Node Redis: Get All Keys Matching Pattern (Detailed Guide w/ Code Examples)
Use Case(s)
One common use case of getting all keys matching a particular pattern in Redis is when you want to fetch all keys that start, end or contain a certain substring. This can be very useful in scenarios where keys are named following a certain convention and you need to retrieve all keys belonging to a specific group.
Code Examples
Using the KEYS
command is one way to get all keys matching a pattern:
const redis = require('redis');
const client = redis.createClient();
client.keys('*pattern*', function (err, keys) {
if (err) return console.log(err);
for(var i = 0, len = keys.length; i < len; i++) {
console.log(keys[i]);
}
});
In this code snippet, you connect to the Redis server using redis.createClient()
. Then, you use the keys('*pattern*')
function to get all keys that match the specified pattern.
Remember that KEYS
command can be resource-intensive on large databases. It's recommended to use SCAN
for such cases, which can be done as follows:
const redis = require('redis');
const client = redis.createClient();
let cursor = '0';
function scan() {
client.scan(cursor, 'MATCH', '*pattern*', 'COUNT', '100', function(err, reply) {
if(err) throw err;
cursor = reply[0];
reply[1].forEach(function(key, i) {
console.log(key);
});
if(cursor === '0') {
return console.log('Scan complete');
} else {
return scan();
}
});
}
scan();
Here we're using the SCAN
command with a cursor to iteratively fetch keys matching the pattern.
Best Practices
Avoid using the KEYS
command in a production environment as it can potentially block the server while executing. Instead, prefer the SCAN
command, which provides an iterator that allows you to retrieve keys in a more controlled manner.
Common Mistakes
One common mistake is not handling the case when the KEYS
or SCAN
commands return an error. Always ensure to handle potential errors in your callbacks.
FAQs
- Q: Can I use regular expressions with the
KEYS
orSCAN
commands? A: No, Redis does not support full regex patterns inKEYS
orSCAN
commands. It supports only glob-style patterns like*
,?
and[chars]
.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
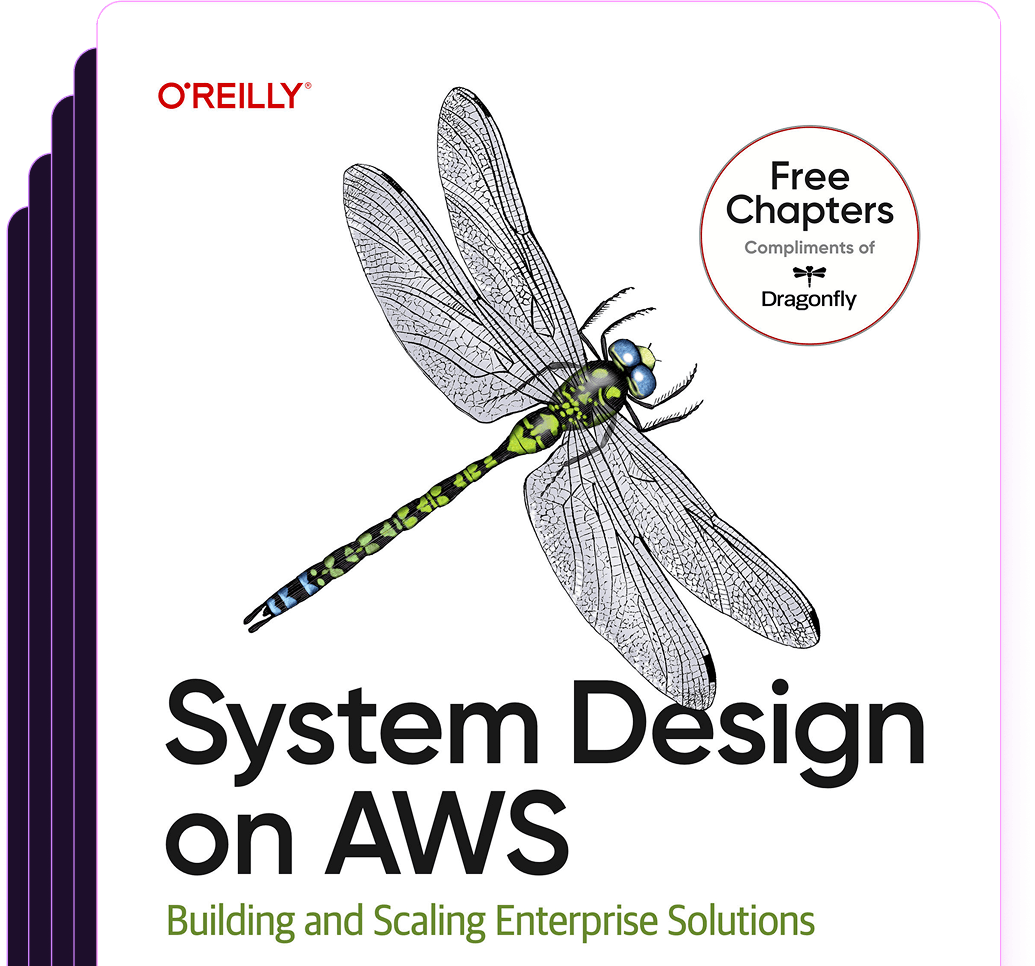
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost