Getting the Last Update Time in Redis with Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
Redis, being an in-memory data structure store, doesn't provide a built-in feature to directly fetch the last update time of a particular key. You may need this information for maintaining caches, debugging, or tracking usage patterns.
Code Examples
The common way to achieve this is by using separate keys to manually store timestamp whenever we make changes.
- Storing timestamps in separate keys
Here is how you can set a separate key for each data key to store the timestamp of the last update.
const redis = require('redis');
const client = redis.createClient();
// Assuming key is your data key
let key = 'user:123';
timeKey = key + ':lastUpdateTime';
client.set(key, 'value', 'EX', 3600); // set key with a TTL of 1 hour
client.set(timeKey, Date.now(), 'EX', 3600); // set timeKey with same TTL
To get the last update time:
client.get(timeKey, function(err, reply) {
console.log(new Date(parseInt(reply)));
});
Best Practices
If you find yourself frequently needing to keep track of the last update time for many keys, you might want to consider structuring your data differently. For instance, if it's possible within your application, you might store your values as part of a larger JSON object that also includes the last updated timestamp.
Common Mistakes
Be careful about expiry times (TTLs). If your 'data key' and 'timestamp key' have different TTLs, they might not expire at the same time, leading to inconsistencies.
FAQs
- Does Redis provide a built-in way to get the last update time of a key?
No, Redis does not provide a built-in feature for this. We need to handle it at application level.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
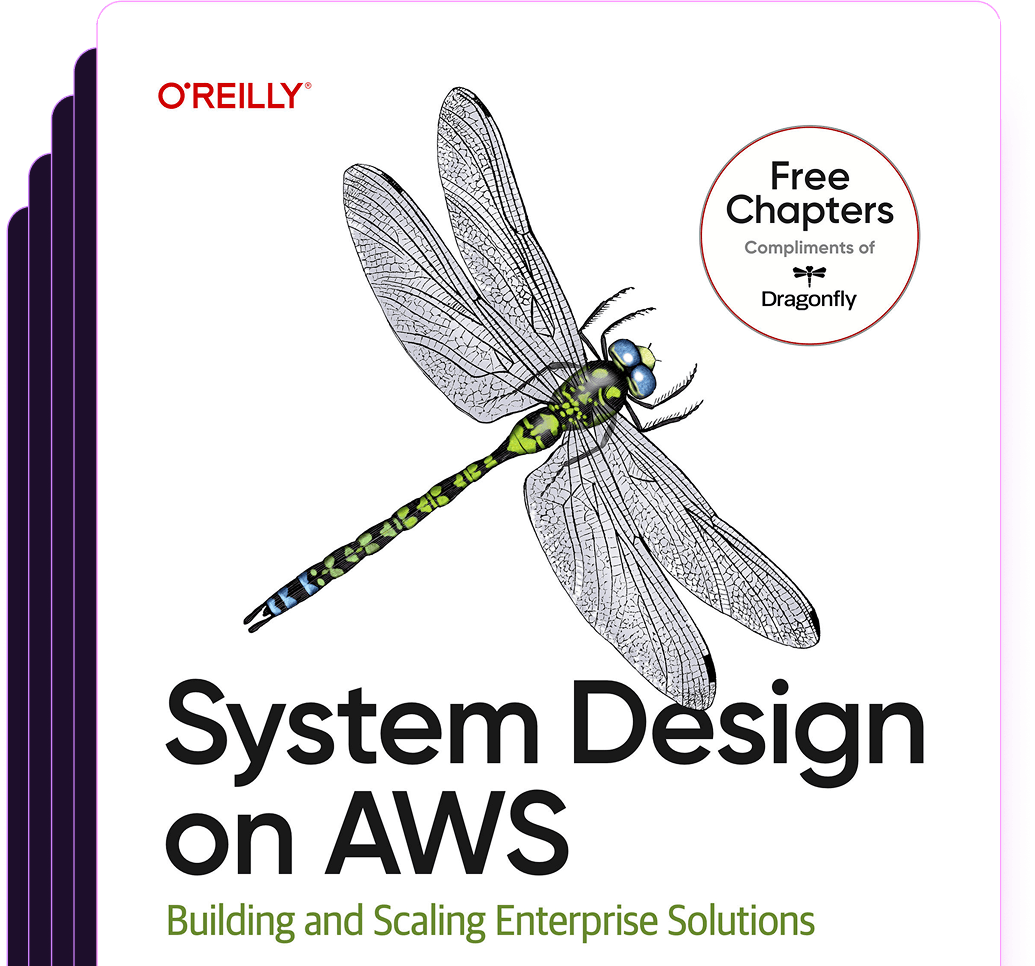
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost