Redis Get All Keys in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Often, while using Redis as a cache or transient data store in Java applications, developers need to retrieve all keys stored in the database. This can be useful for administrative tasks, debugging purposes, or certain specific functional requirements.
Code Examples
Let's use Jedis, a popular Java Redis client, to get all keys from Redis in Java. You can add Jedis to your project using Maven or Gradle.
Example 1: Getting all keys in Redis
```
import redis.clients.jedis.Jedis;
Jedis jedis = new Jedis("localhost");
Set<String> keys = jedis.keys("*");
keys.forEach(System.out::println);
```
This code creates a Jedis instance connected to Redis running on localhost. The keys("*")
command retrieves all keys in the current database. The keys are then printed to the console.
Best Practices
- The
KEYS
command should be used judiciously in production environments because it can negatively impact performance, especially when dealing with large databases. - Instead, consider using
SCAN
command which provides a cursor-based iterator over the collection of keys, which is more efficient and better suited for production.
Common Mistakes
- A common mistake is not handling the potential
JedisConnectionException
. Always put your Redis operations within a try-catch block to handle potential connectivity issues.
FAQs
Q: Can I filter the keys that I retrieve?
A: Yes, you can replace the "*" in the keys()
function with any pattern following Redis glob-style patterns.
Q: Can I use this method to get all keys in a Redis cluster?
A: No, the keys()
function only retrieves keys from the currently selected database in the current Redis instance. For a cluster, you would need to connect and retrieve keys from each node separately.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
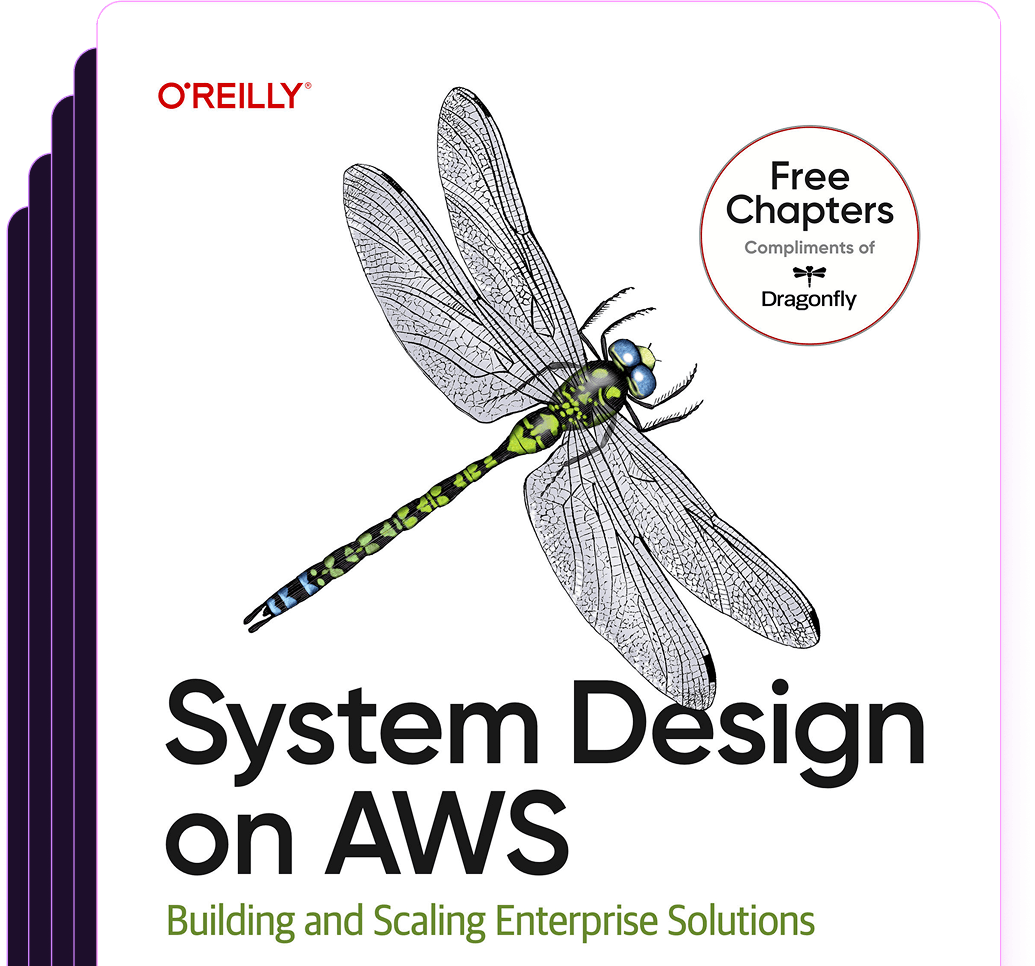
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost