Redis HGET in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
The HGET
command in Redis is used to retrieve the value associated with a specific field from a hash stored at a key. In Golang, you might use this while implementing caching, session management, or any feature where you need to fetch particular data related to an object.
Code Examples
Example 1: Basic Usage of HGET
In this example, we connect to a local Redis instance and retrieve a field's value from a hash. CODE_BLOCK_PLACEHOLDER_0
Here, we first set the "name" field for the hash at "user:1000" to be "John Doe". We then use HGet
to retrieve the name field, which prints out "John Doe".
Best Practices
- Error Handling: Always handle errors returned by
HGet
. This will prevent your application from panicking in case the Redis server is not accessible or some other error occurs. - Connection Management: Create a single Redis client for your application and reuse it. Don't create a new client for each query.
Common Mistakes
- Ignoring Error Returns:
HGet
returns two values - the result and an error. Ignoring the error return value is a common mistake which can lead to unexpected results. - Misunderstanding Nil Return: If no field or key exists,
HGet
will return a nil error which can be mistaken for a connection or server problem. Ensure to check if the key or field exists before attempting to useHGet
.
FAQs
Q: What happens when the key or field does not exist? A: The HGET
command will return a nil response without an error. This could sometimes be misconstrued as an error in connection or server. Always validate that your keys and fields exist before running the command.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
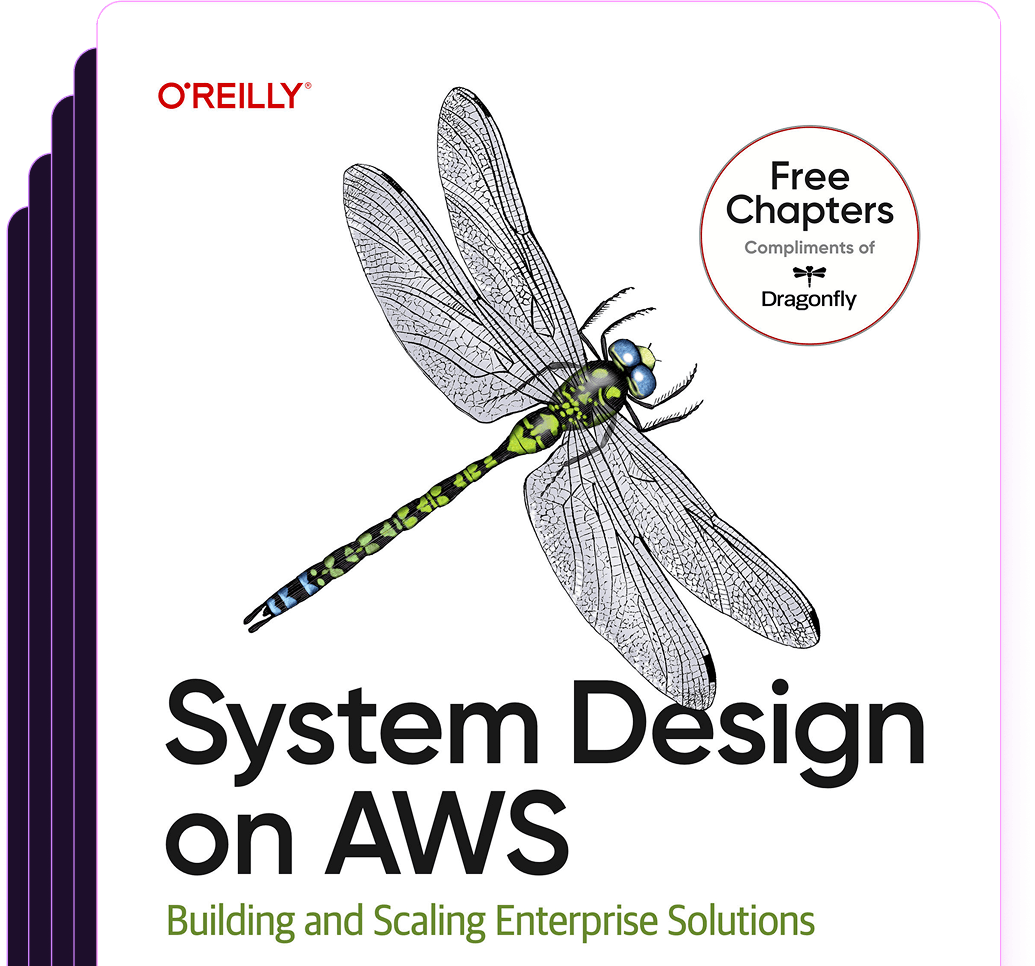
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost