Redis HMGET in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
The HMGET
command in Redis is used to retrieve the value of one or more fields stored in a hash. Common use cases for this command include:
- Fetching multiple values at once from a hash in a Redis database.
- Analyzing data stored as key-value pairs in a hash.
Code Examples
To interact with Redis in Go, we'll use the go-redis
package. This can be installed using go get github.com/go-redis/redis
.
Here's an example of setting and getting multiple fields using HMSET
and HMGET
.
package main
import (
"fmt"
"github.com/go-redis/redis"
)
func main() {
client := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "", // no password set
DB: 0, // use default DB
})
err := client.HMSet("hashkey", map[string]interface{}{
"field1": "value1",
"field2": "value2",
}).Err()
if err != nil {
panic(err)
}
vals, err := client.HMGet("hashkey", "field1", "field2").Result()
if err != nil {
panic(err)
}
for _, v := range vals {
fmt.Println(v)
}
}
In this example, we first create a new Redis client connected to localhost on port 6379. We then set two fields ("field1" and "field2") in the hash at "hashkey" using the HMSet
function. Later, we retrieve the values of "field1" and "field2" using the HMGet
function.
Best Practices
- Always handle errors returned by
HMGet
, don't ignore them. - Use
HMGet
when you need to fetch multiple fields from a hash at once as it's more efficient than callingHGet
multiple times.
Common Mistakes
- Not checking if the key actually contains a hash before calling
HMGet
. If the key does not exist or is associated with a non-hash data type, an error will be returned. - Not handling the case when a field does not exist in the hash. If this happens,
HMGet
will return anil
value for that field.
FAQs
Q: What happens if the hash or the fields do not exist? A: If the hash does not exist, HMGet
will return an empty list. If a field does not exist, HMGet
will include a nil
value in its output for that field.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
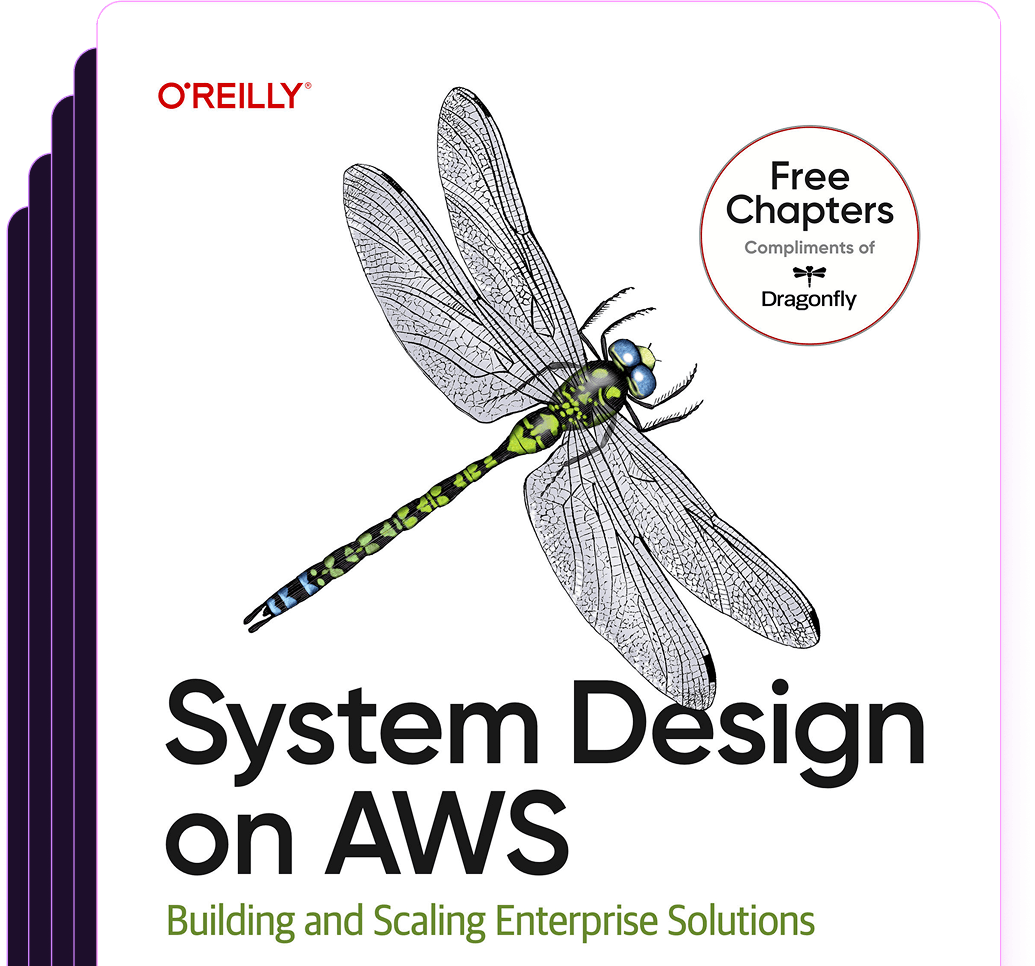
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost