Getting Hash Values at Key in Redis Using Java (Detailed Guide w/ Code Examples)
Use Case(s)
Hashes in Redis are perfect for representing objects. A common use case is when you need to retrieve all field-value pairs (hash values) stored in a hash at a given key.
Code Examples
We will be using Jedis
, a popular Redis client for Java. Let's suppose we have a hash stored at key 'user:1' and want to fetch all fields and their corresponding values.
import redis.clients.jedis.Jedis;
Jedis jedis = new Jedis("localhost");
Map<String, String> userFields = jedis.hgetAll("user:1");
for( Map.Entry<String, String> entry : userFields.entrySet()) {
System.out.println("Field: " + entry.getKey() + ", Value: " + entry.getValue());
}
In this example, hgetAll()
method is used to get all fields and corresponding values of hash stored at 'user:1'.
Best Practices
- It’s important to handle exceptions for network failures or if the key doesn't exist.
- Always close the connection (
jedis.close()
) when you're done to prevent resource leaks.
Common Mistakes
- Not checking if the key exists before trying to get values. You can use
jedis.exists(key)
to confirm. - Trying to get fields from a key that does not store a hash will result in an error. Confirm the type of data structure stored at a particular key using
jedis.type(key)
.
FAQs
- What happens if the key does not exist in Redis? If the key does not exist,
hgetAll()
will return an empty map. - Can I use this method to get values from data structures other than hashes in Redis? No,
hgetAll()
is specifically meant for hashes. Attempting to use it on other data structures will result in an error.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
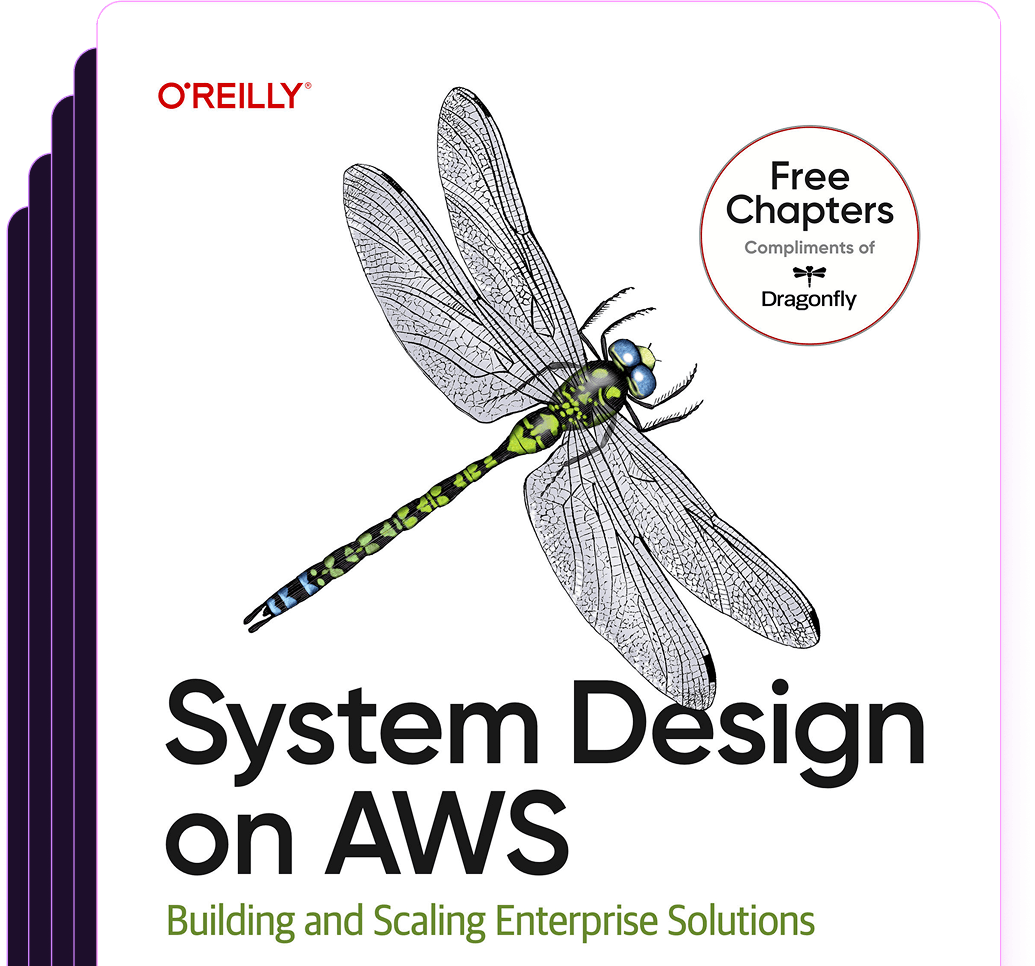
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost