Redis HEXISTS in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
The HEXISTS
command in Redis is used to determine if a hash field exists. This can be particularly useful in scenarios where you need to check the existence of a specific key within a hash before performing certain operations, such as updates or deletions, where these operations are only meaningful if the key exists.
Code Examples
Firstly, ensure that you have imported the necessary redis package for Go:
import "github.com/go-redis/redis/v8"
Here is an example of how to use HEXISTS
in Golang:
package main
import (
"context"
"fmt"
"github.com/go-redis/redis/v8"
)
var ctx = context.Background()
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
err := rdb.HSet(ctx, "myhash", "field1", "value1").Err()
if err != nil {
panic(err)
}
exists, err := rdb.HExists(ctx, "myhash", "field1").Result()
if err != nil {
panic(err)
}
fmt.Println(exists) // Output: true
}
In this code, we first set a field named "field1" with the value "value1" in a hash "myhash". Then we check if "field1" exists in "myhash" using HEXISTS
. If it exists, it will return true
, otherwise it will return false
.
Best Practices
- Always check for errors when using the
HEXISTS
command in order to handle any issues that may occur during its execution. - Use context appropriately for controlling timeouts.
Common Mistakes
- Neglecting to check if the key exists before performing operations can lead to unexpected behavior and potential data inconsistencies.
FAQs
Q: What if the hash does not exist? A: The HEXISTS
command will return false
even if the hash itself does not exist.
Q: What happens if the field does not exist in the hash? A: In this situation, HEXISTS
will also return false
.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
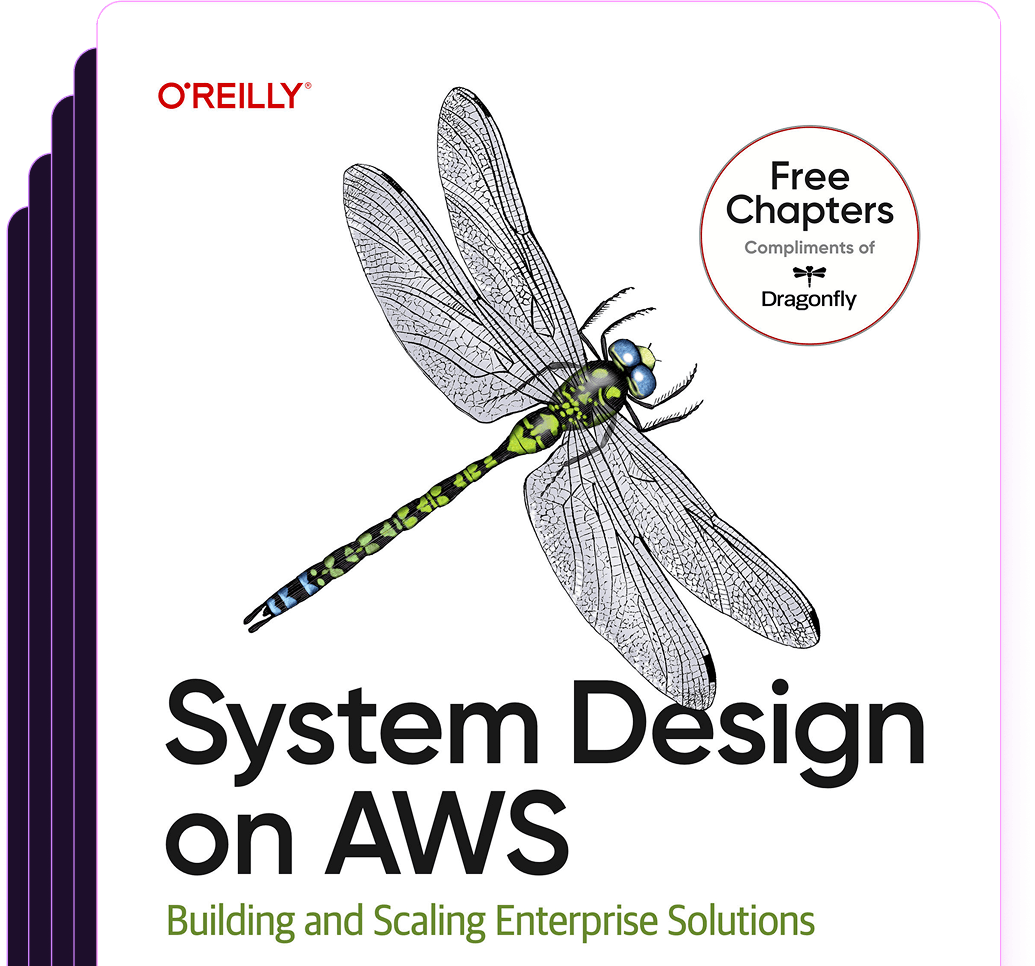
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost