Retrieving a Key from Redis in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Redis, being a powerful key-value store, has various use cases. One fundamental use case is retrieving a value from Redis using a known key. This operation is commonly performed when you want to fetch data that was previously stored in Redis.
Code Examples
Let's suppose we are using Jedis, a popular Redis client for Java.
- Retrieving a single value:
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost");
String key = "myKey";
String value = jedis.get(key);
System.out.println("Value: " + value);
}
}
In this example, we create a Jedis
instance which connects to Redis server running on localhost. Then, using the get
method, we retrieve the value associated with 'myKey'. If the key exists, its corresponding value is printed on the console.
- Handling non-existent keys:
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost");
String key = "nonExistentKey";
String value = jedis.get(key);
if (value != null) {
System.out.println("Value: " + value);
} else {
System.out.println("Key not found");
}
}
}
In this example, we attempt to get the value of 'nonExistentKey'. When a non-existent key is queried, jedis.get(key)
returns null. We use a simple if-else statement to handle this scenario.
Best Practices
Always check if the returned value is not null before performing operations on it. It's a good practice to avoid NullPointerExceptions.
Common Mistakes
Not managing Redis connections properly can lead to issues. Ensure that the Redis connection is safely closed after the operation to prevent resource leaks.
FAQs
- What happens if the key does not exist in Redis? If you try to retrieve a non-existent key from Redis, it will return null.
- Can I connect to a remote Redis server? Yes, while creating your Jedis instance, simply pass the IP address or hostname of your remote Redis server instead of 'localhost'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
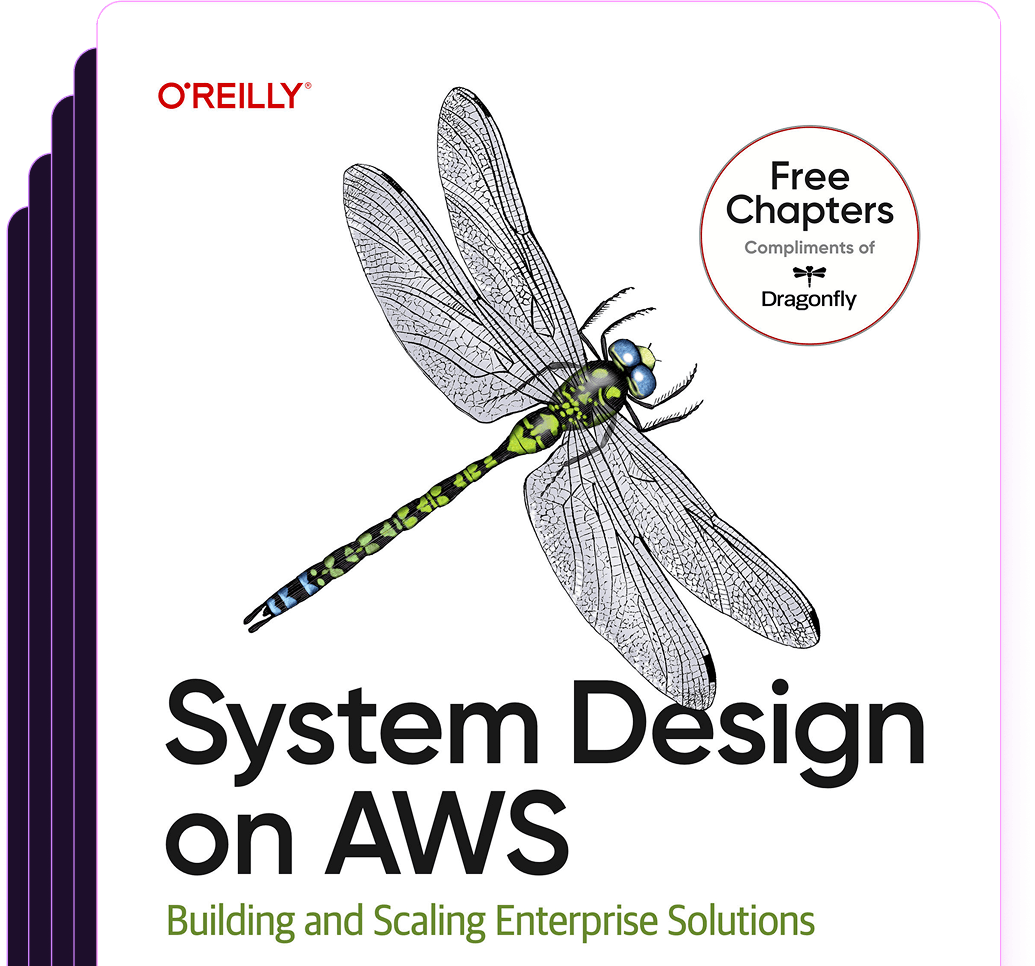
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost