Redis HSET in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
Redis' HSET
command is used to set a field in a hash to a specific value. Common use cases include:
- Storing object-like items, where each object's properties and values are represented by the field-value pairs in the hash.
- Caching data that needs to be quickly retrieved using a key.
Code Examples
Let's consider we're using the popular go-redis
library.
Example 1: Set a field in a hash.
package main
import (
"github.com/go-redis/redis"
"fmt"
)
func main() {
client := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
err := client.HSet("user", "name", "John").Err()
if err != nil {
panic(err)
}
result, err := client.HGet("user", "name").Result()
if err == redis.Nil {
fmt.Println("No name found")
} else if err != nil {
panic(err)
} else {
fmt.Println("Name:", result)
}
}
In this example, we create a new client connection to a local Redis server. We then set the "name" field of the "user" hash to "John". Finally, we retrieve the "name" we just set and print it out.
Best Practices
- Always handle errors from
HSET
. Ignoring errors can lead to hard-to-diagnose issues later on. - Reuse Redis clients as much as possible rather than creating a new one for each operation. It's more efficient in terms of resources.
Common Mistakes
- A common mistake is to assume that
HSET
will return an error if the field already exists in the hash.HSET
will simply overwrite any existing field with the new value.
FAQs
Q: Can I set multiple fields at once?
A: Yes, use the HMSet
function to set multiple field-value pairs at once.
err := client.HMSet("user", map[string]interface{}{
"name": "John",
"age": 30,
}).Err()
This sets both the "name" and "age" fields of the "user" hash.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
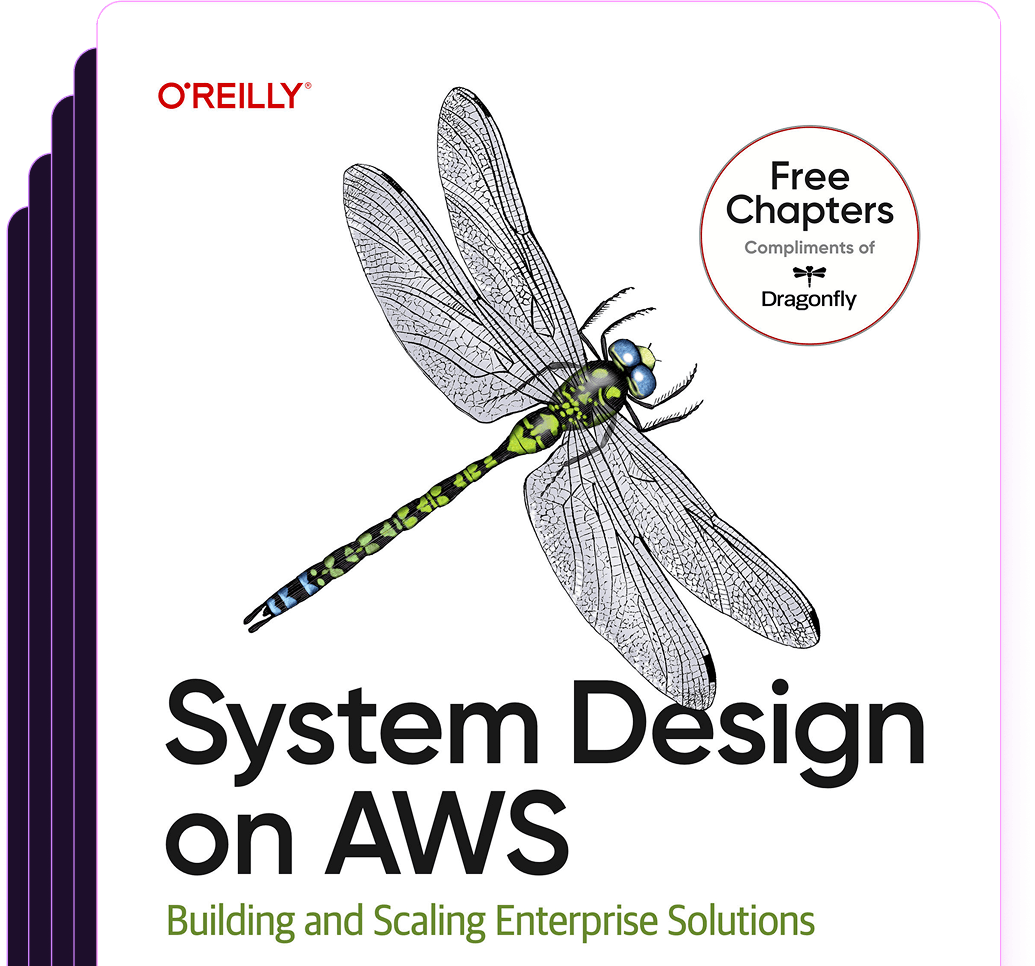
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost