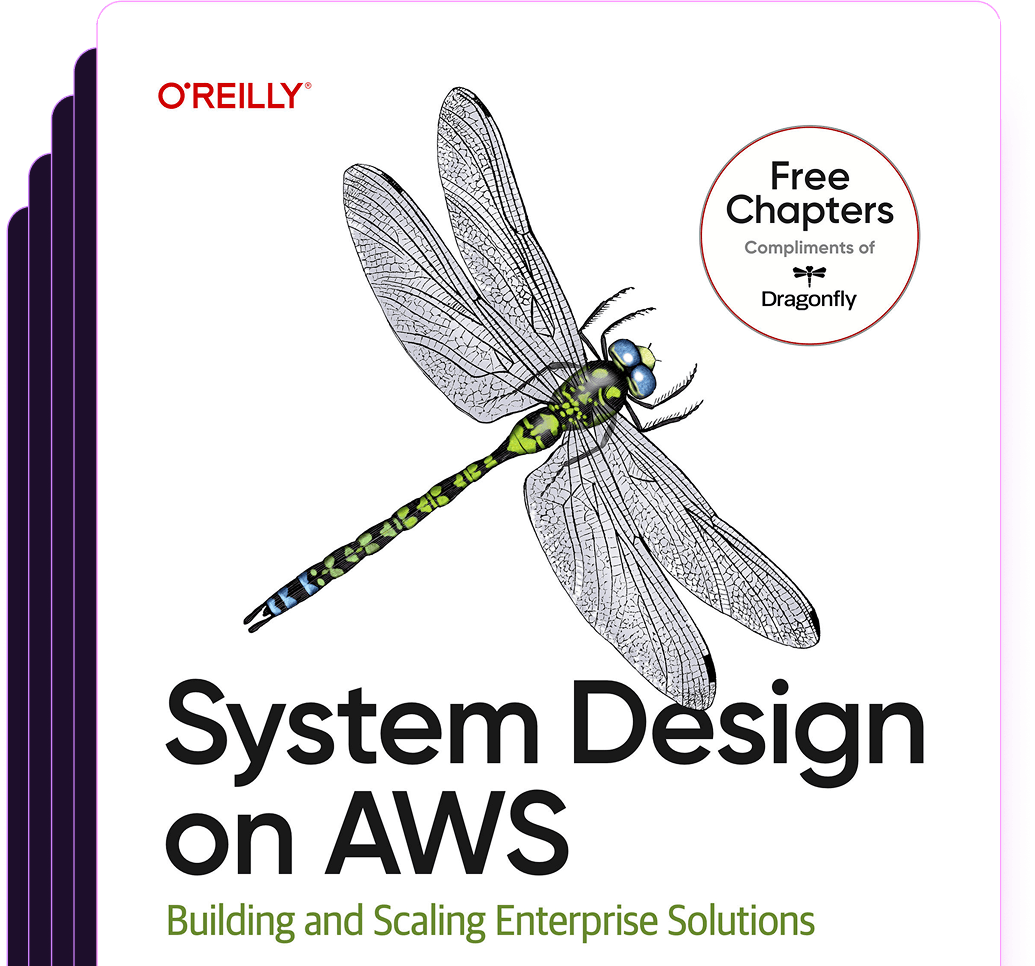
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost