Getting a List from Redis in Java (Detailed Guide w/ Code Examples)
Use Case(s)
In Java applications, Redis is often used as an in-memory database to store and retrieve data structures like lists. Common use cases include storing user sessions, leaderboards, timelines, etc.
Code Examples
Here are examples of how you can get a list from Redis using Java:
Example 1:
Jedis jedis = new Jedis("localhost");
List<String> list = jedis.lrange("mylist", 0 ,5);
for(String item: list){
System.out.println(item);
}
In this example, we're connecting to a local Redis instance, retrieving the first five items from 'mylist', and then printing them out.
Example 2:
Jedis jedis = new Jedis("localhost");
String key = "mylist";
Long length = jedis.llen(key);
List<String> list = jedis.lrange(key, 0 ,length-1);
for(String item: list){
System.out.println(item);
}
In this second example, we're getting the total length of the list first, and then retrieve the entire list.
Best Practices
When working with Redis in Java, always ensure to close the connection when it's no longer needed to free up resources. Also, use exception handling for better error control.
Common Mistakes
A common mistake is not checking if the key exists before trying to retrieve the list. Always check for key existence before any operations.
FAQs
Q: Can I get a specific range of elements from the list?
A: Yes, you can specify the start and end index in the lrange
method.
Q: How can I handle exceptions when retrieving a list from Redis?
A: You can use try-catch blocks to handle any exceptions that may occur during the process.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
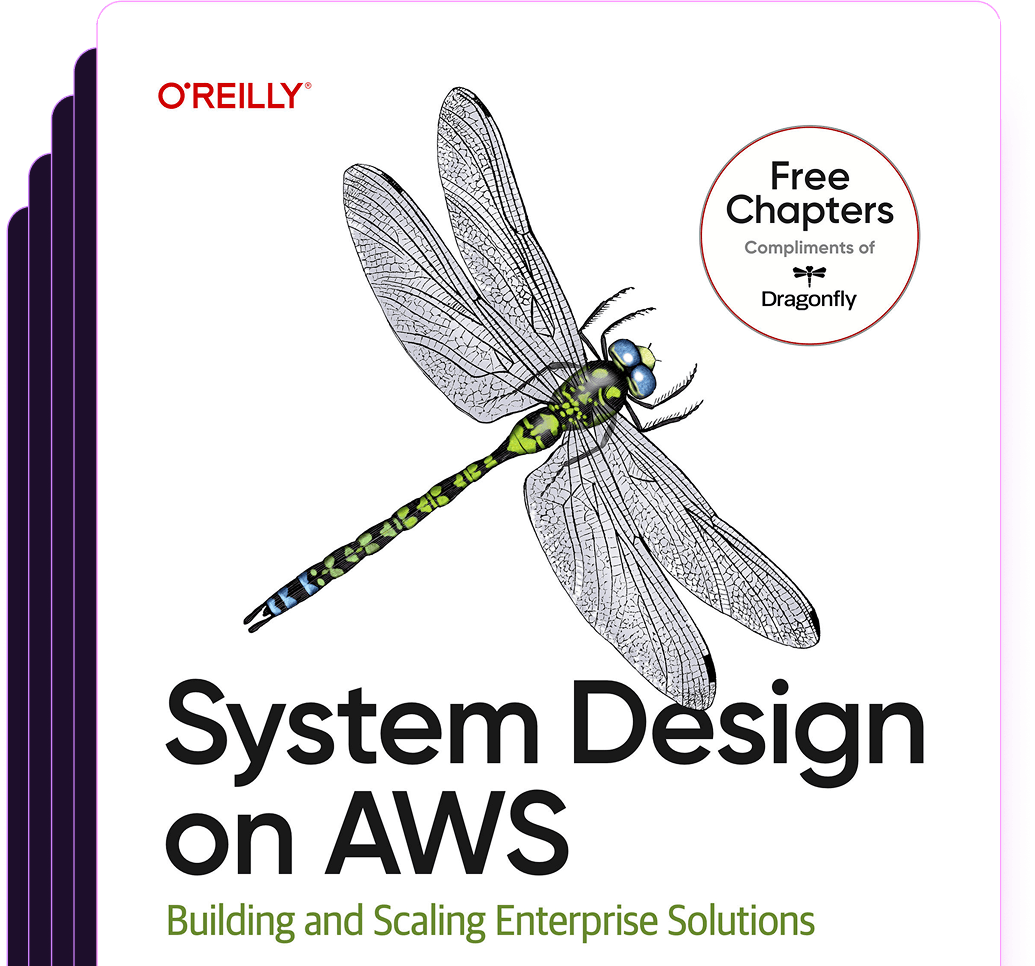
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost