Python: Redis Get Keys Without TTL (Detailed Guide w/ Code Examples)
Use Case(s)
Fetching keys from a Redis database without a set Time To Live (TTL) can be useful for understanding which data within your store may persist indefinitely. This could be helpful when debugging, or when trying to understand memory usage patterns.
Code Examples
In Python with the redis-py
library, you can get all keys without an associated TTL as follows:
import redis
# Establish connection
r = redis.Redis(host='localhost', port=6379, db=0)
# Get all keys in the database
all_keys = r.keys()
# Filter out those with any TTL value set
keys_without_ttl = [key for key in all_keys if r.ttl(key) == -1]
This script first fetches all keys in the Redis instance, then filters them by checking the TTL of each key. If ttl(key)
returns -1
, there is no expiry for that key.
Best Practices
When using the keys() function, be mindful that it can be slow on large databases, because it may block the server while retrieving the data. It's more efficient to use the SCAN command where possible.
Common Mistakes
A common mistake is to forget that the ttl
method returns -1
both when the key does not exist and when the key has no associated TTL. Make sure the key exists before interpreting -1
as 'no TTL set'.
FAQs
**Q: What does a TTL of -1 mean in Redis? ** A: In Redis, a TTL (or time-to-live) value of -1 means that the key does not have an expiration time.
**Q: Is there a way to set default TTL for all keys in Redis? ** A: While Redis itself doesn't provide a built-in way to set a default TTL for all keys, you can implement this logic in your application. Ensure you set a TTL every time you create a new key.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Redis: Get Key Where Value in Python
- Getting Redis Replicas in Python
- Troubleshooting Wrong Type Error in Python Redis GET
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
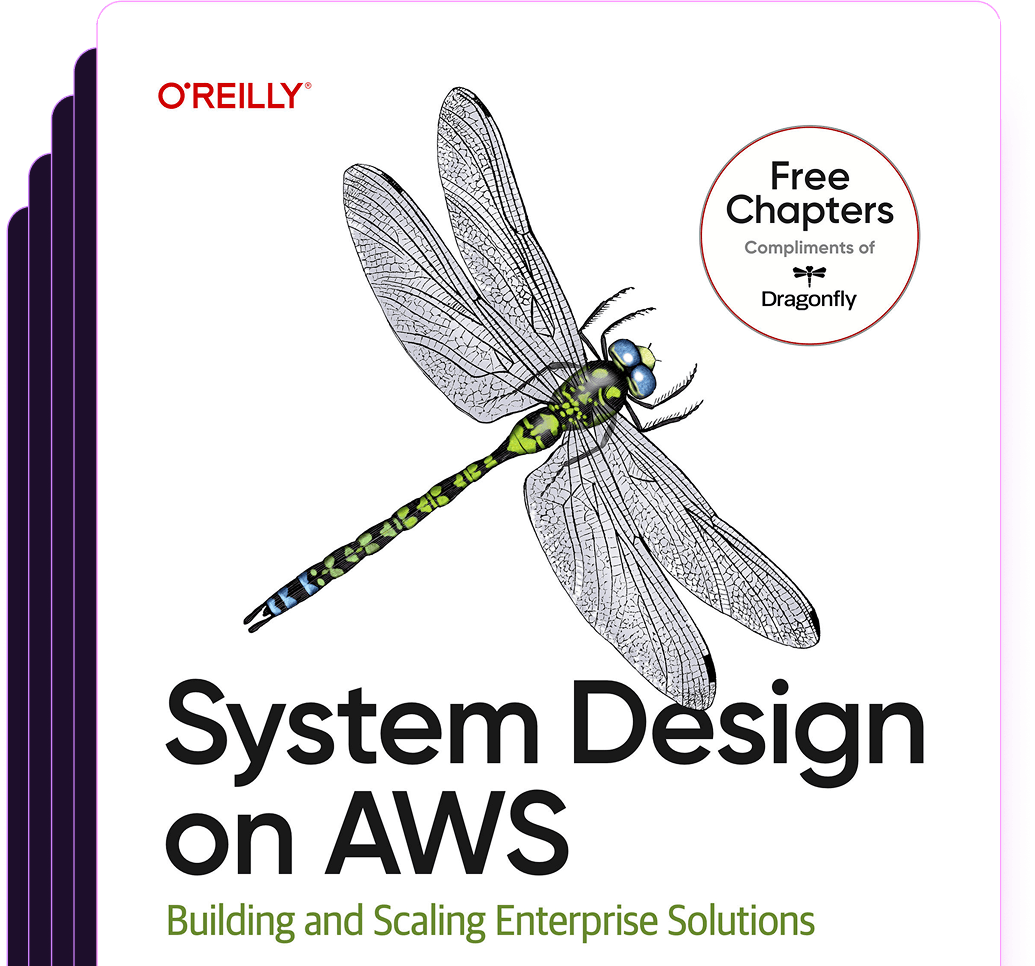
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost