Redis HMSET in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
HMSET is a Redis command used for inserting multiple field-value pairs into a hash stored at a key. Common use cases include storing objects with many fields or attributes, such as user profiles where each field represents an attribute of the user like name, age, email, etc.
Code Examples
Let's consider we are using "go-redis" library, which is a popular Redis client for Golang. To install it, you can use go get
:
go get github.com/go-redis/redis
Now let's see an example of how to use HMSET in Go.
package main
import (
"github.com/go-redis/redis"
"fmt"
)
func main() {
client := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "", // no password set
DB: 0, // use default DB
})
// Set multiple fields in a hash
err := client.HMSet("user_profile", map[string]interface{}{
"name": "John Doe",
"age": 30,
"email": "john.doe@example.com",
}).Err()
if err != nil {
panic(err)
}
fmt.Println("User profile set successfully")
}
In this example, we're creating a new Redis client, connecting to a local Redis instance. Then we're using the HMSet method of the client to set multiple fields (name, age, email) in a hash identified by the key "user_profile".
Best Practices
- It's best to handle potential errors from the HMSet operation to ensure data integrity.
- If a large number of fields need to be updated, use HMSET instead of multiple HSET commands for performance optimization.
Common Mistakes
- Not checking or handling errors from HMSet. If there's an issue with the Redis server or network, you'll want to know about it and handle it appropriately.
- Using wrong types for field values. As Redis can only store strings, Golang types are converted to strings before storing. When retrieving values, remember to convert them back to needed types.
FAQs
Q: Does HMSET overwrite existing keys? A: Yes, if you use HMSET with a key that already exists, it will overwrite the previous value with the new one.
Q: What happens if the field already exists in the hash? A: If the field already exists in the hash, HMSET will update the field with the new value.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
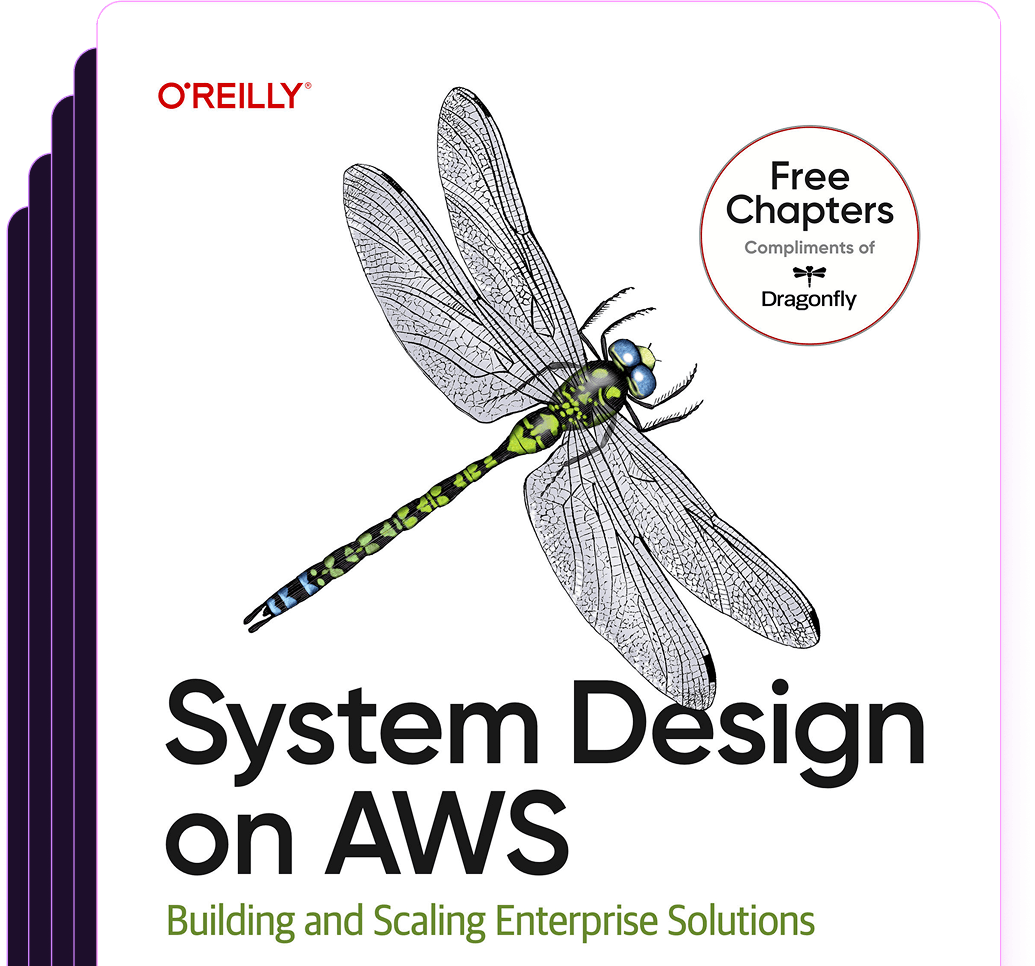
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost