Checking if a Key Exists in Redis with Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js, when interacting with a Redis database, you might need to check whether a particular key exists in the database or not. This is helpful when you want to avoid overwriting existing data or handle missing keys differently.
Code Examples
Below is an example of how you can use exists
function from the redis
package in Node.js:
const redis = require('redis');
const client = redis.createClient();
client.on('connect', () => {
console.log('Connected to Redis...');
});
let key = 'myKey';
client.exists(key, (err, reply) => {
if(err) {
console.error(err);
} else {
if(reply === 1) {
console.log('Key exists.');
} else {
console.log('Key does not exist.');
}
}
});
In this code snippet, we first create a connection to the Redis server using redis.createClient()
. We then use the exists()
method provided by the redis
package to check if a specific key (in this case 'myKey') exists in the database.
Best Practices
When checking if a key exists, make sure to handle errors properly to prevent any application crashes. It's also advisable to close the connection to the Redis server once you're done performing your operations to free up system resources.
Common Mistakes
A common mistake is to assume that the key exists without checking. If the key does not exist and you try to use it, this can cause errors in your application.
FAQs
- Is there a limit to the number of keys that can be checked for existence at a time? No, Redis does not impose any limit on this. However, checking a large number of keys at once can potentially slow down your application due to the latency of the operation.
- What does the
exists
function return? Theexists
function returns 1 if the key exists and 0 if the key doesn't exist.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
- Node Redis: Get Number of Subscribers
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
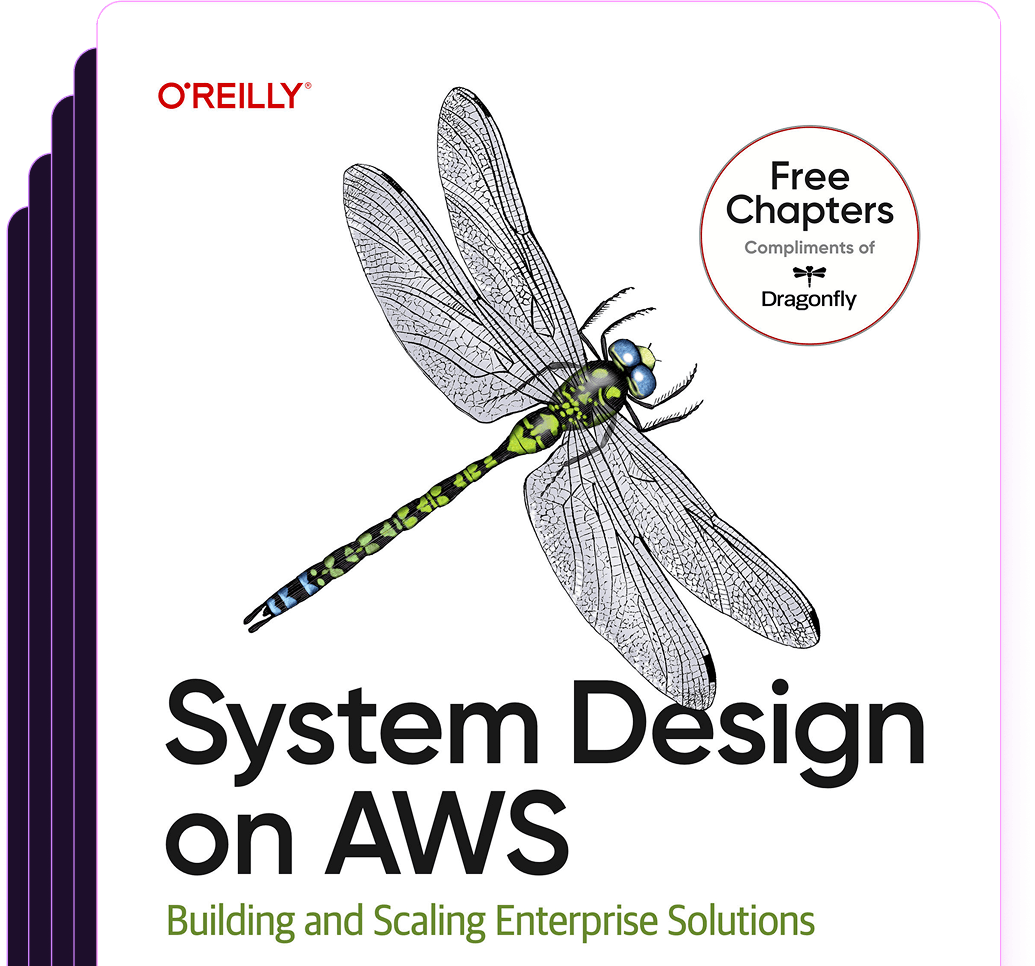
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost