Redis HGETALL in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
The HGETALL
command in Redis is used to retrieve all the fields and their corresponding values of a hash stored at a specific key. Common use cases include:
- Fetching user data by user ID, where each field-value pair represents a different aspect of user information.
- Obtaining entire datasets for further processing or analysis.
Code Examples
In Golang, you can interact with Redis using the go-redis
package. Below are examples of how to use the HGETALL
command:
package main
import (
"fmt"
"github.com/go-redis/redis/v8"
"golang.org/x/net/context"
)
var ctx = context.Background()
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
err := rdb.HSet(ctx, "user:1001", "name", "John", "age", "30").Err()
if err != nil {
panic(err)
}
val, err := rdb.HGetAll(ctx, "user:1001").Result()
if err != nil {
panic(err)
}
fmt.Println("user:1001", val)
}
In this example, we first create a hash with the HSET
command. The hash is at key user:1001
and has two fields, name
and age
, with corresponding values John
and 30
. We then retrieve all fields and their values using the HGETALL
command.
Best Practices
- Make sure that the key exists before calling
HGETALL
. If the key does not exist, Redis will return an empty list or set. - Keep hash fields and values small to conserve memory.
Common Mistakes
- Misunderstanding that
HGETALL
retrieves all keys in a Redis instance. It actually retrieves all fields and their values for a given hash key. - Using
HGETALL
on large hash instances can lead to blocking operations and impact performance. Consider usingHSCAN
if you only need part of the fields.
FAQs
Q: What happens if the key does not exist in Redis when I call HGETALL? A: Redis will return an empty list or set.
Q: What is the time complexity of the HGETALL command in Redis? A: The time complexity is O(N) where N is the size of the hash.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
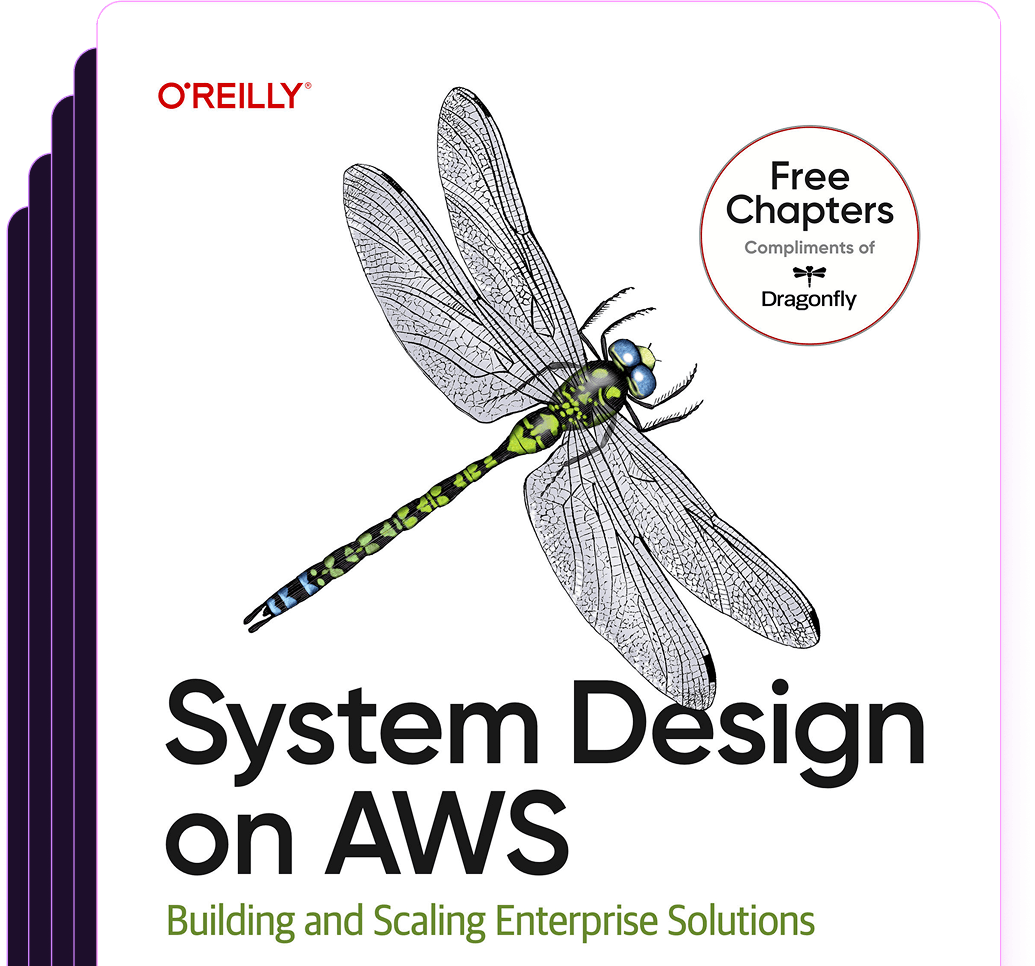
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost